Generate PDF from HTML with iText: A Complete Guide
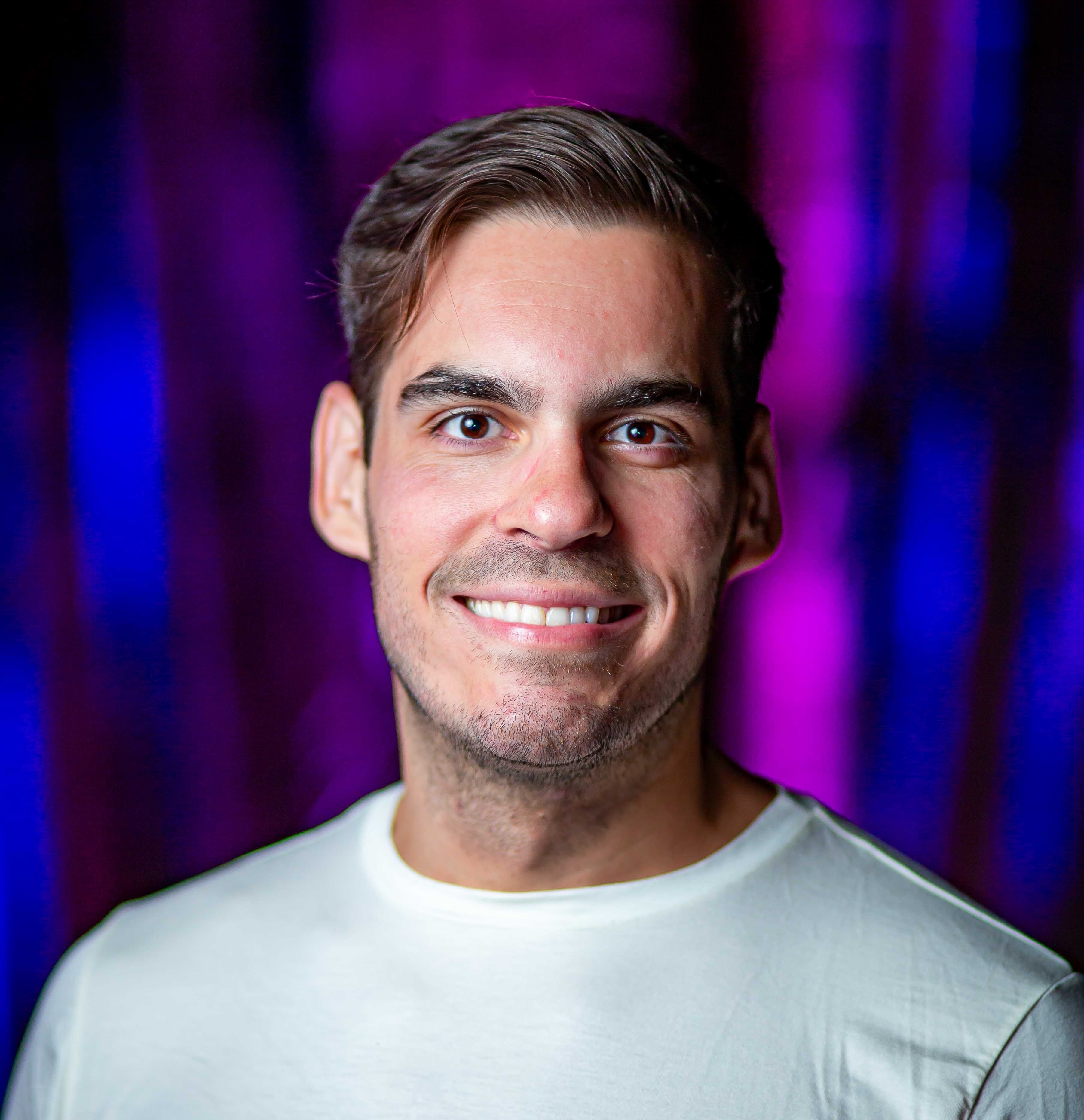
Understanding iText for PDF Generation
iText is a robust Java PDF library that empowers developers to create, manipulate, and edit PDF documents programmatically. It’s an essential tool for converting HTML content into PDFs, making it invaluable for generating dynamic PDF reports in SaaS applications.
You canm cfull documentation here.
Comparison Between iText and Other Java PDF Libraries
When considering PDF generation in Java, several libraries stand out:
iText: Offers comprehensive features for PDF creation and manipulation, including support for interactive forms, digital signatures, and complex layouts.
Flying Saucer: Specializes in rendering XHTML and CSS 2.1 content to PDF but lacks some advanced PDF features.
OpenPDF: An open-source fork of iText 4, suitable for basic PDF tasks but not as feature-rich as the latest iText versions.
Apache PDFBox: Provides capabilities for creating and manipulating PDFs but has limited support for HTML to PDF conversion.
Playwright: Primarily a browser automation tool that can generate PDFs from web pages but isn’t specialized for PDF customization.
Setting Up iText in Your Java Project
Configuring Your Environment
To ensure seamless PDF generation, set up your development environment properly:
• Java Development Kit (JDK): Install JDK 8 or higher.
• Build Tools: Use Maven or Gradle for dependency management.
• Integrated Development Environment (IDE): Opt for IntelliJ IDEA, Eclipse, or NetBeans for efficient coding.
Installing iText: Step-by-Step Guide for Java
Add iText to your project by including it as a dependency.
For Maven projects:
For Gradle projects:
Converting HTML to PDF Using iText
The Essentials of HTML to PDF Conversion
Converting HTML to PDF involves parsing HTML content and rendering it into a PDF format. iText simplifies this process with its html2pdf module, which handles HTML and CSS rendering seamlessly.
Creating a Complete Invoice HTML/CSS File as Example
To demonstrate dynamic data handling, we’ll create an invoice template using a template engine like Thymeleaf. The template engine allows you to inject dynamic data into your HTML before conversion.
invoice.html:
Using Template Engines for Dynamic Content
Template engines like Thymeleaf, Freemarker, or Velocity allow you to inject dynamic data into your HTML templates. Here’s how to use Thymeleaf to populate the invoice with dynamic data.
Writing Java Code for HTML to PDF Conversion
First, process the HTML template with the template engine to generate the final HTML.
Setting up Thymeleaf:
Generating the PDF:
Handling CSS, Images, and Fonts in Your PDFs
To correctly render CSS, images, and custom fonts, make sure to:
• Set Base URI: Define the base URI if your template references external resources.
• Embed Fonts: Use FontProvider to include custom fonts.
• Include Images: Ensure image paths are accessible and relative to the base URI.
Example with ConverterProperties:
Troubleshooting Common Issues in Conversion
Dynamic Data Not Displaying: Ensure the template engine correctly processes the data and that the variables in the template match those in your data model.
CSS Styles Missing: Confirm that styles are included inline or properly linked and that the base URI is set if needed.
Fonts Not Rendering: Embed the fonts using FontProvider to prevent font substitution.
Customizing PDFs with iText’s API
Enhance your PDFs by adding interactive elements or further customizing the layout.
Adding a Watermark:
How to Use a PDF API to Automate PDF Creation at Scale
For SaaS platforms, automating PDF generation at scale might require offloading the heavy lifting to a PDF API.
It's also an option to integrate with third-party APIs like pdforge you can handle high-volume PDF generation, complex formatting, and post-processing, all from a single backend call.
Here’s an example of how to integrate pdforge in Rails to convert HTML content into a PDF via an API call:
This code sends a POST request to the pdforge API, receives the generated PDF, and saves it locally.
Conclusion
When generating PDFs that require dynamic data and complex layouts, using iText in combination with a template engine like Thymeleaf is highly effective. This approach allows you to create flexible HTML templates that can be populated with data at runtime, making it ideal for SaaS applications needing customized reports.
If your requirements are simple and don’t necessitate advanced PDF features or dynamic content, libraries like OpenPDF, Flying Saucer or Playwright might be sufficient. They offer basic PDF generation capabilities without the overhead of more complex libraries.
For scaling PDF generation without burdening your own infrastructure, consider using third-party PDF APIs like pdforge. These services can handle large volumes and high concurrency, allowing you to focus on developing your application rather than managing PDF generation.
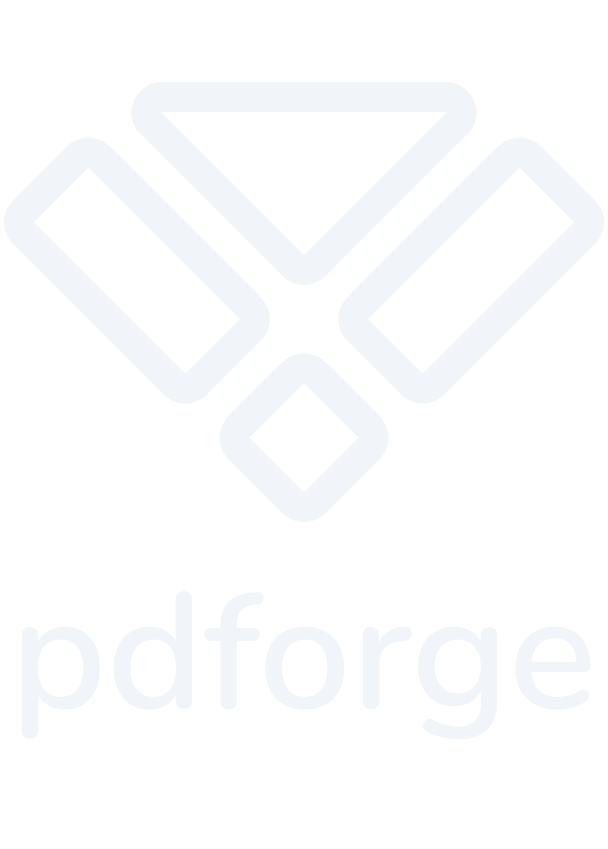
Try for free
7-day free trial