Importance of Visual Data Representation in PDF Reports
Graphs in PDF reports are essential tools for presenting complex data in a comprehensible format. In the realm of SaaS applications, integrating dynamic graphs into PDF documents elevates the quality and professionalism of reports provided to clients and stakeholders.
Visualizing data through graphs in PDFs simplifies the interpretation of intricate information. It enhances the user experience by providing clear insights, making graphs in PDFs a vital feature for any SaaS solution aiming to deliver impactful reports.
Common Challenges in Embedding Graphs into PDFs
Developers often face challenges when embedding graphs into PDFs, such as rendering issues, maintaining quality during conversion, and ensuring compatibility across various PDF viewers. Overcoming these obstacles is crucial for delivering high-quality, data-rich PDF reports.
An Overview of the Top Chart Libraries in JavaScript
To effectively embed graphs into PDFs, choosing the right JavaScript chart library is paramount. Below is an overview of the top chart libraries, along with their pros and cons, and code snippets illustrating how to generate a simple line chart using vanilla JavaScript.
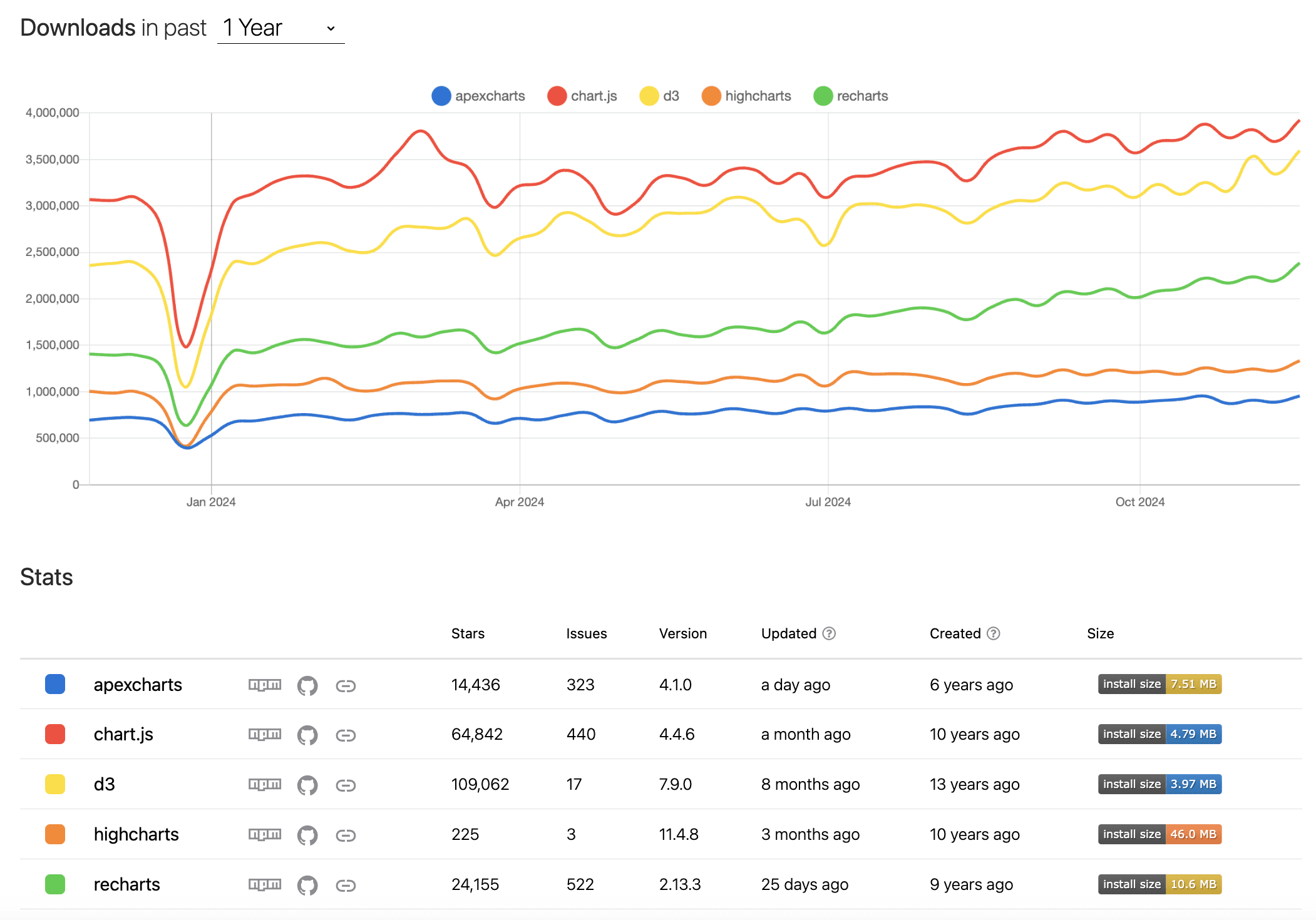
ApexCharts
Pros:
Easy to use with extensive documentation.
Wide range of chart types.
Works seamlessly with vanilla JavaScript and HTML templates.
Cons:
Some advanced features may require additional configuration.
Code Snippet:
<!-- Include ApexCharts library -->
<script src="https://cdn.jsdelivr.net/npm/apexcharts"></script>
<!-- HTML element for the chart -->
<div id="chart"></div>
<!-- Script to generate the chart -->
<script>
var options = {
chart: {
type: 'line',
height: 350,
},
series: [{
name: 'Revenue',
data: [4500, 4700, 5200, 5800, 6000, 6200, 7000]
}],
xaxis: {
categories: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul']
}
};
var chart = new ApexCharts(document.querySelector("#chart"), options);
chart.render();
</script>
Recharts
Pros:
Built on React components.
Ideal for React-based applications.
Cons:
Not suitable for projects not using React.
Less straightforward with vanilla JavaScript.
Code Snippet:
import React from 'react';
import { LineChart, Line, XAxis, YAxis, CartesianGrid } from 'recharts';
const data = [
{ name: 'Jan', revenue: 4500 },
{ name: 'Feb', revenue: 4700 },
{ name: 'Mar', revenue: 5200 },
{ name: 'Apr', revenue: 5800 },
{ name: 'May', revenue: 6000 },
{ name: 'Jun', revenue: 6200 },
{ name: 'Jul', revenue: 7000 },
];
const SimpleLineChart = () => (
<LineChart width={600} height={300} data={data}>
<CartesianGrid stroke="#ccc" />
<XAxis dataKey="name" />
<YAxis />
<Line type="monotone" dataKey="revenue" stroke="#8884d8" dot={false} />
</LineChart>
);
export default SimpleLineChart;
Chart.js
Pros:
Simple and flexible.
Supports multiple chart types.
Cons:
Limited customization compared to other libraries.
May require plugins for advanced features.
Code Snippet:
<!-- Include Chart.js library -->
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
<!-- HTML element for the chart -->
<canvas id="chart" width="400" height="200"></canvas>
<!-- Script to generate the chart -->
<script>
var ctx = document.getElementById('chart').getContext('2d');
var chart = new Chart(ctx, {
type: 'line',
data: {
labels: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul'],
datasets: [{
label: 'Revenue',
data: [4500, 4700, 5200, 5800, 6000, 6200, 7000],
borderColor: 'rgba(75, 192, 192, 1)',
fill: false
}]
},
options: {
tooltips: { enabled: false },
animation: { duration: 0 }
}
});
</script>
Highcharts
Pros:
Offers advanced chart types.
Highly customizable.
Cons:
Requires a license for commercial use.
Larger file size may affect performance.
Code Snippet:
<!-- Include Highcharts library -->
<script src="https://code.highcharts.com/highcharts.js"></script>
<!-- HTML element for the chart -->
<div id="chart"></div>
<!-- Script to generate the chart -->
<script>
Highcharts.chart('chart', {
chart: {
type: 'line',
animation: false
},
title: { text: null },
xAxis: {
categories: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul']
},
yAxis: { title: { text: null } },
series: [{
name: 'Revenue',
data: [4500, 4700, 5200, 5800, 6000, 6200, 7000],
enableMouseTracking: false
}],
tooltip: { enabled: false }
});
</script>
D3.js
Pros:
Extremely powerful for custom data visualizations.
Offers unmatched flexibility.
Cons:
Steep learning curve.
Complex for simple charts.
Code Snippet:
<!-- Include D3.js library -->
<script src="https://d3js.org/d3.v6.min.js"></script>
<!-- HTML element for the chart -->
<svg id="chart" width="600" height="400"></svg>
<!-- Script to generate the chart -->
<script>
// Set the dimensions and margins of the graph
var margin = {top: 20, right: 30, bottom: 50, left: 60},
width = 600 - margin.left - margin.right,
height = 400 - margin.top - margin.bottom;
// Append the SVG object to the div called 'chart'
var svg = d3.select("#chart")
.append("svg")
.attr("width", width + margin.left + margin.right)
.attr("height", height + margin.top + margin.bottom)
.append("g")
.attr("transform",
"translate(" + margin.left + "," + margin.top + ")");
// Define the data
var data = [
{month: 'Jan', revenue: 4500},
{month: 'Feb', revenue: 4700},
{month: 'Mar', revenue: 5200},
{month: 'Apr', revenue: 5800},
{month: 'May', revenue: 6000},
{month: 'Jun', revenue: 6200},
{month: 'Jul', revenue: 7000}
];
// Set the ranges
var x = d3.scalePoint()
.domain(data.map(function(d) { return d.month; }))
.range([0, width]);
var y = d3.scaleLinear()
.domain([4000, 8000])
.range([height, 0]);
// Add the X Axis
svg.append("g")
.attr("transform", "translate(0," + height + ")")
.call(d3.axisBottom(x));
// Add the Y Axis
svg.append("g")
.call(d3.axisLeft(y));
// Define the line
var valueline = d3.line()
.x(function(d) { return x(d.month); })
.y(function(d) { return y(d.revenue); });
// Add the valueline path
svg.append("path")
.data([data])
.attr("class", "line")
.attr("d", valueline)
.attr("fill", "none")
.attr("stroke", "#8884d8")
.attr("stroke-width", 2);
// Remove tooltips and animations
// D3.js does not add tooltips or animations by default,
// so no additional code is needed to disable them.
</script>
Our Recommendation
We recommend ApexCharts because it integrates easily with vanilla JavaScript and HTML templates. Its simplicity and powerful features make it ideal for embedding graphs in PDFs without the need for complex configurations.
You can check out the full documentation for ApexCharts here.
Creating Dynamic Graphs with JavaScript and HTML
Let’s create an invoice HTML/CSS file with a line chart section using ApexCharts.
Step 1: Set Up Your HTML Template
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Invoice with Graph</title>
<link rel="stylesheet" href="styles.css">
<script src="https://cdn.jsdelivr.net/npm/apexcharts"></script>
</head>
<body>
<div class="invoice">
<h1>Invoice #12345</h1>
<div id="chart"></div>
</div>
<script src="script.js"></script>
</body>
</html>
Step 2: Styling the Invoice (styles.css)
.invoice {
max-width: 800px;
margin: 0 auto;
font-family: Arial, sans-serif;
}
Step 3: Importing the Library and Creating the Chart (script.js)
var options = {
chart: {
type: 'line',
height: 350,
toolbar: {
show: false,
},
animations: {
enabled: false,
animateGradually: {
enabled: false,
},
dynamicAnimation: {
enabled: false,
}
}
},
series: [{
name: 'Revenue',
data: [4500, 4700, 5200, 5800, 6000, 6200, 7000]
}],
xaxis: {
categories: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul']
}
};
var chart = new ApexCharts(document.querySelector("#chart"), options);
chart.render();
HTML Template Engines for Dynamic Graphs
To inject dynamic data into your HTML template, you can use template engines like Handlebars. This allows you to populate the series object of ApexCharts options dynamically.
Using Handlebars:
<div id="chart"></div>
<script>
var options = {
chart: {
type: 'line',
height: 350,
toolbar: {
show: false,
},
animations: {
enabled: false,
animateGradually: {
enabled: false,
},
dynamicAnimation: {
enabled: false,
}
}
},
series: [{
name: 'Revenue',
data: {{json revenueData}}
}],
xaxis: {
categories: {{json categories}}
}
};
var chart = new ApexCharts(document.querySelector("#chart"), options);
chart.render();
<
Server-Side Rendering (e.g., with Node.js and Express):
app.get('/invoice', function(req, res) {
var data = {
revenueData: [4500, 4700, 5200, 5800, 6000, 6200, 7000],
categories: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul']
};
res.render('invoice', data);
});
Using PDF Libraries to Generate the PDF
After setting up your HTML and JavaScript, you can convert the web page into a PDF using libraries like Puppeteer.
Example with Puppeteer:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('http://localhost:3000/invoice', {waitUntil: 'networkidle0'});
await page.pdf({ path: 'invoice.pdf', format: 'A4' });
await browser.close();
})();
Using a Third-Party No-Code Template Builder
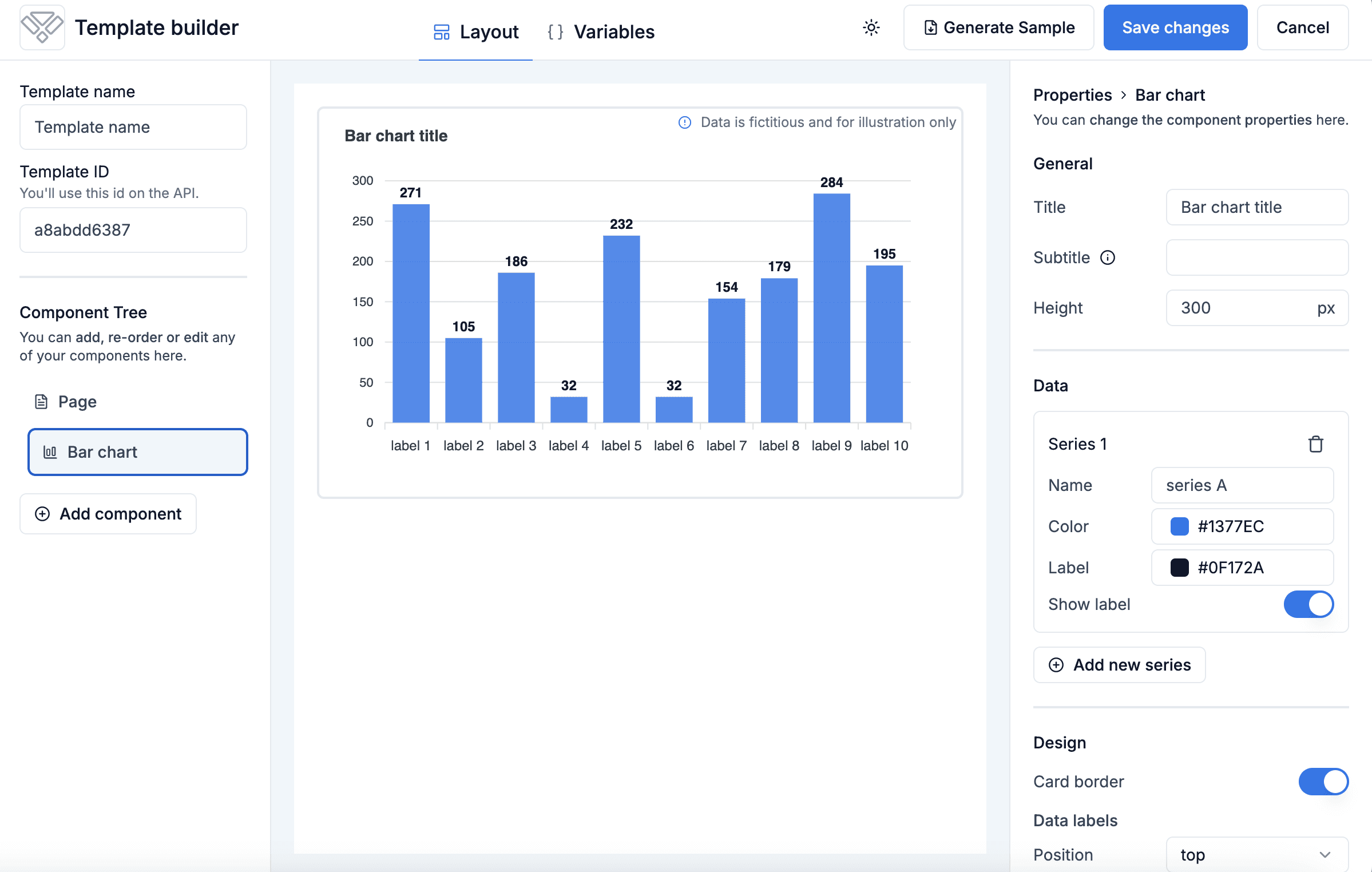
If you prefer a no-code solution, pdforge offers a template builder that allows you to generate charts inside PDFs without any coding.
• User-Friendly Interface: Design your templates with drag-and-drop functionality.
• Dynamic Data Integration: Easily connect your data sources.
• Professional Output: Create aesthetically pleasing PDFs with embedded charts.
Conclusion
Incorporating graphs in PDFs significantly enhances the effectiveness of your reports. While several JavaScript libraries are available, ApexCharts stands out for its ease of use with vanilla JavaScript and HTML templates. However, for better-looking layouts and to simplify the process, using third-party PDF generation APIs like pdforge is highly recommended.