How to Generate PDF from HTML Using Dompdf
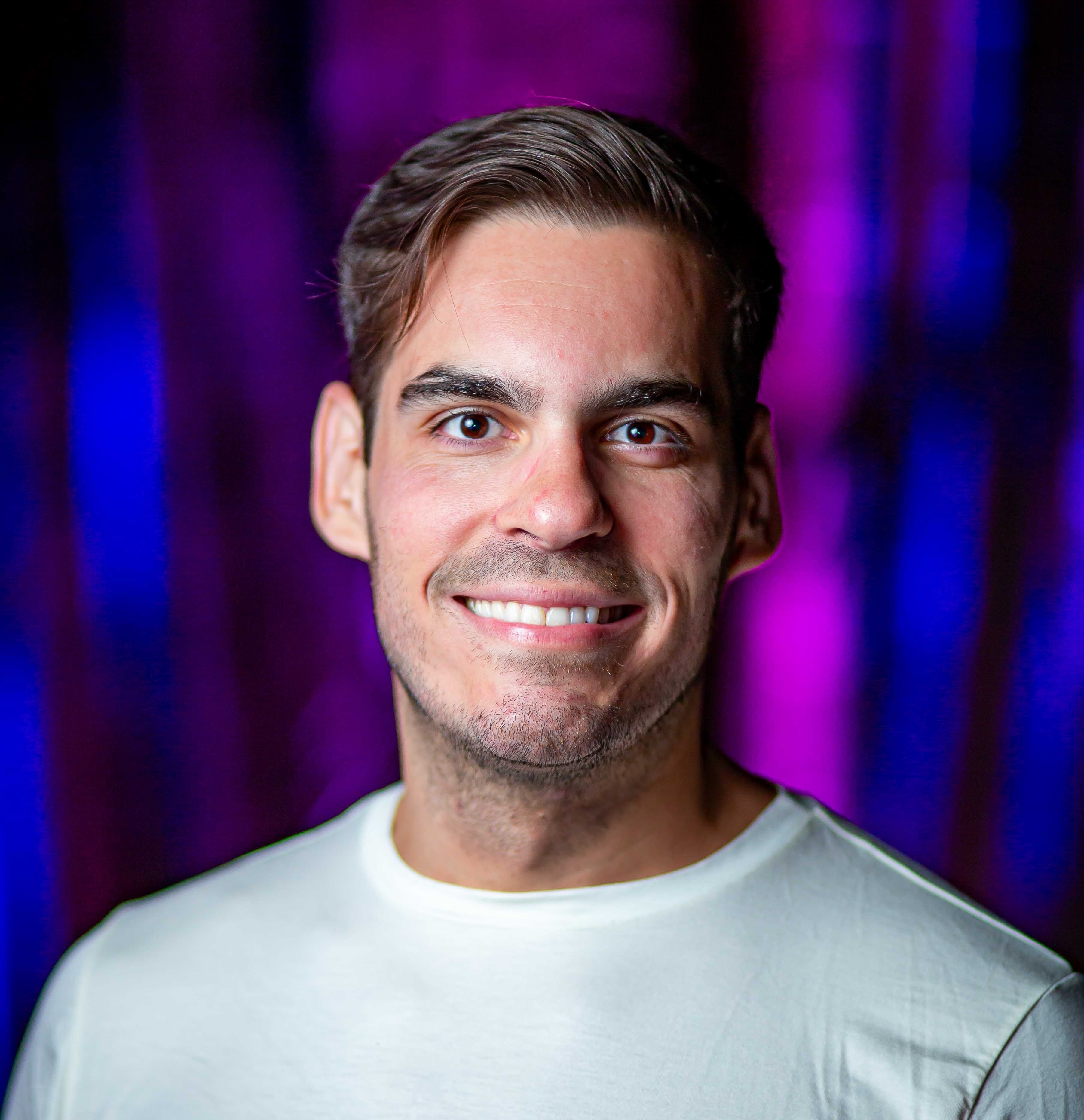
Introduction to Dompdf
Dompdf is a powerful, open-source library that converts HTML and CSS directly into PDF documents, making it easy for PHP developers to create professional-looking PDFs without manually constructing layouts. In this guide, you’ll learn:
- How to install Dompdf using Composer
- Step-by-step instructions for rendering static and dynamic HTML
- Advanced tips for styling, images, and pagination
- Best practices for SaaS environments and large-scale PDF generation
You can access the full documentation for dompdf here.
Comparison Between Dompdf and Other PHP PDF Libraries
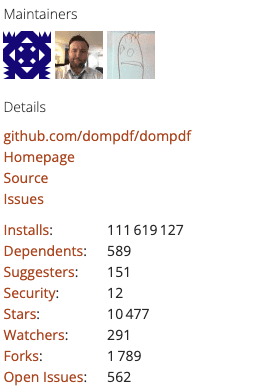
When choosing a PHP library for PDF generation, three names often arise: Dompdf, TCPDF, and MPDF. Here’s a quick feature comparison:
Dompdf excels in simplicity—perfect for projects that rely heavily on existing HTML. If you need more robust performance for extremely large PDFs, TCPDF may offer speed advantages but requires more low-level PDF structuring. mPDF falls somewhere in between, balancing HTML support with decent performance.
If you want to deep dive on a full comparison between the best PHP PDF libraries, check out this guide.
Setting Up Dompdf in Your PHP Project
Integrating Dompdf into your project is straightforward, especially when using Composer for dependency management.
Installing Dompdf via Composer
1. Install Composer: If you haven’t already, download and install Composer from getcomposer.org.
2. Require Dompdf: In your project directory, run the following command:
3. Include the Autoloader: Add the Composer autoloader to your PHP script:
Now, you’re ready to start generating PDFs from any HTML or CSS content!
Configuring Dompdf
Initialize Dompdf in your script:
Set the paper size and orientation (optional):
Exploring the Dompdf basic usage
Load your HTML content:
Render the PDF:
Output the generated PDF to the browser:
Generating Dynamic PDFs with Dompdf
Creating visually appealing PDFs requires well-structured HTML and CSS. Below is a simplified invoice template showing how you can embed dynamic data (like customer details, items, and totals) within HTML.
1. Invoice Template (invoice_template.php)
2. Sample PHP Variables (invoices.php or similar)
3. Rendering the Invoice with Dompdf
How It Works
1. Prepare Data: Define $customerName, $items, etc. in a PHP file.
2. Include Template: Use ob_start() and include 'invoice_template.php' to capture the rendered HTML (with dynamic data) into a variable.
3. Render: Load the HTML into Dompdf, set paper size/orientation, and call $dompdf->render().
4. Output: Finally, output the PDF to the browser with $dompdf->stream(), or save it to the server with $dompdf->output().
This approach keeps your invoice layout cleanly separated in invoice_template.php and allows for any dynamic data you need. Feel free to add additional styling, headers, footers, or images to suit your design requirements.
Handling Images, Pagination, Headers, and Footers
1. Embedding Images in the PDF
To include images (e.g., a company logo) in your generated PDFs, you can use an absolute URL or base64-encoded data. Make sure remote loading is enabled if you’re using external URLs.
If your images are local or behind authentication, consider converting them to base64 to ensure they render correctly.
2. Adding Pagination (Headers and Footers)
Dompdf can automatically paginate lengthy content, and you can inject headers/footers (including page numbers) by using fixed positions in your HTML/CSS. Below is an example of how to create a header with a logo and a footer that displays page numbers.
CSS for Fixed Header and Footer
HTML Structure with Header & Footer
PHP Integration
After preparing the above HTML/CSS, integrate it with Dompdf in your PHP script:
Note: Make sure the <header> and <footer> elements are positioned with negative margins so they occupy the space you configured in the @page { margin: ... } settings, but don’t overlap your main content.
Alternative: Scaling PDF Generation with Third-Party APIs
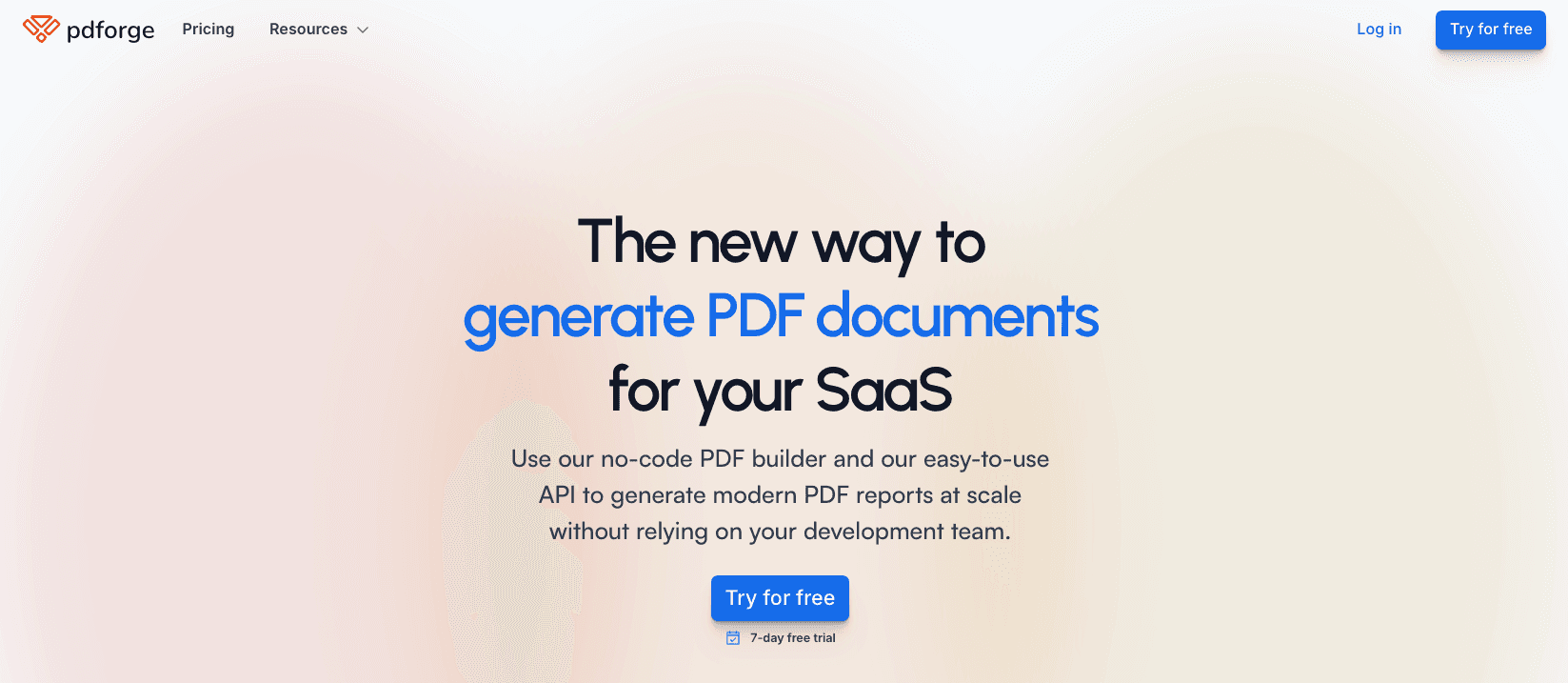
For larger SaaS platforms requiring automated PDF generation at scale, integrating a PDF Generation API like pdforge can offload the heavy lifting. This approach is ideal for SaaS platforms with high volumes of PDF requests.
With pdforge, you can create beautiful reports with flexible layouts and complex components with an easy-to-use opinionated no-code builder. Let the AI do the heavy lifting by generating your templates, creating custom components or even filling all the variables for you.
You can handle high-volume PDF generation from a single backend call.
Here’s an example of how to generate pdf with pdforge via an API call:
You can create your account, experience our no-code builder and create your first layout template without any upfront payment clicking here.
Conclusion
Dompdf offers a developer-friendly way to convert HTML and CSS into polished PDF files—ideal for invoices, reports, or any document-heavy SaaS feature. Its ease of use and strong community support make it a go-to for small to medium-sized projects. However, if you anticipate rapid growth or have strict performance requirements, explore TCPDF or mPDF for more granular control.
If you don't want to waste time maintaining pdfs layouts and their infrastructure or if you don't want to keep track of best practices to generate PDFs at scale, third-party PDF APIs like pdforge will save you hours of work and deliver a high quality pdf layout.