Introduction to FPDF: Converting HTML to PDF in PHP
Generating dynamic PDF reports is a common requirement for SaaS applications. Converting HTML to PDF simplifies this process by allowing developers to design reports using familiar HTML and CSS. In this article, we’ll explore how to leverage the FPDF library in PHP to transform HTML content into polished PDF documents, streamlining report generation in your application.
You can check out the full documentation here.
Comparing FPDF with Other PDF Libraries
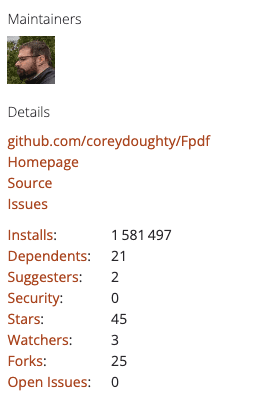
While numerous PDF libraries are available for PHP, FPDF stands out due to its simplicity and flexibility. Unlike other options that may require extensions or paid licenses, FPDF (1.6 million installs) is free, pure PHP, and doesn’t depend on additional modules. Libraries like TCPDF (69.2 million installs) and Dompdf (111.6 million installs) offer more features but can be heavier and more complex to implement. FPDF provides a lightweight alternative ideal for developers seeking a straightforward solution.
Setting Up FPDF in Your SaaS Application
Installing the FPDF Library in PHP Projects
To begin using FPDF, install it into your PHP project. You can download the library from the official FPDF website or install it via Composer:
Initial Configuration and Setup Steps
After installing FPDF, include it in your script:
Troubleshooting Common Installation Issues
If you encounter errors like “Class ‘Fpdf\Fpdf’ not found,
” ensure that your autoloader is correctly configured and that the namespace matches the installed library. Verify that all required files are accessible and that there are no permission issues on your server.
Converting HTML to PDF Using FPDF
Parsing HTML Content for PDF Generation (Full Invoice Example)
Suppose you have an HTML template for an invoice:
You’ll need to parse this HTML and replace placeholders with actual data.
Step-by-Step Guide to Implementing HTML to PDF Conversion
1. Load and Prepare the HTML Content
2. Extend FPDF to Handle HTML Content
FPDF doesn’t natively support HTML parsing, so we’ll extend it:
3. Generate the PDF
Integrating Images, Fonts, and Styles in PDFs
Adding Images
Custom Fonts
Styles
Since FPDF doesn’t support CSS files, apply styles directly within your HTML or through FPDF methods.
Using Third-Party PDF APIs for Scale
As your application scales, you might face performance challenges with server-side PDF generation. In such cases, consider using third-party PDF APIs like pdforge. These services handle PDF rendering externally, reducing server load and offering additional features like concurrent processing and advanced formatting.
To integrate the PDForge API:
1. Sign Up: Register for an account at pdforge.com.
2. API Key: Obtain your API key from the dashboard.
3. API Calls: Modify your code to send HTML content to the API endpoint:
Replace 'your-template' with your actual template ID and 'your-api-key' with your API key. This method offloads PDF generation to pdforge, allowing for better scalability.
Conclusion
FPDF provides a pragmatic lightweight solution for generating PDFs from HTML in PHP applications, offering simplicity and control. It’s ideal for straightforward reports and documents.
However, for more complex HTML rendering or high-load environments, consider alternatives like TCPDF or DOMPDF, which offer more robust HTML and CSS support.
When scalability and performance are critical, third-party PDF APIs like pdforge can handle the heavy lifting, allowing your application to maintain optimal performance.
Choose the approach that best fits your application’s needs, considering factors like complexity, scalability, and resource constraints.