Overview: Top PDF gems for Ruby on Rails in 2025
The Rails ecosystem provides an abundance of powerful gems for generating PDF documents.
We’ll explore the best gems for pdf generation, comparing how they convert html to pdf or use direct rendering methods. This article delves into three main categories: browser-based gems for pixel-perfect output, non-browser-based gems for deeper control over the PDF structure, and third-party APIs that offload rendering to external services.
Three Main Categories
Browser-based solutions (Puppeteer-Ruby, Grover, WickedPDF) leverage headless browsers or rendering engines for full CSS and JavaScript support.
Non-browser-based libraries (Prawn, PDFKit, HexaPDF) use direct drawing or partial HTML rendering for efficient document creation.
Third-party APIs (pdforge) handle the entire generation pipeline externally.
Below is an invoice template that every gem will use to generate the same PDF, allowing you to compare their output firsthand and see the differences in action.
Browser-Based Libraries: Convert HTML to PDF in Ruby on Rails
Browser-based gems transform HTML into PDFs by running a headless browser instance. They’re perfect for advanced JavaScript rendering, CSS transitions, or brand-heavy designs.
Generating PDF with Puppeteer-Ruby
Puppeteer-Ruby wraps the official Puppeteer library, offering precision rendering and comprehensive JavaScript support.
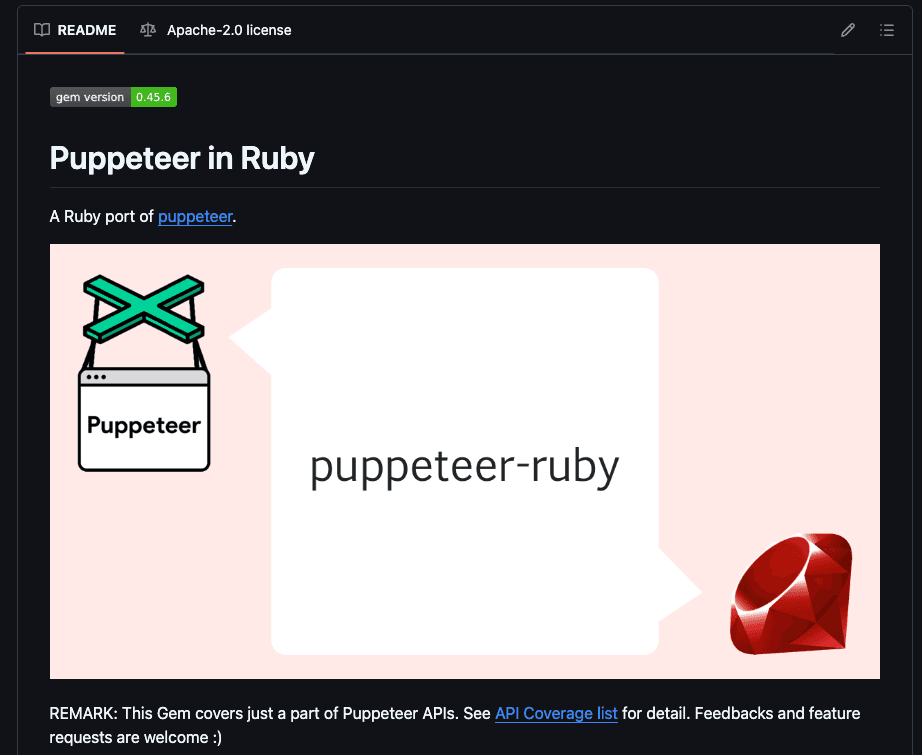
Installation & Basic Setup
Add puppeteer-ruby to your Gemfile and run bundle install.
In a controller, you can incorporate Puppeteer like this:
Enjoy full-page rendering, JavaScript execution for dynamic UI components, and robust CSS styling.
Ready to explore more? Unlock advanced Puppeteer usage in our Essential Puppeteer-Ruby Guide.
Generating PDF with Grover
Grover is a Rails-friendly approach to converting html to pdf using headless Chrome, often boasting simpler configuration than Puppeteer.
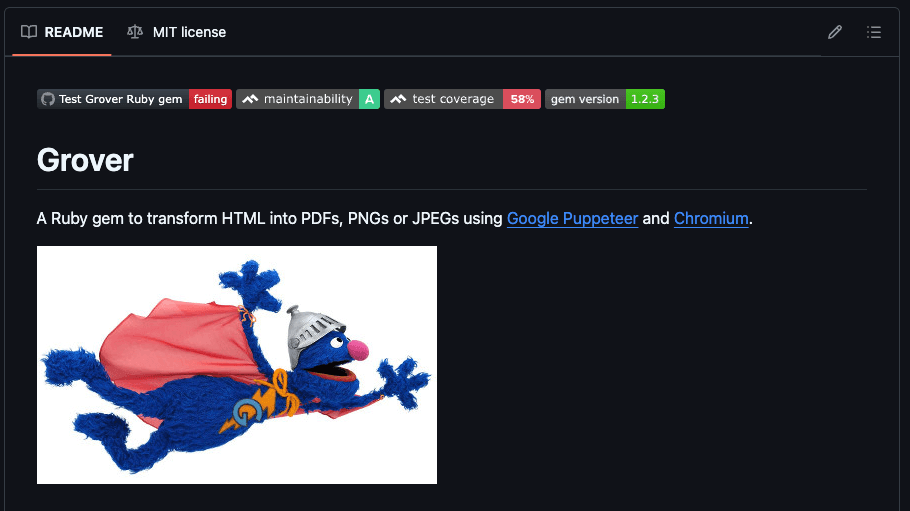
Installation & Configuration
Include grover in your Gemfile:
Then configure an initializer if needed:
Sample Code for Conversion
Grover performs well under load, integrates smoothly with Rails views, and has fewer dependencies.
Want to dive deeper? Check out our Grover Integration Tips to learn more.
Generating PDF with WickedPDF
WickedPDF uses wkhtmltopdf behind the scenes, making it a classic favorite in Rails for quick and easy PDF generation.
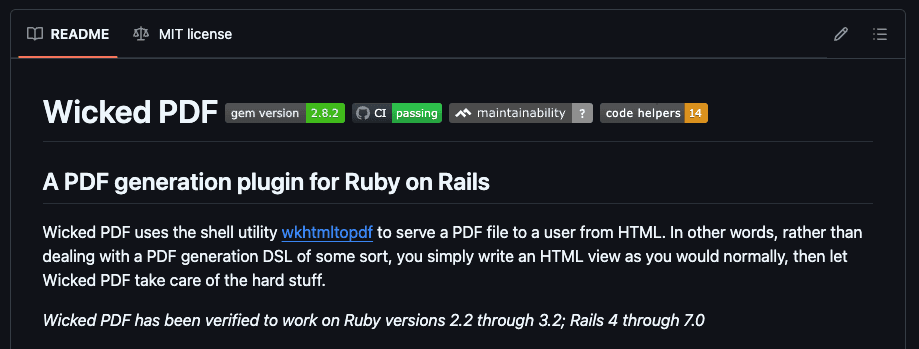
Setup & Installation
Add to Gemfile then run bundle install
Create an initializer:
Rendering a Rails View
WickedPDF excels at generating straightforward documents but can falter with heavy JavaScript usage.
Looking for more insights? Access our Complete WickedPDF Guide now.
Non-Browser-Based Libraries: Direct PDF Generation with Ruby’s Canvas API
Non-browser-based gems create PDFs via low-level drawing commands or partial rendering engines. They are typically faster, lighter on dependencies, and well suited for standardized or data-heavy reports.
Generating PDF with Prawn
Prawn delivers a powerful yet minimalist API for custom layouts. It foregoes HTML rendering in favor of direct PDF commands.
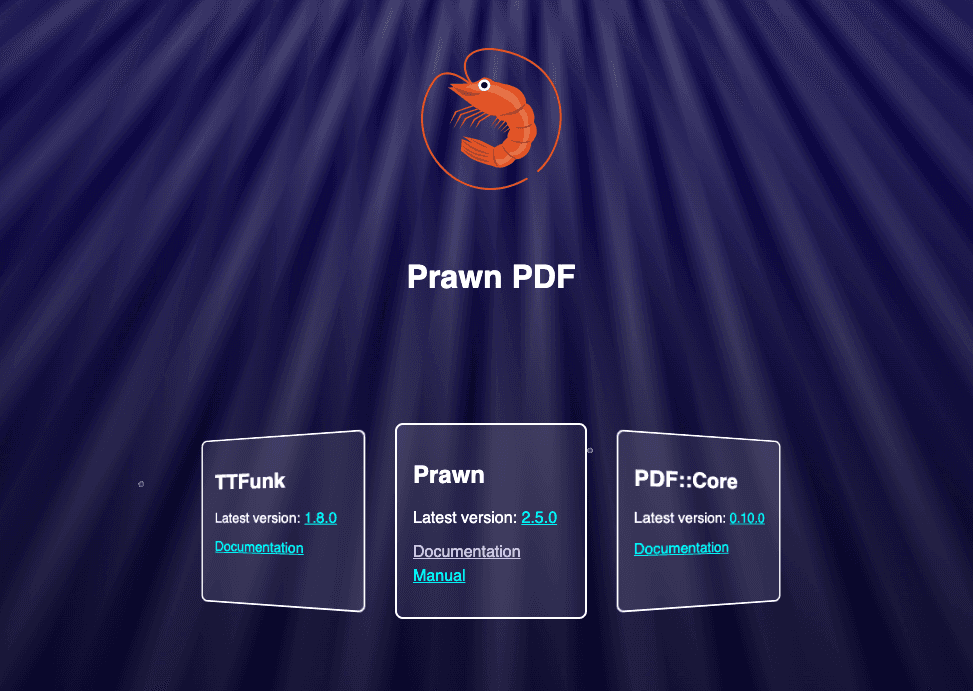
Installation & Setup
Add to Gemfile then run bundle install
Creating the invoice:
Prawn is both fast and highly configurable, making it a top choice among developers seeking direct Canvas API control.
Curious for more details? Explore the Ultimate Prawn PDF Guide.
Generating PDF with PDFKit
PDFKit simplifies HTML-to-PDF creation but doesn’t rely on a full browser environment. It uses wkhtmltopdf—similar to WickedPDF—yet keeps the interface minimal.
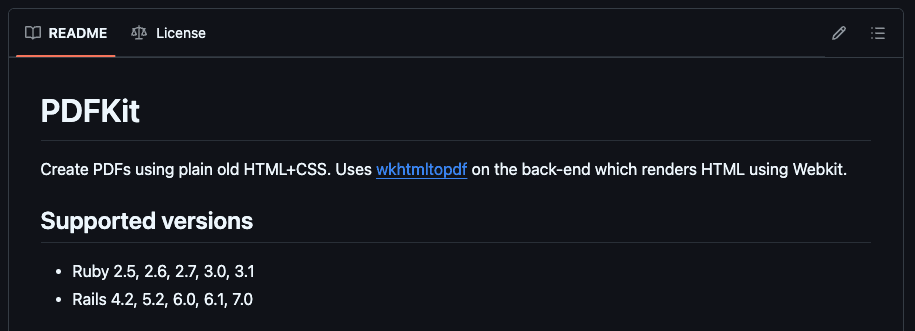
Setup in Rails
Add to Gemfile then run bundle install
Create an initializer:
HTML Invoices Conversion
PDFKit often outperforms heavier browser-based solutions but provides limited JS support.
For advanced features, check out our Deep Dive into PDFKit.
Generating PDF with HexaPDF
HexaPDF offers a modular design, letting you manipulate individual PDF objects, merge existing files, or optimize PDF sizes.
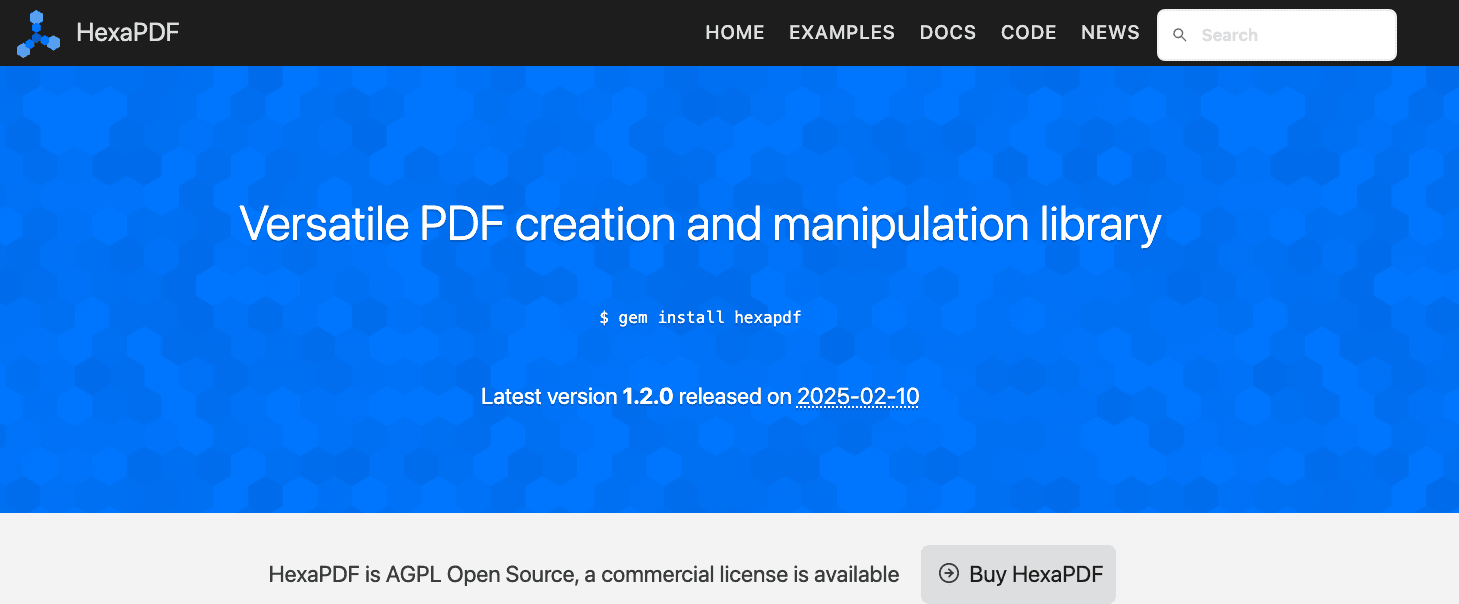
Installation & Example Usage
Add to Gemfile then run bundle install
Generating the invoice:
With efficient memory usage and built-in optimization, it’s ideal for large-scale or advanced PDF workflows.
Want to learn the finer points? Browse our HexaPDF Advanced Techniques section.
Third-Party PDF Generation APIs: Scalable and Flexible Solutions
Offloading pdf generation to an external service helps large businesses and SaaS platforms handle spiky traffic without tying up server resources.
Generating PDF with PDForge
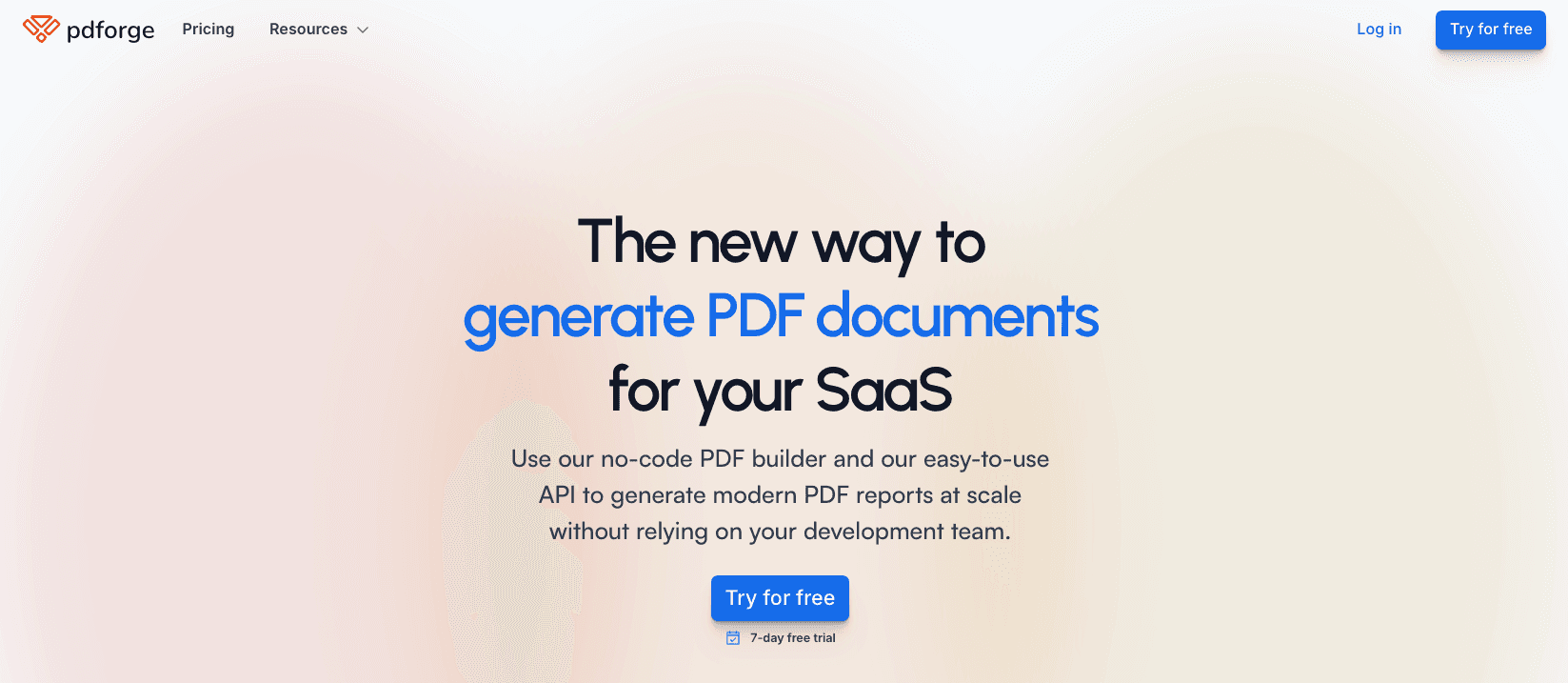
pdforge is a third-party pdf generation API. You can create beautiful reports with flexible layouts and complex components with an easy-to-use opinionated no-code builder. Let the AI do the heavy lifting by generating your templates, creating custom components or even filling all the variables for you.
You can handle high-volume PDF generation from a single backend call.
Here’s an example of how to generate pdf with pdforge via an API call:
You can create your account, experience our no-code builder and create your first layout template without any upfront payment clicking here.
If you want to explore other Third Party APIs, we listed all the top 7 PDF Generation APIs in 2025 for PDF Automation. Take a look and choose the best option for you!
Comparison of the Best PDF Libraries for Ruby on Rails
Below is a comparison among these best libraries. Determine which meets your needs based on ease of use, performance, feature set, and scalability.
Conclusion
Choosing from the best pdf gem for you depends on both functionality requirements and personal workflow preferences. For streamlined, structured invoices, Prawn remains an excellent non-browser-based choice thanks to its minimal dependencies. If you’re focusing on a browser-based approach with quick setup, WickedPDF is a classic choice for producing well-styled PDFs in a familiar Rails environment.
Meanwhile, choose third-party pdf generation APIs, like pdforge, if you don't want to waste time maintaining pdfs layouts and their infrastructure or if you don't want to keep track of best practices to generate PDFs at scale.