How to Generate PDF from HTML Using Puppeteer in Node.js
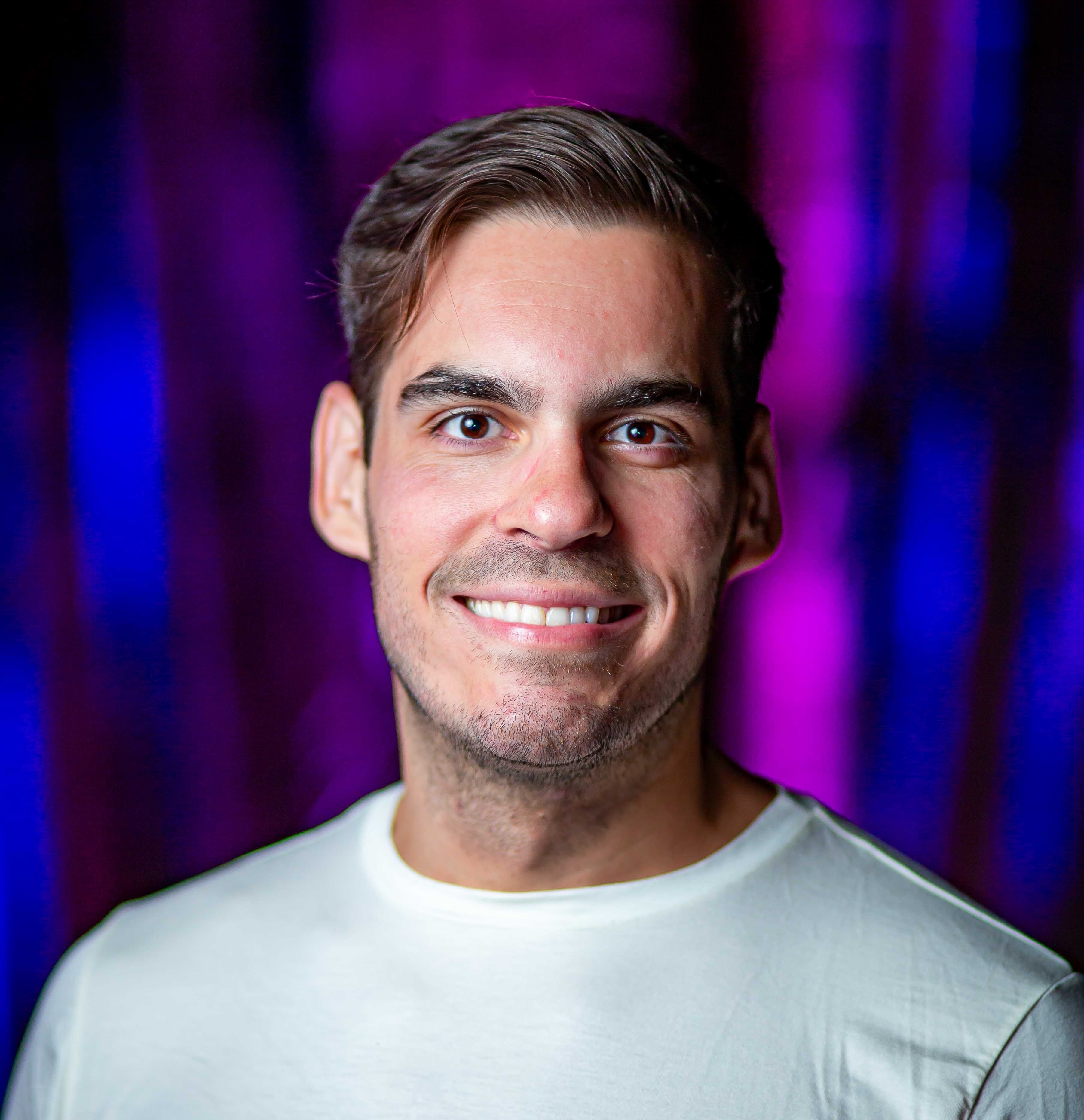
Introduction and the Growing Need for Automated PDF Reports
Automated PDF generation has become a vital component in SaaS platforms, especially when it comes to providing dynamic content like reports, invoices, and contracts. Manual generation is inefficient, and for many SaaS applications, the ability to convert HTML to a PDF programmatically is essential for scalability. Puppeteer, a headless Chrome Node.js API, enables you to automate this process with precision, handling complex layouts and modern web technologies seamlessly.
Puppeteer also has a vast documentation that you can access here.
Why It’s Recommended to Use Playwright Instead of Puppeteer
While Puppeteer remains a powerful choice, Playwright—a newer framework developed by Microsoft—has emerged as an attractive alternative. Playwright builds upon Puppeteer’s core functionality, but offers additional features like multi-browser support and better cross-platform reliability. Moreover, it handles more complex browser automation scenarios, making it an excellent option for teams looking to scale their PDF generation or run automated tests alongside their PDF workflows.
If you go to npmtrends, you can see the behaviour of users moving from Puppeteer to Playwright:
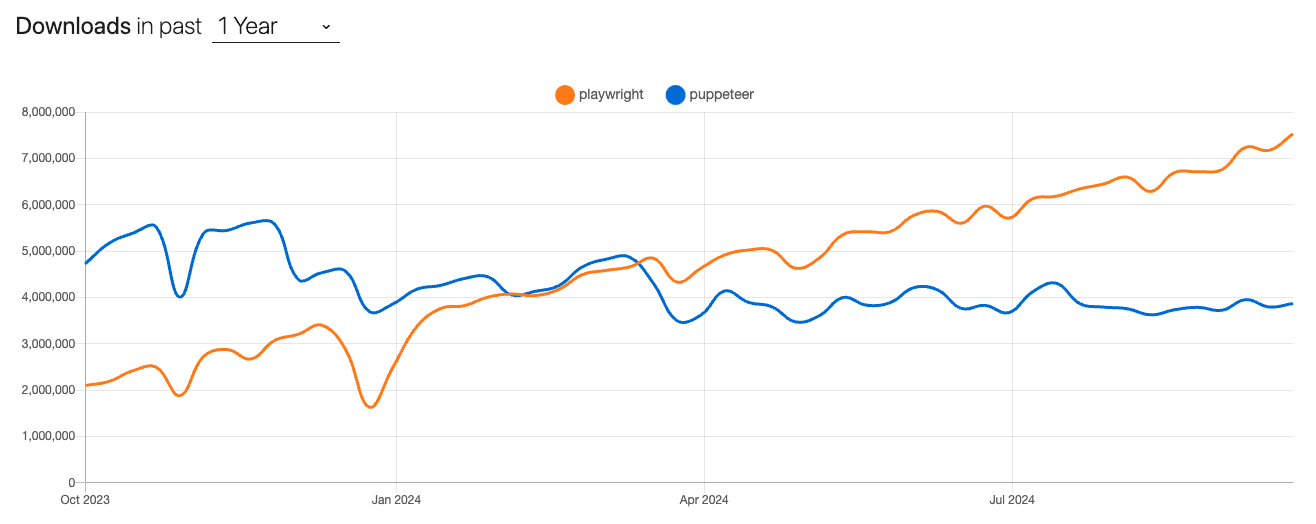
Setting Up Puppeteer in a Node.js Environment
Installing Puppeteer and Node.js: Step-by-Step Guide
To install Puppeteer, run:
Ensure Node.js is installed and set up your project structure as follows:
Exploring Puppeteer’s Capabilities for PDF Creation
Puppeteer gives you full control over rendering web content and saving it as PDFs. With built-in browser automation, it easily handles HTML, CSS, and JavaScript, making it ideal for generating dynamic documents that resemble what users see in the browser.
Converting HTML to PDF Using Puppeteer
Example HTML Report Interface
Here’s a simple HTML template representing a mocked report interface:
You can use this interface to generate a PDF using Puppeteer.
Example 1: Using an HTML File to Generate a PDF Report with Puppeteer
Example 2: Using an Internal URL of Your SaaS to Generate the PDF with Puppeteer
Key Puppeteer Methods for PDF Generation: An In-Depth Look
Puppeteer’s page.pdf()
function provides flexibility in generating PDFs. Here are the options:
- path
: The output file name for the PDF.
- format
: Paper size, e.g., `A4`, `Letter`, `Legal`.
- landscape
: Whether to print in landscape mode. Default is `false`.
- scale
: Scale of the webpage rendering (between `0.1` and `2`).
- printBackground
: Include background colors/images. Default is `false`.
- margin
: Margins for the PDF, specified as an object with `top`, `bottom`, `left`, and `right` properties.
- displayHeaderFooter
: Adds additional headers/footers, useful for page numbers.
Best Practices for Formatting and Styling HTML Before PDF Conversion
To ensure consistency in your PDFs, structure your HTML using clean and semantic code. Leverage print-specific stylesheets (@media print
) to fine-tune your document’s appearance. Avoid elements that won’t translate well in a static PDF, like videos or JavaScript-driven interactions.
Optimizing Your Puppeteer-Based PDF Stack
Making a Serverless Architecture for Scaling Requests and Memory Usage
For large-scale PDF generation, consider serverless solutions such as AWS Lambda. This enables you to process PDF requests without overloading your servers, ensuring that each request operates independently with optimal memory usage.
Debugging and Troubleshooting Common Puppeteer Issues
Use headful mode for debugging by launching Puppeteer with { headless: false }
. This lets you see what’s happening in real-time during PDF generation, especially useful when dealing with dynamic content or CSS issues.
Tips for Optimizing Performance in Large-Scale PDF Generation
Reuse browser instances to save on memory and processing time. Launching a new browser for each request is resource-intensive, especially when handling high volumes. Here’s an optimized approach:
Conclusion
Although we have other HTML-to-PDF conversion libraries, such as Playwright, pdf-lib, or jsPDF, Puppeteer is a great and versatile tool for generating PDFs from HTML in Node.js. Its headless browser lets developers automate and scale PDF creation. Whether it’s financial reports, invoices, or dynamic content, Puppeteer provides accurate rendering and flexibility, making it a key solution for SaaS platforms.
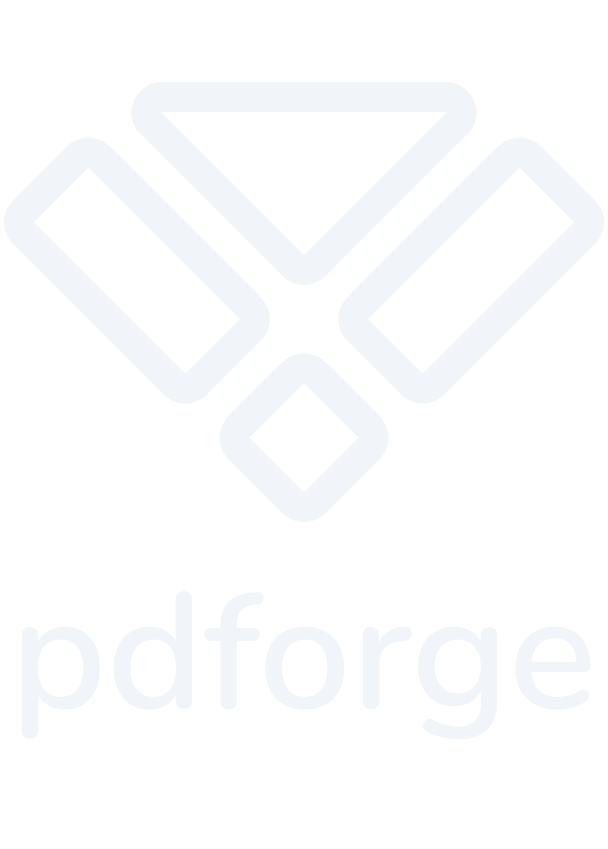
Try for free
7-day free trial