How to convert HTML to PDF using iTextSharp (Updated 2025)
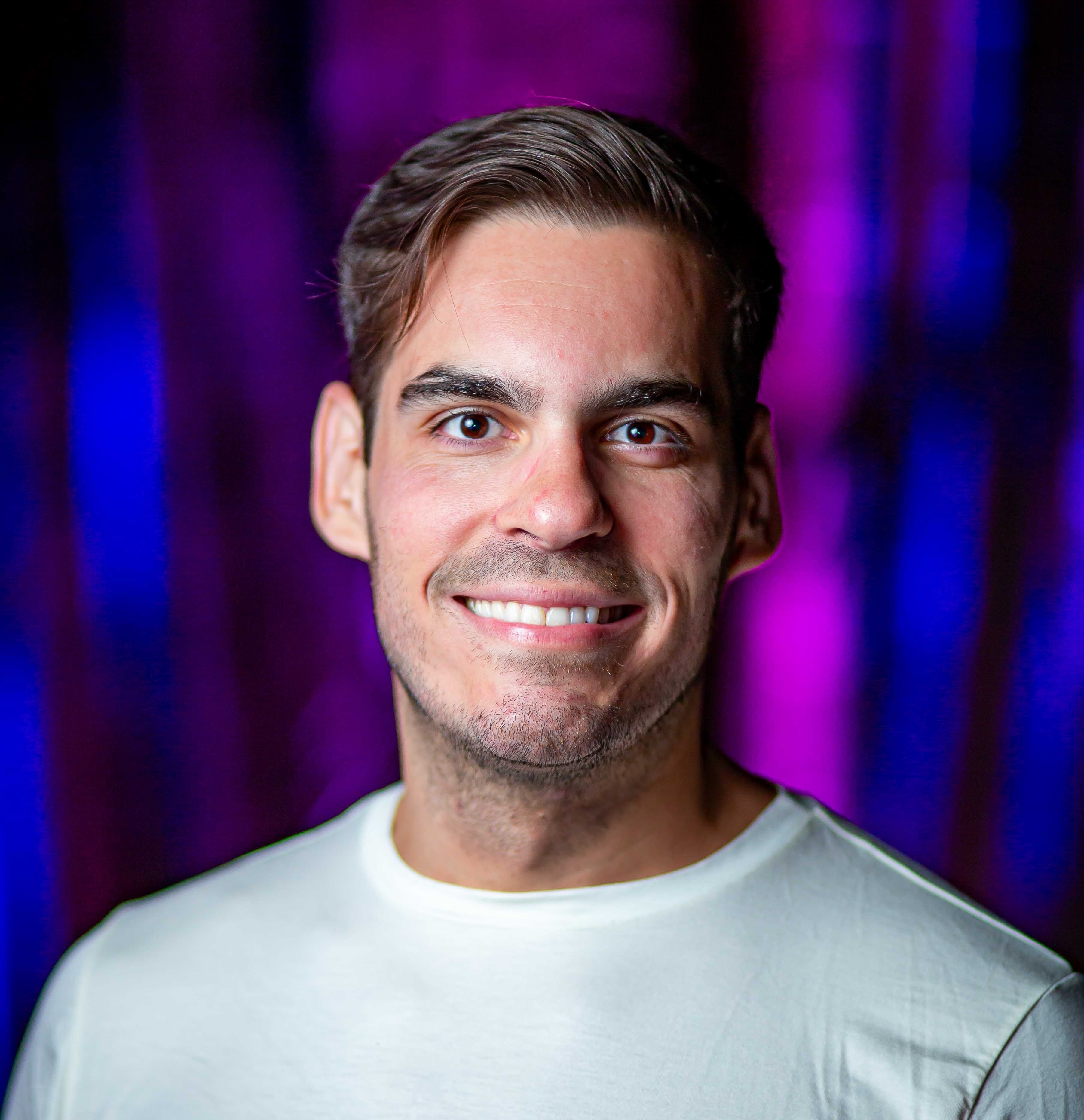
Introduction: Why iTextSharp?
iTextSharp is a robust PDF library for .NET, making it an excellent tool for generating PDF reports in SaaS applications, whether for invoices, summaries, or detailed documents. In this article, we’ll explore how to convert HTML to PDF using iTextSharp. By leveraging C# and this library, you’ll be able to integrate PDF generation seamlessly into your projects.
It’s available under AGPL or commercial licenses, so be mindful of licensing terms when building proprietary or enterprise solutions.
You can check out the full documentation here.
Comparison Between iTextSharp and Other C# PDF Libraries
When selecting a PDF library, it’s crucial to consider factors like functionality, ease of use, and licensing. Here’s how iTextSharp compares to other popular options:
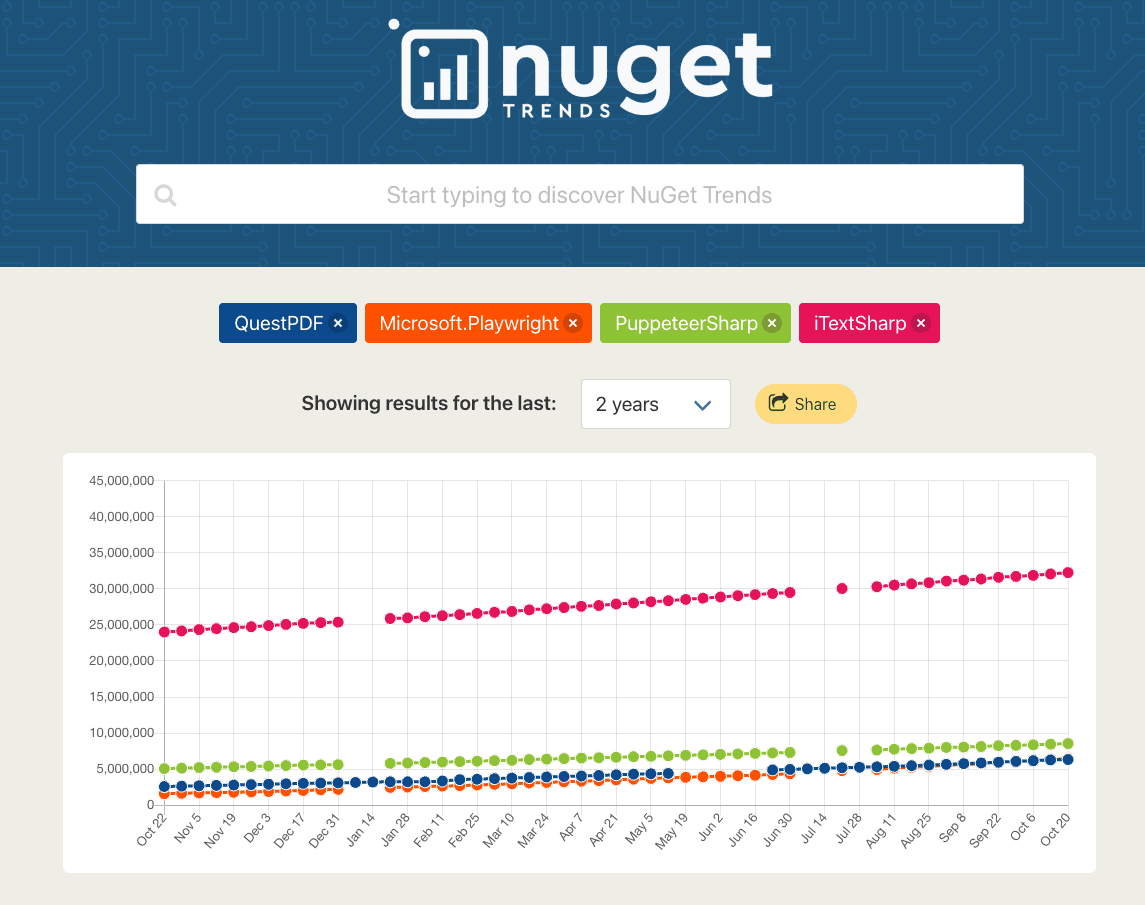
• iTextSharp: The most popular pdf library for C#. A feature-rich library supporting advanced PDF creation and manipulation. Ideal for complex tasks but requires attention to licensing for commercial use.
• PdfSharp: An open-source library suitable for basic PDF tasks. While it excels in creating PDFs from scratch, it lacks native support for HTML to PDF conversion without additional extensions.
• QuestPDF: A modern, open-source library offering a fluent API for building PDFs using C#. It excels at programmatic PDF generation but doesn’t natively support HTML to PDF conversion.
• PuppeteerSharp and Playwright: Headless browser tools that render HTML to PDF by simulating a browser environment. They handle modern HTML/CSS well but can introduce unnecessary overhead for simple tasks.
iTextSharp strikes a balance between functionality and performance, making it a solid choice for converting HTML to PDF in a .NET environment.
We also have a full comparison between C# PDF generation libraries, that you can check out here.
Setting Up iTextSharp in Your C# Project
Before diving into code, you’ll need to set up iTextSharp in your project.
Installing iTextSharp via NuGet in a .NET Environment
Use the NuGet Package Manager to install iTextSharp:
Or via the Package Manager Console:
Ensure that your project targets a compatible .NET framework version.
Preparing Your Project for HTML to PDF Conversion
Add the necessary namespaces in your C# files:
Verify that all dependencies are correctly referenced to avoid runtime issues.
Implementing HTML to PDF Conversion with iTextSharp
Let’s walk through creating a complete invoice in HTML/CSS and converting it to PDF.
Start by designing your invoice template in HTML:
Save this HTML content as invoice_template.html.
Using Template Engines for Dynamic HTML Generation
To generate dynamic invoices populated with data, integrate a template engine like Razor or Scriban. This allows you to inject variables and loop over data structures.
Example with Scriban:
This approach enables you to create personalized invoices by passing different data models.
Writing C# Code to Convert HTML to PDF
Now, let’s write the C# code to convert the HTML content into a PDF document:
Troubleshooting & FAQ
Why are my CSS styles missing?
Ensure CSS is either inline or in the <style> block within the HTML. iTextSharp’s XMLWorker can struggle with linked external styles unless you reference absolute URLs or embedded styles.
How do I handle images?
Use absolute paths or convert images to base64 for reliable rendering.
What about special characters or fonts?
Specify the correct encoding (UTF-8) and embed fonts if you need special glyphs.
How can I improve performance for large documents?
Consider streaming the PDF output and reusing iTextSharp objects if possible, or upgrading to iText7 which can offer better performance in some cases.
Scaling PDF Generation with a Third-Party API
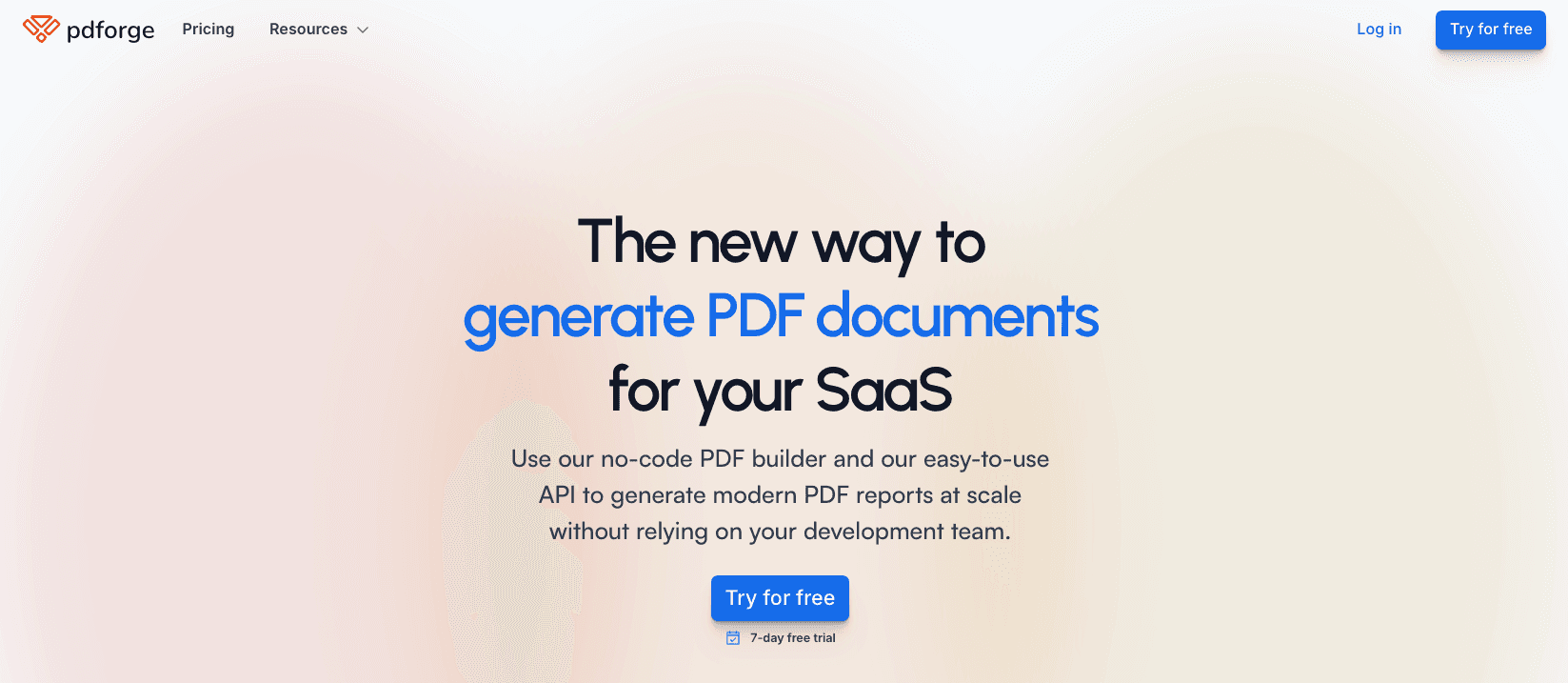
For larger SaaS platforms requiring automated PDF generation at scale, integrating a PDF Generation API like pdforge can offload the heavy lifting. This approach is ideal for SaaS platforms with high volumes of PDF requests.
With pdforge, you can create beautiful reports with flexible layouts and complex components with an easy-to-use opinionated no-code builder. Let the AI do the heavy lifting by generating your templates, creating custom components or even filling all the variables for you.
You can handle high-volume PDF generation from a single backend call.
Here’s an example of how to generate pdf with pdforge via an API call:
You can create your account, experience our no-code builder and create your first layout template without any upfront payment clicking here.
Conclusion: Choosing the Right PDF Workflow
iTextSharp is a formidable .NET library for HTML-to-PDF conversion, offering strong customization and PDF manipulation features.
When to Use iTextSharp: If you need fine-grained PDF control (forms, annotations), or you prefer an AGPL-based solution.
When to Consider Alternatives:
PuppeteerSharp for pixel-perfect rendering of modern CSS/JavaScript.
QuestPDF for a fluent, code-based layout approach.
PdfSharp for lightweight projects without heavy HTML needs.
If you don't want to waste time maintaining pdfs layouts and their infrastructure or if you don't want to keep track of best practices to generate PDFs at scale, third-party PDF APIs like pdforge will save you hours of work and deliver a high quality pdf layout.
Updated for 2025 to reflect the latest iTextSharp features and best practices.
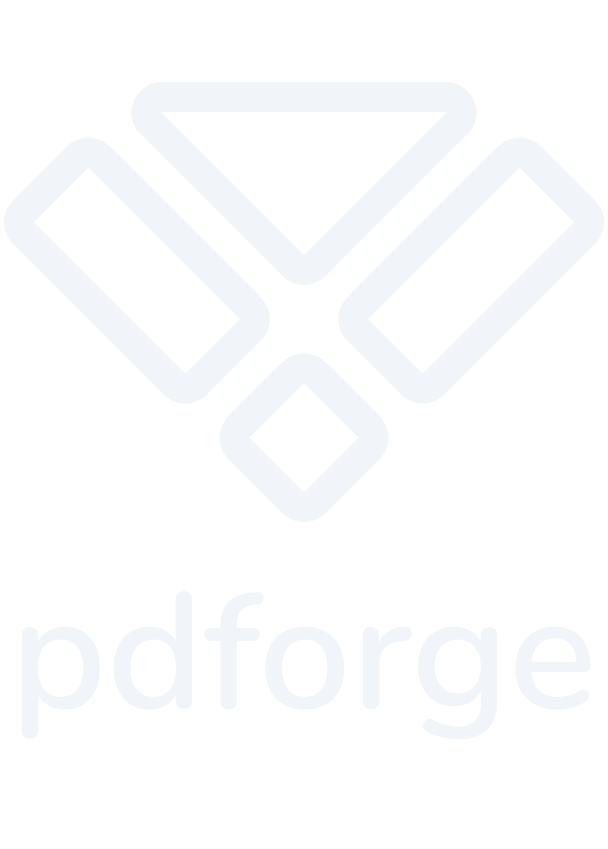
Try for free
7-day free trial