Introduction to PDFKit: A Reliable PDF Library for Node.js
PDFKit is a powerful open-source library for generating PDF documents in Node.js. Unlike many PDF libraries, PDFKit operates with both flexibility and precision, allowing developers to build documents programmatically or by rendering existing HTML. It supports embedding images, fonts, and CSS styles, making it ideal for complex document layouts.
You can check out the full documentation here.
Alternative PDF Libraries: How PDFKit Compares to Other Tools
While PDFKit is a great tool, it's important to be aware of alternatives.
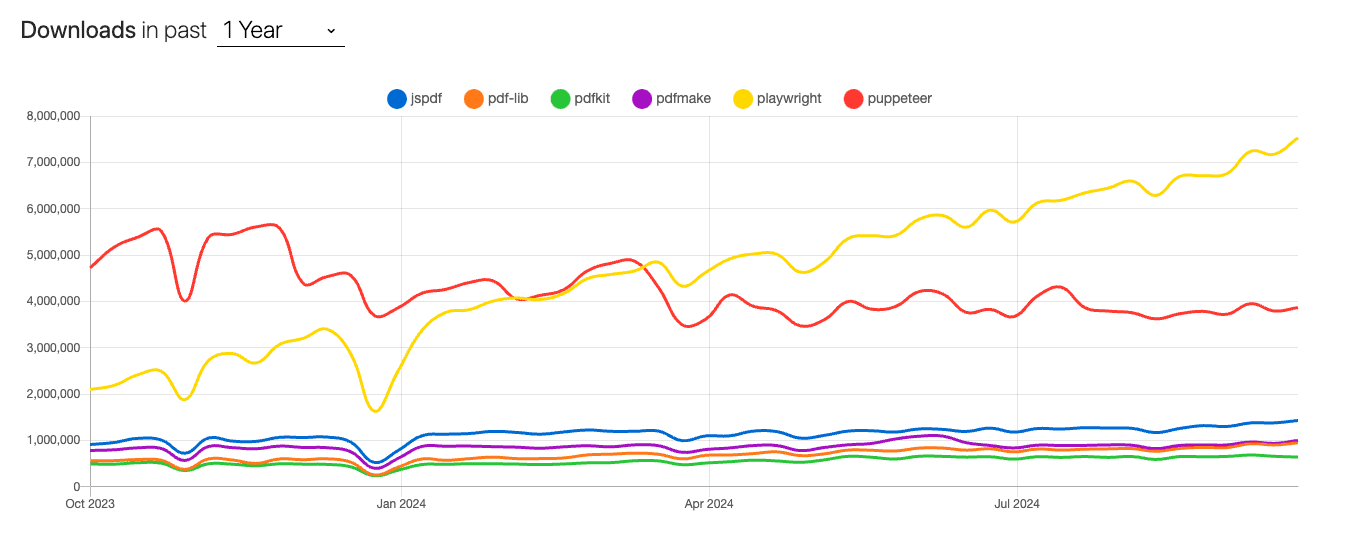
Libraries like Playwright or Puppeteer are widely used for rendering HTML into PDFs via headless browser instances. These tools offer greater accuracy in rendering CSS and are excellent for visually rich documents but come at the cost of increased resource usage.
In contrast, PDFKit’s lightweight nature makes it a good fit for projects where performance and simplicity are key, especially for backend services or API-based PDF generation. Other good alternative to PDFKit with the same characteristics is jsPDF.
If you want to go deep on a full comparison between pdf libraries in javascript for 2025, you can check out this guide.
Setting Up PDFKit in Node.js
Before diving into generating PDFs, let’s get PDFKit up and running in your Node.js environment. Below is a step-by-step guide to install and configure the library.
Installing PDFKit: Step-by-Step Guide for Node.js
To get started, initialize your Node.js project and install PDFKit using npm:
Once installed, require the library in your project:
Creating a Basic PDF Document with PDFKit
To create a basic PDF, instantiate a new `PDFDocument` and pipe the output to a file:
This will generate a simple PDF containing the text "Hello, PDFKit!". However, the true power of PDFKit comes into play when you start generating more complex documents, especially when rendering HTML content.
Structuring Your HTML for Seamless PDF Generation
Structuring HTML effectively is essential for clean conversion to PDF. When preparing HTML for conversion, it's critical to use a layout structure that works well with both PDFKit and CSS rendering engines. A typical structure for an invoice might look like this:
Converting HTML to PDF with PDFKit: A Practical Guide
Now that we’ve structured our HTML, we can move on to converting it to a PDF document using PDFKit.
Rendering HTML in PDF Format: Key Techniques and Best Practices
PDFKit doesn’t directly convert HTML to PDF like some tools, but it allows you to build the PDF programmatically. To achieve an HTML-to-PDF workflow, we can use libraries like html-to-pdf
to parse the HTML and render it with PDFKit. This involves first generating HTML and then programmatically placing that content into a PDFKit document.
Handling CSS and Media Queries in HTML to PDF Conversion
When converting HTML to PDF, PDFKit requires that you take special care with CSS. Inline styles and media queries may not always behave as expected. It's essential to keep CSS minimal and use PDF-friendly layouts. Avoid complex flexbox or grid layouts that may not render well outside of a browser context.
Embedding Images and Fonts with PDFKit
PDFKit supports embedding custom fonts and images to enhance the PDF layout:
Customizing Page Layouts: Headers, Footers, and Margins in PDFKit
You can also customize headers, footers, and margins for each page in your PDF:
PDF Security and Encryption
PDFKit enables you to encrypt PDFs, adding an extra layer of security to sensitive documents:
How to Handle Large HTML Files and Pagination in Node.js
When dealing with large HTML files, pagination becomes a key consideration. PDFKit can handle automatic pagination, ensuring content is split across pages without breaking mid-section:
Troubleshooting Common Issues
1. Images Not Showing?
Make sure your image paths are correct relative to the current working directory, and that you’ve loaded the correct format (JPEG/PNG).
2. Text Overlapping or Layout Issues?
Use `doc.moveDown()` or custom line spacing. Check if you’re specifying a `width` for text blocks. For HTML-based flows, ensure inline CSS is minimal.
3. Large PDF Output Size?
Optimize images, consider using standard fonts, or compress embedded images to reduce the file size.
How to Use a PDF API to Automate PDF Creation at Scale
For larger SaaS platforms requiring automated PDF generation at scale, integrating a PDF Generation API like pdforge can offload the heavy lifting. This approach is ideal for SaaS platforms with high volumes of PDF requests.
With pdforge, you can create beautiful reports with flexible layouts and complex components with an easy-to-use opinionated no-code builder. Let the AI do the heavy lifting by generating your templates, creating custom components or even filling all the variables for you.
You can handle high-volume PDF generation from a single backend call.
Here’s an example of how to generate pdf with pdforge via an API call:
You can create your account, experience our no-code builder and create your first layout template without any upfront payment clicking here.
Conclusion
PDFKit shines when flexibility and programmatic control are needed in PDF generation. It’s perfect for backend services or Node.js-based SaaS products. However, choosing the right tool depends on your specific requirements and scalability needs.
If CSS fidelity or browser-like rendering is crucial, tools like Playwright or Puppeteer might be a better fit.
If you don't want to waste time maintaining pdfs layouts and their infrastructure or if you don't want to keep track of best practices to generate PDFs at scale, third-party PDF APIs like pdforge will save you hours of work and deliver a high quality pdf layout.