Generate PDFs from HTML Using TCPDF: A Developer’s Guide
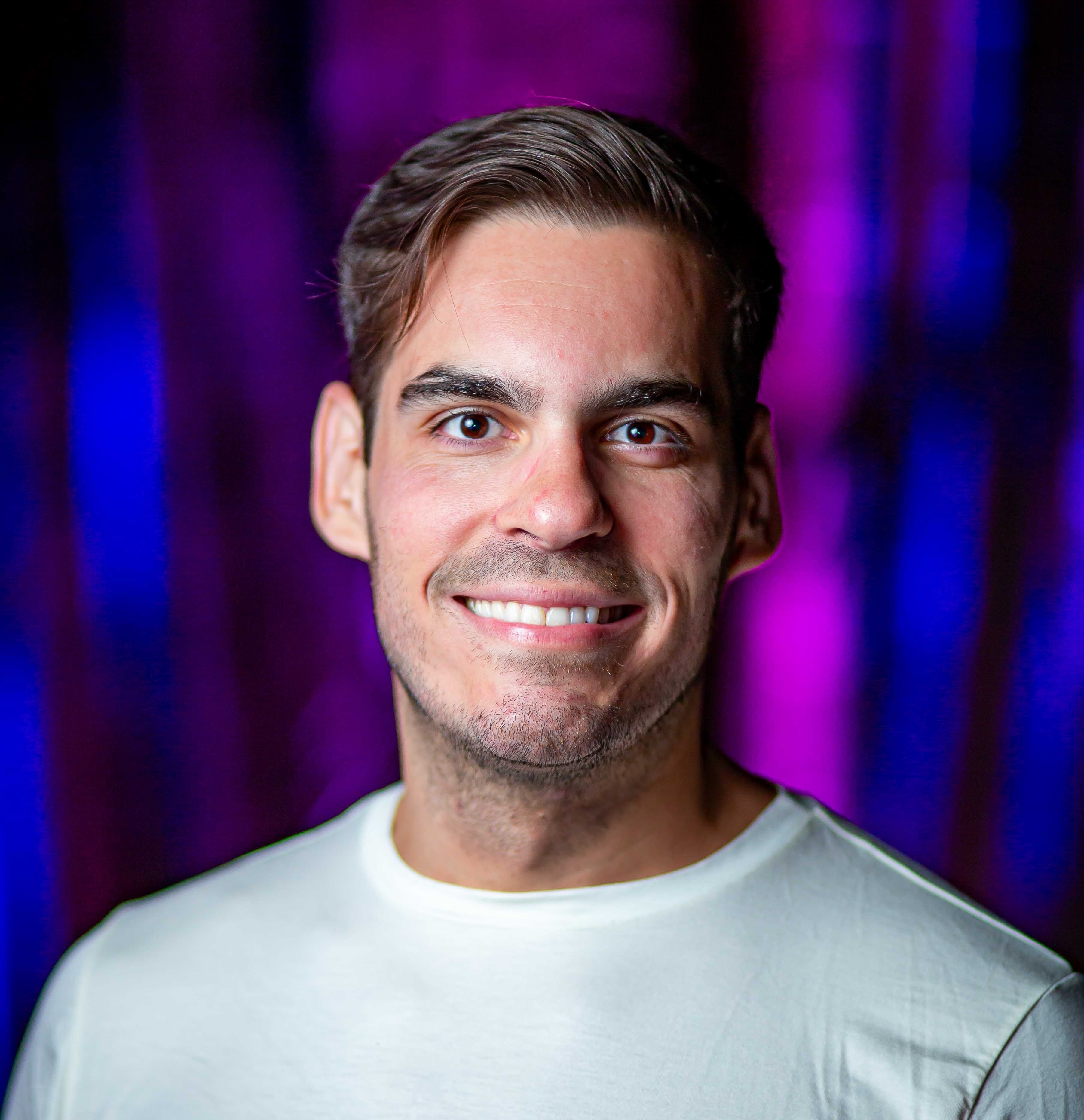
Introduction to TCPDF: Converting HTML to PDF in PHP
Generating PDFs from HTML content is a common requirement in SaaS applications, particularly for creating invoices, reports, or any downloadable documents. In this guide, we’ll explore how to leverage TCPDF, a powerful PHP library, to convert HTML into PDFs seamlessly.
You can check out the full documentation here.
Comparison Between TCPDF and Other PHP PDF Libraries
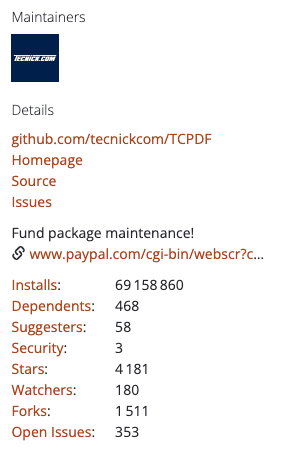
When it comes to PHP PDF libraries, several options are available, including Dompdf, mPDF and FPDF.
Here’s how TCPDF stands out:
• TCPDF (69.2 million installs): Offers extensive features, including support for complex scripts, right-to-left languages, and barcodes. It’s entirely written in PHP and doesn’t require any external libraries.
• FPDF (1.6 million installs): Lightweight and easy to use but lacks advanced features like HTML to PDF conversion.
• Dompdf (111.6 million installs): Focuses on converting HTML and CSS to PDF but can be slower and consume more memory.
• mPDF (53 million installs): Similar to TCPDF with good HTML support but can have performance issues with large documents.
Choosing TCPDF provides a balance of performance and advanced capabilities, making it suitable for robust applications.
Setting Up TCPDF in Your Project
Installing TCPDF: Step-by-Step Guide for PHP Environments
To integrate TCPDF into your project, follow these steps:
1. Install via Composer (recommended):
2. Include TCPDF in your script:
3. Create an instance of TCPDF:
Configuring TCPDF for Optimal HTML to PDF Conversion
Set up your PDF document’s properties:
Adjusting these settings ensures your PDF displays correctly with appropriate metadata.
Exploring the TCPDF API Essentials
Key methods in the TCPDF API include:
• AddPage(): Adds a new page.
• writeHTML(): Converts HTML content to PDF.
• Output(): Outputs the PDF document.
Understanding these methods is crucial for effective PDF generation.
Generating PDFs from HTML Using TCPDF
Preparing Your HTML for Accurate PDF Rendering
Creating a well-structured HTML template is essential. Below is a full HTML example for an invoice:
This template includes placeholders for dynamic data such as customer details and invoice items, with explicit tags and CSS for styling.
Implementing HTML to PDF Conversion with TCPDF Code Examples
Load your HTML content and convert it to PDF:
In the writeHTML() method, the parameters are:
• $html: The HTML content to convert.
• $ln (true): Adds a new line after the text.
• $fill (false): Indicates whether to fill the background.
• $reseth (true): Resets the last cell height.
• $cell (false): If true, treats the text as a cell.
• $align (''): Alignment of the text; empty string means default alignment.
Understanding these parameters allows fine-tuning of the PDF output.
Styling and Customizing PDFs: Tips and Tricks
Enhance the appearance of your PDFs by:
• Using CSS in the <style> tag to style elements.
• Applying custom fonts for branding.
• Adjusting layout to improve readability.
Example of adding a custom font:
Dynamic Data Integration: Generating PDFs from Database Content
Populate the invoice with dynamic content:
Assign these variables before including the HTML template so they render within the PDF.
Additional examples of dynamic content:
• Conditional Formatting: Apply different styles based on conditions.
• Dynamic Calculations: Calculate totals and taxes dynamically.
• Custom Messages: Add personalized notes.
Enhancing PDFs with Images, Fonts, and Graphics
Incorporate images into your PDF:
Parameters explained:
• $file ('images/logo.png'): Path to the image file.
• $x (15): X-coordinate position in millimeters.
• $y (10): Y-coordinate position in millimeters.
• $w (50): Width of the image in millimeters.
• $h (''): Height of the image; if empty, it maintains aspect ratio.
• $type ('PNG'): Image file type.
• $link (''): URL to link the image to.
• $align ('T'): Vertical alignment (‘T’ for top).
• $resize (false): Whether to resize the image.
• $dpi (300): Image resolution in dots per inch.
• $palign (''): Alignment of the image.
• $ismask (false): Whether the image is a mask.
• $imgmask (false): Image object returned by another call to Image().
• $border (0): Whether to draw a border around the image.
• $fitbox (false): How to fit the image into the bounding box.
• $hidden (false): If true, the image is not displayed.
Understanding these parameters allows precise placement and scaling of images.
Using Third-Party PDF APIs for Scale
As your application scales, you might face performance challenges with server-side PDF generation. In such cases, consider using third-party PDF APIs like pdforge. These services handle PDF rendering externally, reducing server load and offering additional features like concurrent processing and advanced formatting.
To integrate the PDForge API:
1. Sign Up: Register for an account at pdforge.com.
2. API Key: Obtain your API key from the dashboard.
3. API Calls: Modify your code to send HTML content to the API endpoint:
Replace 'your-template' with your actual template ID and 'your-api-key' with your API key. This method offloads PDF generation to pdforge, allowing for better scalability.
Conclusion
TCPDF is a robust solution for generating PDFs from HTML in PHP, offering extensive customization and control. It’s ideal for applications requiring intricate designs and dynamic data integration.
However, for simpler needs or when dealing with high traffic, other libraries like Dompdf or third-party PDF Generation APIs like pdforge may be more appropriate due to their simplicity or scalability. Assess your project’s specific requirements to choose the most suitable PDF generation approach.
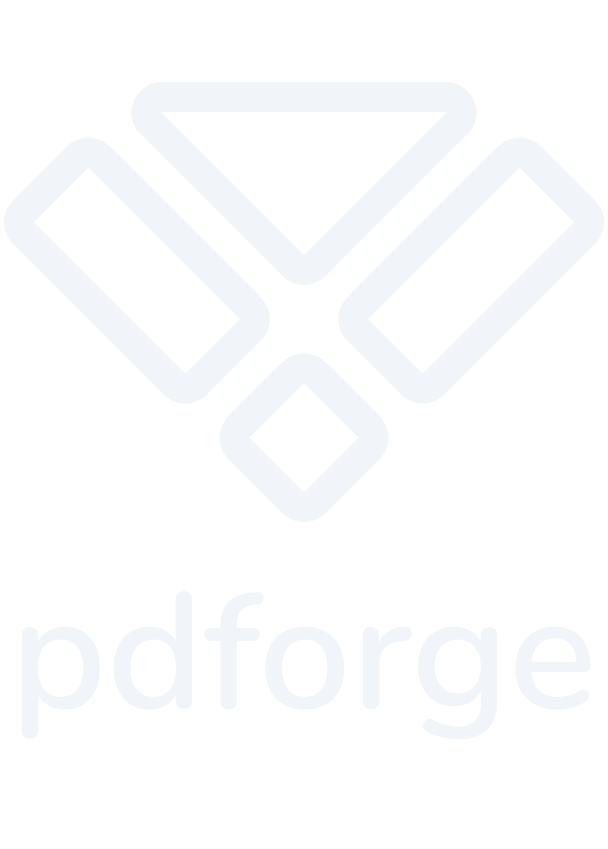
Try for free
7-day free trial