Quick Tutorial on Generating PDF from HTML with OpenPDF
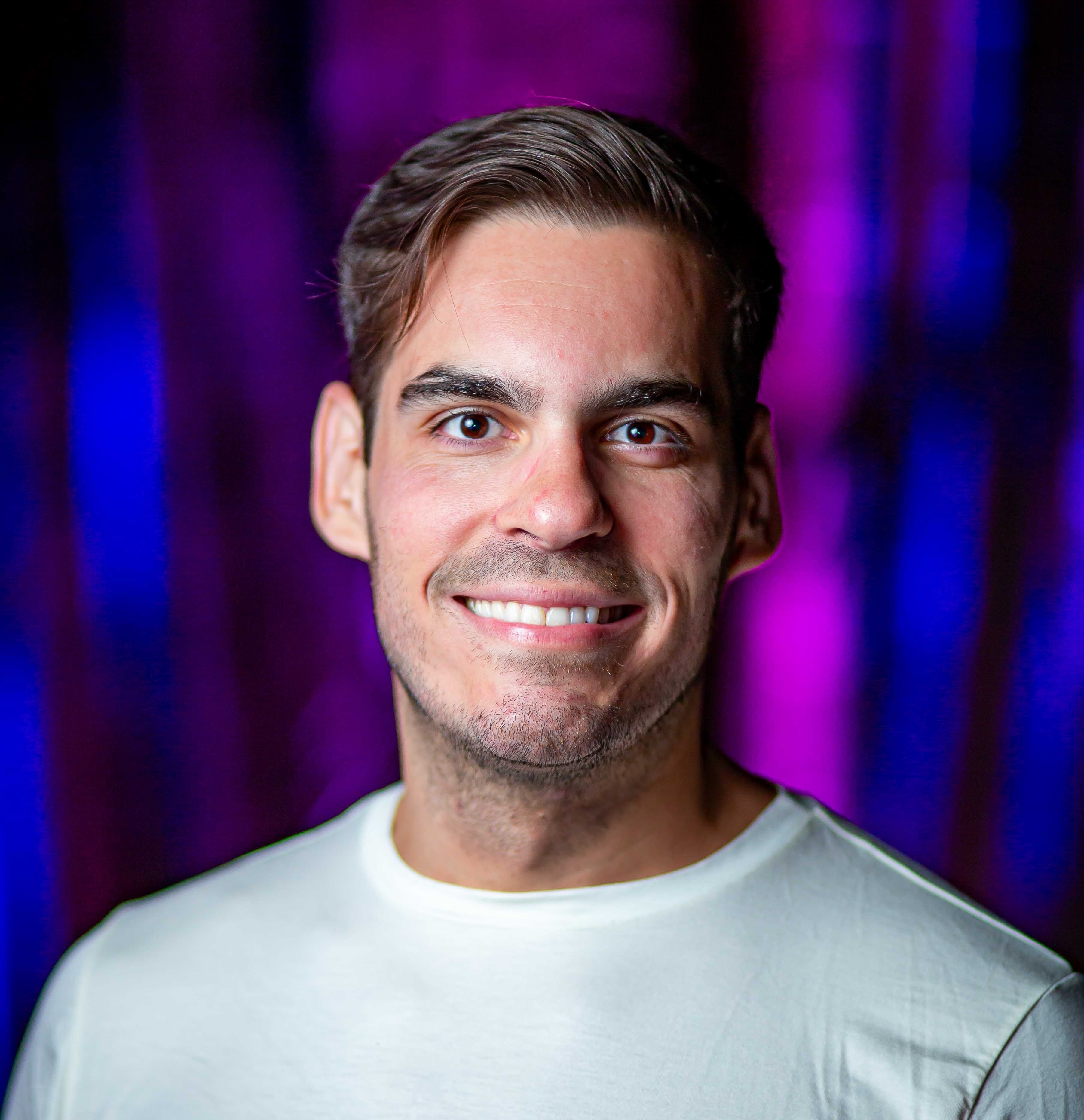
Introduction to OpenPDF for HTML to PDF Conversion
OpenPDF is an open-source Java library that enables developers to create and manipulate PDF documents programmatically. In SaaS applications, generating dynamic PDF reports from HTML content is a common requirement. While OpenPDF doesn’t support direct HTML to PDF conversion out of the box, it can be combined with other tools to achieve effective results.
You can check out the full documentation here.
Comparison Between OpenPDF and Other Java PDF Libraries
When choosing a PDF library or tool for your Java project, it’s crucial to consider features, licensing, community support, and how well it integrates with your technology stack.
OpenPDF: An LGPL/MPL-licensed library suitable for commercial use, focusing on PDF creation and manipulation within Java applications.
iText: A powerful library with extensive features, but newer versions are AGPL-licensed, which may not fit all projects due to licensing restrictions.
Apache PDFBox: Allows low-level PDF manipulation but lacks comprehensive HTML to PDF conversion support.
Flying Saucer: Specializes in rendering XHTML and CSS 2.1 to PDF, making it suitable for HTML to PDF tasks, though it’s less actively maintained.
Playwright: Primarily a browser automation tool that supports headless browser operations. It can render complex HTML and CSS to PDF by leveraging Chromium’s print to PDF capabilities, making it a good choice for generating PDFs from web content.
Setting Up OpenPDF in Your Java Project
To start using OpenPDF, add it to your project’s dependencies.
For Maven projects:
For Gradle projects:
Installing OpenPDF: A Quick Start Guide
Add the Dependency: Include OpenPDF in your pom.xml or build.gradle.
Refresh Dependencies: Update your project to fetch the new library.
Verify Imports: Ensure you can import OpenPDF classes in your code.
Configuring Your Environment for HTML to PDF
Since OpenPDF doesn’t natively support HTML to PDF conversion, you’ll need to integrate it with an HTML parser like JSoup to manually map HTML elements to PDF elements.
Converting HTML to PDF with OpenPDF
Let’s walk through creating a PDF invoice by parsing an HTML template.
Creating a Complete Invoice HTML/CSS File as an Example
Create an invoice.html file:
Writing Java Code for HTML to PDF Conversion
Add JSoup to your dependencies:
Implement the conversion:
Handling Dynamic Data in Your PDF
To deal with dynamic data, you can use placeholders in your HTML template and replace them at runtime.
Example:
In your Java code:
Handling CSS and Images in Your PDF Output
While OpenPDF doesn’t support CSS, you can manually apply styles.
• Fonts and Colors: Use OpenPDF’s Font class to set font styles and colors.
• Images: Extract image sources from HTML and add them using OpenPDF’s Image class.
Example of adding an image:
Example of styling text:
Best Practices for Using OpenPDF in Production
• Error Handling: Implement comprehensive exception management to catch and log errors.
• Resource Management: Use try-with-resources to ensure documents and streams are closed properly.
• Performance Optimization: Reuse fonts and images to optimize memory usage and performance.
How to Use a PDF API to Automate PDF Creation at Scale
For SaaS platforms, automating PDF generation at scale might require offloading the heavy lifting to a PDF API.
It's also an option to integrate with third-party APIs like pdforge you can handle high-volume PDF generation, complex formatting, and post-processing, all from a single backend call.
Here’s an example of how to integrate pdforge in Rails to convert HTML content into a PDF via an API call:
This code sends a POST request to the pdforge API, receives the generated PDF, and saves it locally.
Conclusion
OpenPDF is a solid choice when you need fine-grained control over PDF generation and are comfortable with manual mapping from HTML to PDF elements. It’s suitable for projects where you have simple HTML content and need extensive customization of the PDF output.
If your project involves complex HTML and CSS that need to be converted to PDF, libraries like Flying Saucer or iText, or tools like Playwright may be more fitting. Playwright can render complex web pages and generate PDFs using headless browsers, which is particularly useful when your content relies heavily on modern web technologies.
For scaling PDF generation or when you prefer not to handle the conversion logic yourself, third-party services like pdforge provide robust APIs. They handle complex HTML and CSS conversions efficiently, allowing you to focus on other aspects of your application.
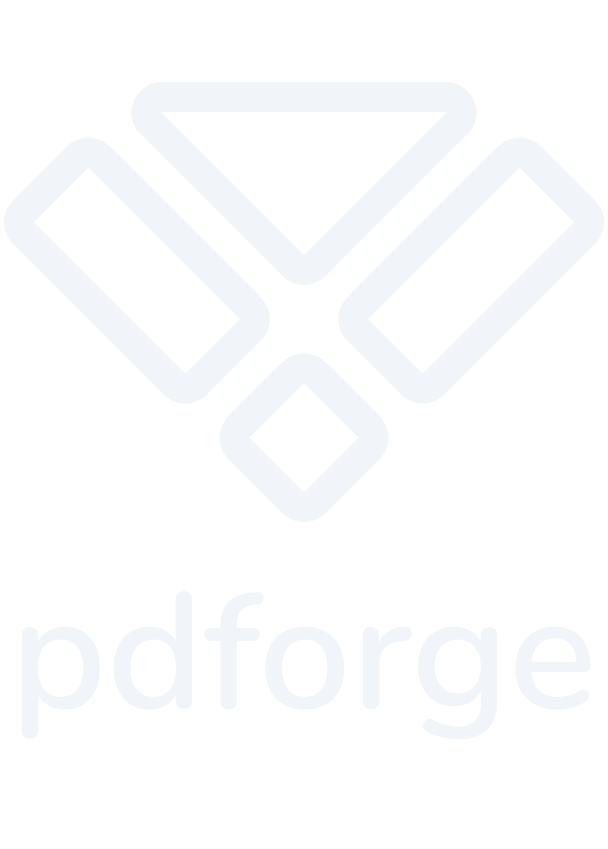
Try for free
7-day free trial