Overview of WickedPDF: A Trusted PDF Library for Rails
WickedPDF is a powerful PDF library for Ruby on Rails that enables developers to generate PDF documents directly from HTML. By using wkhtmltopdf, WickedPDF converts HTML, CSS, and JavaScript into accurate, high-quality PDFs, capturing the intended style and layout seamlessly.
You can check out the full documentation here.
Comparison Between WickedPDF and Other Ruby PDF Libraries
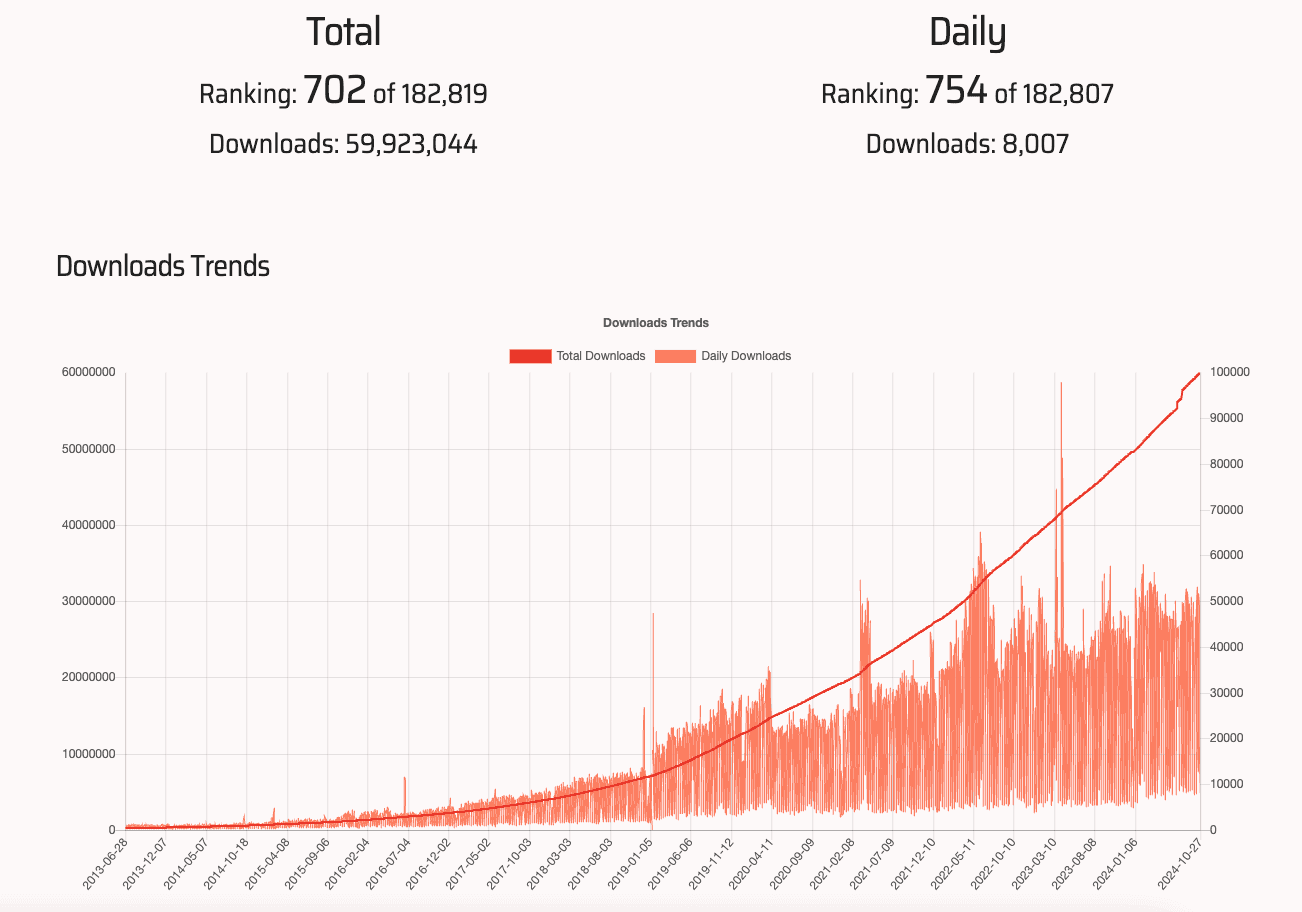
Ruby offers a range of PDF generation libraries, each with unique features:
Prawn and HexaPDF - Offer complete control over document structure, making them ideal for generating PDFs from scratch. However, they lack support for direct HTML conversion, which limits their flexibility for web-based applications.
PDFKit - Enable HTML-to-PDF conversion but don’t offer seamless integration with Rails, requiring extra configuration to work effectively within Rails projects.
Puppeteer-ruby and Grover - Uses a headless Chromium instance for rendering, offering excellent compatibility with complex HTML and JavaScript, though it may be heavier and more resource-intensive for simple PDF needs.
In comparison, WickedPDF Provides smooth HTML-to-PDF rendering with deep Rails integration, simplifying setup and use. It’s an excellent choice for Rails projects that need straightforward HTML-to-PDF generation. If you want to dig deeper on a comparison between WickedPDF and other Ruby pdf gems, we also have a detailed article with a full comparison between the best PDF gems for Ruby on rails in 2025.
Setting Up WickedPDF and Required Dependencies
Installing WickedPDF and PDF Rendering Dependencies
To get started, add the wicked_pdf gem to your Rails project, along with wkhtmltopdf, which handles the actual PDF rendering. Add the following to your Gemfile and install with bundle install:
After installing wicked_pdf, download wkhtmltopdf and ensure it’s accessible in your system path, or configure WickedPDF to locate it directly.
Project Folder Structure
To organize your project for PDF generation, create dedicated folders for layout templates, style sheets, and any other assets used in your PDF files. You might structure it as follows:
Use pdf.html.erb
as a base layout for your PDFs and keep custom styling in pdf.css to maintain a clean structure.
Configuring WickedPDF for HTML to PDF Conversion
Setting up global PDF configurations simplifies WickedPDF usage across your project. Create an initializer (config/initializers/wicked_pdf.rb
) where you define options such as page orientation, page size, and default margin settings.
Generating PDFs from HTML in Ruby on Rails
Structuring HTML Templates for PDF Output
For structured PDF documents like invoices, create an HTML template that includes detailed sections, such as headers, itemized tables, and totals. In views/layouts/pdf.html.erb
, set up the layout template:
Using CSS for PDF Styling and Formatting with WickedPDF
Incorporate inline CSS within your HTML template or create an external stylesheet to control the layout, fonts, colors, and spacing. This approach maintains a consistent appearance for your PDF outputs. Using pdf.css helps keep the PDF styling separate, especially if it diverges from your main app styles.
Generating PDF Documents Programmatically with WickedPDF
To programmatically generate PDFs, set up a controller action that calls render pdf:. Here’s an example in the InvoicesController:
This code fetches the invoice record, then renders it in both HTML and PDF formats, allowing for seamless viewing.
Creating PDF Reports via Controller Actions in Rails
WickedPDF makes it simple to produce reports by adapting render pdf: in your controllers.
To create a custom report, pass data to the view, which will display it according to your defined layout. Rails helpers like link_to
and number_to_currency
can enhance report readability.
Handling Different File Delivery Options: Inline, Download, and Email
For flexibility in file delivery, WickedPDF allows you to specify disposition, which controls whether the PDF opens inline, prompts download, or attaches to an email. For example:
Leveraging WickedPDF Options for Custom PDF Settings
Customize your PDFs using WickedPDF’s advanced options. For instance, adding headers and footers is straightforward with header and footer parameters, enabling you to insert branding or metadata at the top and bottom of each page. The disable_smart_shrinking option can also be helpful in addressing layout issues, making content scaling consistent across pages.
How to Use a PDF API to Automate PDF Creation at Scale
For SaaS platforms, automating PDF generation at scale might require offloading the heavy lifting to a PDF API.
It's also an option to integrate with third-party APIs like pdforge you can handle high-volume PDF generation, complex formatting, and post-processing, all from a single backend call.
Here’s an example of how to integrate pdforge in Rails to convert HTML content into a PDF via an API call:
This code sends a POST request to the pdforge API, receives the generated PDF, and saves it locally.
Conclusion
Choosing the right PDF library depends on your project’s specific needs:
WickedPDF is ideal for HTML-to-PDF conversions within Rails, particularly for documents like invoices or reports that rely on HTML layouts.
Prawn or HexaPDF are better suited for highly customized PDFs built from scratch.
Meanwhile, for scalable, automated PDF generation, third-party APIs like pdforge are the optimal choice for high-demand applications.