What is Playwright and How Can I Use It to Convert HTML to PDF?
Playwright is a powerful open-source automation library designed to handle browser tasks, such as testing, web scraping, and PDF generation. It supports multiple browsers, including Chromium, Firefox, and WebKit, ensuring consistent rendering of web content. Developers widely use Playwright due to its simplicity, speed, and exceptional handling of dynamic content.
When it comes to generating PDFs from HTML, Playwright stands out because it captures exactly what the browser displays—including CSS styling, JavaScript-rendered content, and complex page layouts. This ensures pixel-perfect quality, making it perfect for generating invoices, reports, or dynamic documentation.
Playwright also has well-written, robust documentation that you can use if you run into any trouble.
If you're using other programming languages, check out our other guides:
Alternative PDF Libraries: How Playwright Compares to Other Tools
Several JavaScript alternatives exist for PDF generation:

Puppeteer: Similar to Playwright but supports only Chromium browsers.
jsPDF: Lightweight and easy-to-use, but limited with complex CSS and JavaScript rendering.
pdf-lib: Great for PDF manipulation, but lacks HTML rendering capabilities.
pdfmake: Useful for structured document creation, less flexible with complex HTML/CSS.
pdfkit: Primarily for simple PDF documents, struggles with complex layouts and dynamic content.
react-pdf: Ideal for React applications but not suited for general-purpose HTML-to-PDF rendering.
Playwright has recently seen skyrocketing popularity in downloads and community support according to npmtrends data. Its flexibility, browser compatibility, and exceptional handling of dynamic content have contributed significantly to its growing adoption.
If you want to dig deeper on a comparison between playwright and other javascript pdf libraries, we also have a detailed article with a full comparison between the best PDF libraries for NodeJs in 2025.
Step 01: Setting Up Playwright for HTML to PDF Conversion
First, ensure Node.js is installed on your machine. If not, download it from nodejs.org.
Then, install Playwright via npm:
This command automatically includes all necessary browser engines (Chromium, Firefox, WebKit).
Step 02: Generating Your First "Hello World" PDF
Creating a PDF with Playwright involves running a simple Node.js script. Let's create our first basic example:
Alternative 01: Generating PDF from a URL
Here’s how you can generate a PDF from any URL:
This script:
Opens a headless Chromium browser
Navigates to your chosen URL
Saves the resulting PDF
Alternative 02: Generating PDF from an HTML File
If you already have an HTML file or a raw HTML string, follow these steps:
Directly using a string without a file:
Playwright Print Configurations (Footer, Header, Margins, etc.)
Playwright allows detailed customizations, such as setting headers, footers, margins, and more:
Refer to the Playwright Print Configuration documentation for more detailed options.
Using HTML Template Engines
For dynamic HTML generation, template engines like Handlebars, EJS, or Pug can streamline the process. Here’s an example using Handlebars:
Step 03: Creating a Serverless Architecture to Scale PDF Generation
Playwright can be resource-intensive, consuming significant memory and processing power when rendering complex HTML content. To efficiently handle high volumes of PDF generation requests, using a serverless architecture like AWS Lambda is highly beneficial. This approach reduces costs by scaling automatically and only consuming resources when needed.
Note that you must install the browser engine (e.g., Chromium) on the Lambda layer or any other serverless service you're using. While various installation guides exist online, what worked best for me was using a dockerized container to install the browser engine effectively.
I’ve prepared a detailed step-by-step guide to help you set this up, available here.
Alternative: Scale PDF Generation with Third-Party APIs
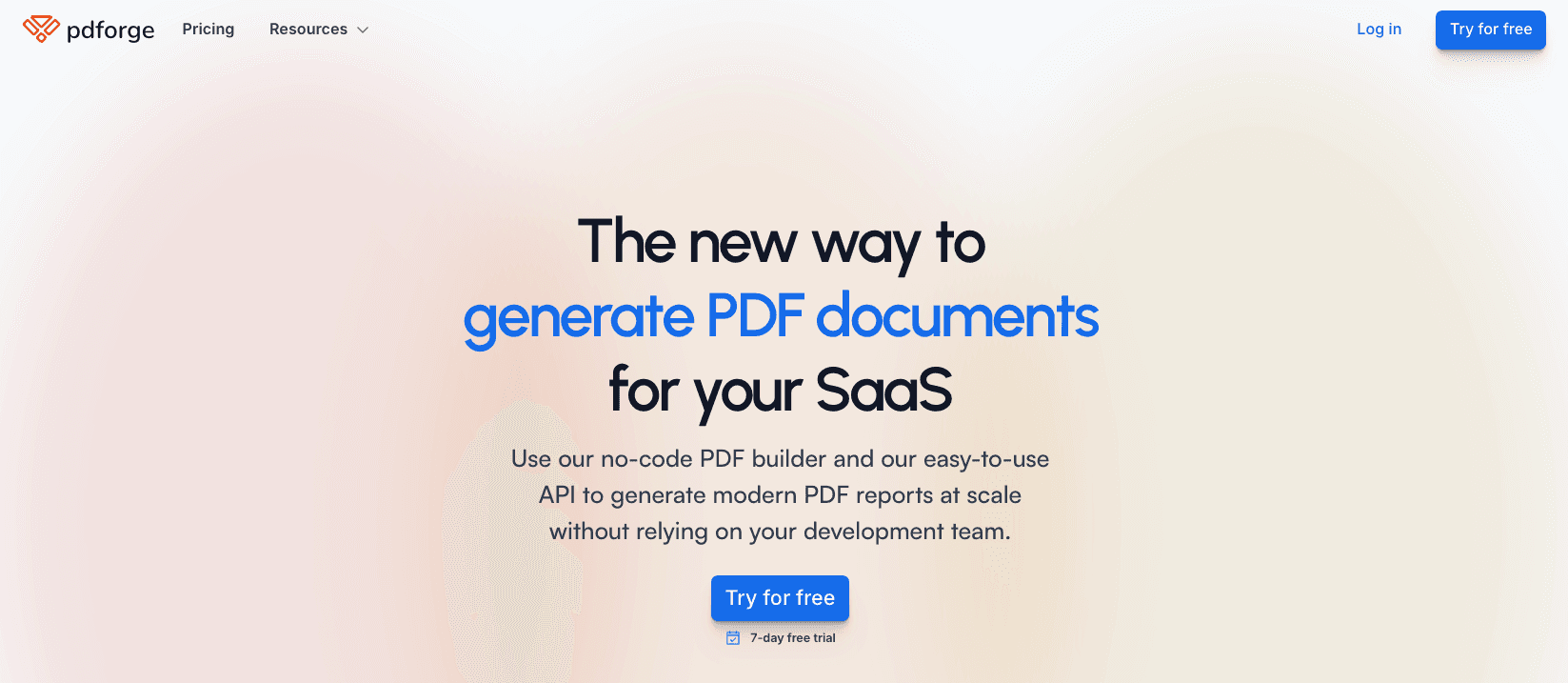
For larger SaaS platforms requiring automated PDF generation at scale, if you're developing using no-code platforms like Bubble or creating automation workflows using platforms like n8n, make or zapier, integrating a PDF Generation API like pdforge can offload the heavy lifting.
With pdforge, you can create beautiful PDF documents in minutes using our AI-first PDF Generation. You can fine-tune de design with an easy-to-use opinionated no-code builder and start generating PDFs using our API or native integration with no-code tools. Let the AI do the heavy lifting by generating your templates, creating custom components or even filling all the variables for you.
You can handle high-volume PDF generation from a single backend call.
Here’s an example of how to generate pdf with pdforge via an API call:
You can create your account, experience our no-code builder and create your first layout template without any upfront payment clicking here.
If you prefer a managed service, third-party APIs like pdforge offer scalable HTML-to-PDF generation without infrastructure setup or design changes management from you. These solutions manage everything from HTML template rendering to PDF delivery.
Conclusion
Playwright is a robust, versatile tool for generating PDFs from HTML. Its ability to render pixel-perfect PDFs, combined with rapidly increasing popularity, positions it as an exceptional solution for generating high-quality PDFs with complex layouts at scale.
If you don't want to waste time maintaining pdfs layouts and their infrastructure or if you don't want to keep track of best practices to generate PDFs at scale, third-party PDF APIs like pdforge will save you hours of work and deliver a high quality pdf layout.