Generating PDFs from HTML with jsPDF: The Best Practices
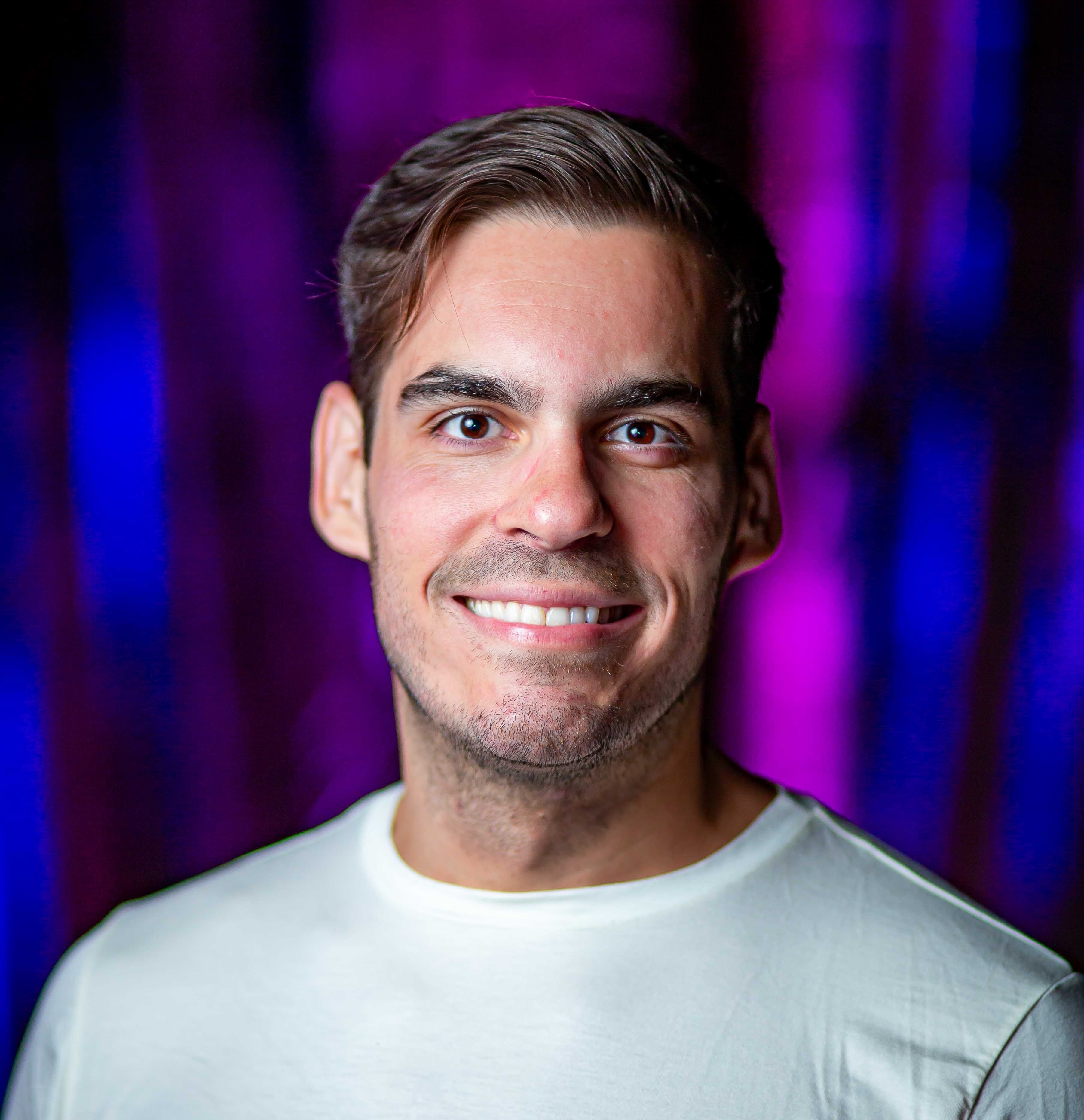
An Introduction to the javascript pdf generation library: jsPDF
Creating downloadable PDFs in web applications is essential for many SaaS platforms, whether it’s generating invoices, reports, or summaries. One tool that developers often turn to for this is jsPDF, a lightweight library that allows PDF generation on the client side without relying on a backend.
You can get to the full documentation here.
Why Use jsPDF When Building PDFs for Your Application?
jsPDF is the most popular PDF library on npm for generating PDFs purely in JavaScript.
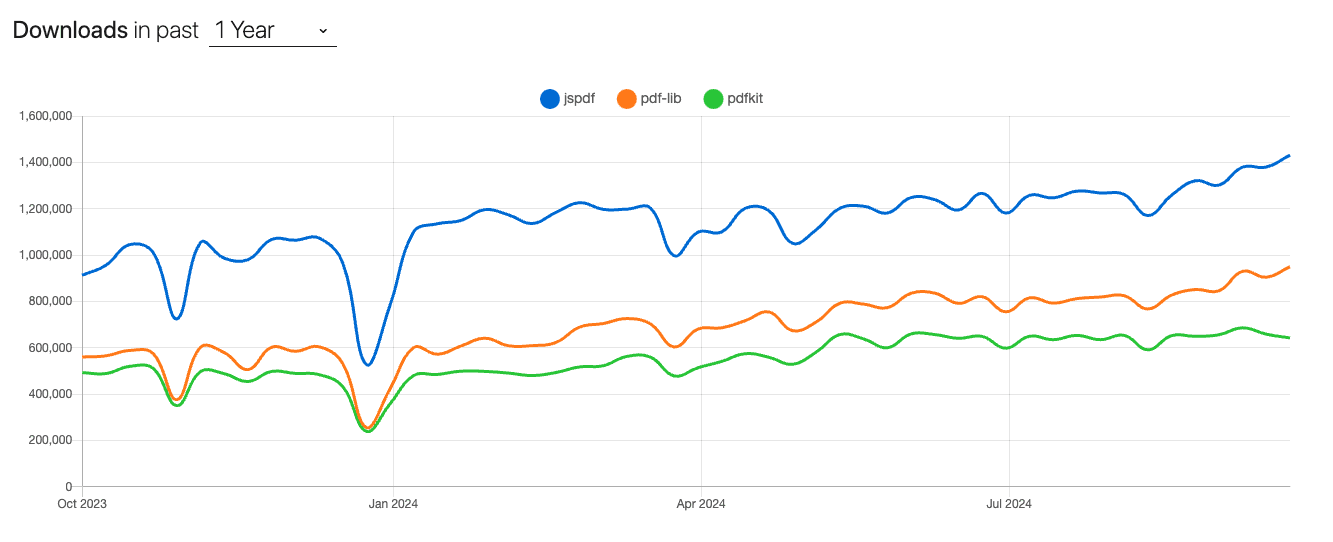
Its client-side capabilities make it a great option for lightweight use cases where server resources are limited. But it's important to note that jsPDF is a low-level solution. Alternatives like Playwright and Puppeteer are more robust and versatile, as they can automate full web page rendering to PDF, including complex layouts and media, making them preferable for applications requiring more sophisticated PDF generation.
Setting Up jsPDF for HTML to PDF Conversion
How to Install jsPDF in Your JavaScript Project
Getting started with jsPDF is straightforward. You can install it using npm or directly include it in your HTML.
Or by including the CDN:
Here's a basic example of generating a PDF:
Key Features of jsPDF for Developers with Examples
Custom Text: Control fonts and colors:
Image Support: Embed images directly into the PDF:
Tables: Utilize the AutoTable plugin for structured data:
Best Practices for Generating PDFs from HTML with jsPDF
Structuring Your HTML File for Clean PDF Outputs
When converting HTML to PDF, keeping your HTML clean and structured is essential for accurate rendering. Here's an example of well-organized HTML and CSS that ensures clean outputs:
This minimal HTML, paired with CSS for layout and styling, ensures that the structure will remain consistent when converted into a PDF using jsPDF.
Using JavaScript to Style and Format PDF Content
With jsPDF, you can replicate the appearance of your HTML using JavaScript. However, it's important to apply styles manually, as CSS isn't directly transferred. Here's a simple way to apply text styles:
Leveraging jsPDF Plugins for Enhanced PDF Generation
For advanced layouts like tables, charts, or large datasets, jsPDF plugins like AutoTable are indispensable. They help structure data without manually positioning every element.
Handling Large HTML Documents Efficiently with jsPDF
Handling large HTML documents can be tricky with jsPDF, but breaking the content into smaller sections and generating them in steps can mitigate performance issues.
Here’s a way to handle long content by generating separate sections and merging them:
Transforming HTML to PDF with jsPDF and html2canvas
The html2canvas library is a powerful tool that captures HTML elements and renders them into canvas images, which can then be embedded into PDFs using jsPDF.
This approach allows for a more accurate representation of complex HTML layouts, including CSS styles and images, which jsPDF alone might not fully support. Here’s how you can use html2canvas with jsPDF to convert HTML to PDF:
In this example, html2canvas captures the HTML element with the ID content
, converts it into an image, and then jsPDF embeds that image into the PDF. This technique is ideal for preserving the exact look and feel of your HTML content, including complex layouts, images, and CSS.
HTML Template Engines
Using an HTML template engine like Handlebars can streamline the generation of dynamic PDFs by templating your HTML and filling it with data programmatically.
Here’s a basic example using Handlebars:
Optimizing PDF Performance and Integration in SaaS Applications
Reducing PDF File Sizes for Better Performance
When generating PDFs in a SaaS environment, file size can impact both user experience and system performance. To reduce PDF sizes, compress embedded images and limit the use of high-resolution assets. For example, use image compression before embedding:
Reducing font usage and minimizing graphical elements can also result in smaller, faster PDFs.
How to Use a PDF API to Automate PDF Creation at Scale
For SaaS platforms, automating PDF generation at scale might require offloading the heavy lifting to a PDF API. By integrating APIs like pdforge you can handle high-volume PDF generation, complex formatting, and post-processing, all from a single backend call.
These APIs handle HTML to PDF conversion more efficiently than jsPDF for large-scale, complex use cases.
Conclusion
While jsPDF offers a solid, low-level approach for generating PDFs from HTML within client-side JavaScript, it does come with limitations. It’s great for small to medium-sized tasks but can feel cumbersome for larger or more dynamic documents. For a more developer-friendly experience, especially when dealing with more complex requirements, tools like Playwright and Puppeteer are far more capable, offering full web page rendering and a more streamlined workflow for generating PDFs in modern SaaS applications, or using pdforge api for pdf creation at scale.
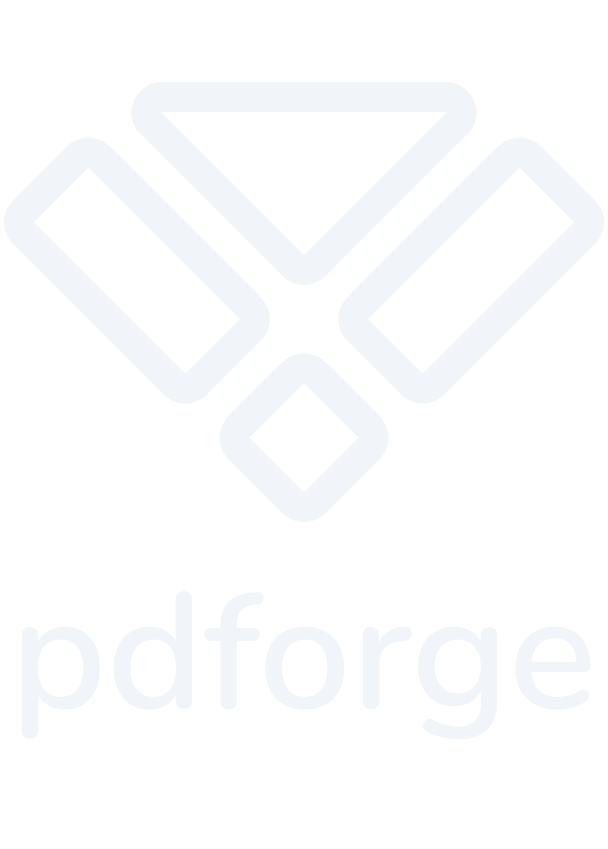
Try for free
7-day free trial