Introduction: Why Use PDFMake for HTML to PDF Conversion in SaaS
For SaaS applications, generating PDF reports is essential for delivering structured, portable, and printable documents to users. PDFMake, a versatile pdf library, allows developers to build custom PDF documents directly from JavaScript objects. Its flexibility makes it an attractive choice for creating tailored reports, invoices, or any document that requires a standardized format, giving developers precise control over content and layout without relying on browser-based rendering.
You can check the full documentation here.
Alternative PDF Libraries: How PDFMake Compares to Other Tools
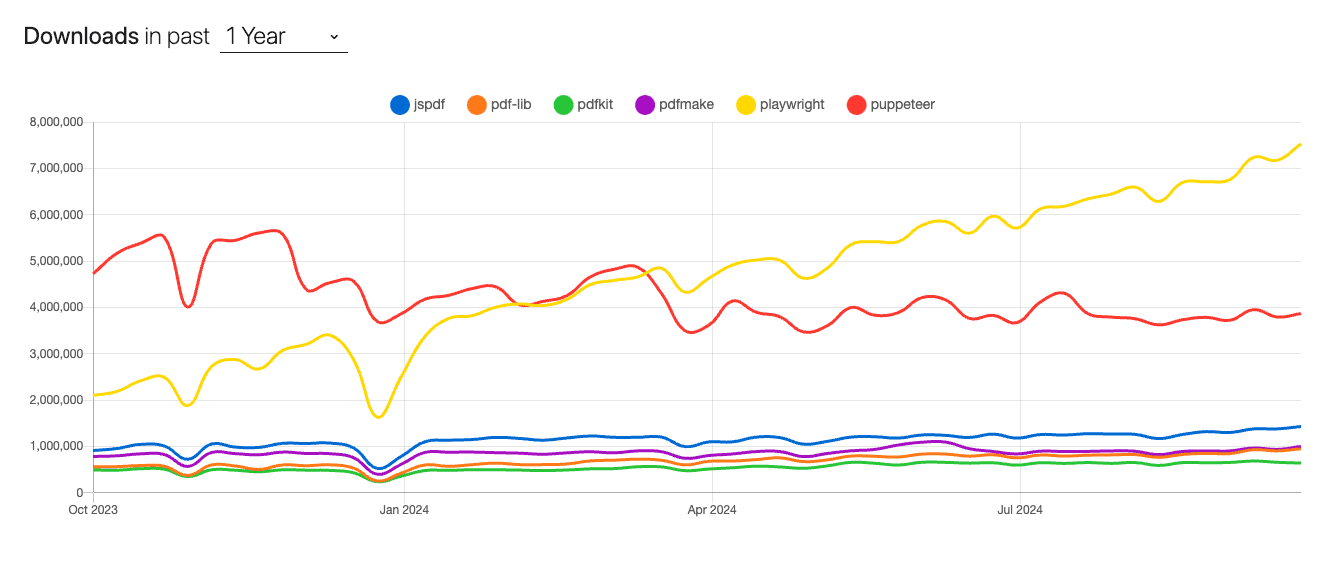
When comparing PDF generation tools, PDFMake falls into the category of low-level libraries like jsPDF, where developers define PDF structure programmatically using a JSON-like document object. On the other hand, high-level tools such as Playwright and Puppeteer operate by capturing a rendered HTML page through a headless browser.
While high-level libraries are often easier for rendering complete web pages into PDFs, PDFMake excels in scenarios where fine-grained control over the document structure and layout is required.
If you want to dig deeper on a comparison between playwright and other javascript pdf libraries, we also have a detailed article with a full comparison between the best PDF libraries for NodeJs in 2025.
Setting Up Your Environment for PDFMake and Node.js
Installing PDFMake and Node.js for Seamless Integration
To start building PDFs, ensure that you have Node.js installed. Then, integrate PDFMake into your project by installing it with npm:
This command adds PDFMake to your project, allowing you to programmatically generate PDFs from within a Node.js environment. With this setup, you can begin assembling PDFs using JavaScript objects.
Setting Up Your Project Structure for HTML to PDF Conversion
To demonstrate how PDFMake can replicate an HTML document, let’s use an example of an invoice written in plain HTML and CSS:
This simple invoice file will serve as our reference for building a similar layout using PDFMake. Since PDFMake doesn't render HTML directly, we must replicate the structure programmatically.
Converting HTML to PDF with PDFMake: Step-by-Step Guide
Styling Your PDFs: How to Replicate HTML and CSS Styles Using PDFMake
Since PDFMake doesn’t convert raw HTML into PDFs, you need to define the content structure programmatically. Let’s start by replicating the invoice’s heading and text:
This structure defines the header and customer details, mimicking the HTML’s layout. You can add custom fonts and styles as needed.
Using PDFMake’s Table and Layout Features for Structured Reports
Next, we’ll convert the invoice table to PDFMake’s table format. PDFMake provides a simple API for creating tables and specifying cell alignment, similar to HTML tables:
Here, the body
attribute defines the rows and cells of the table, effectively replicating the HTML table structure.
Adding Images, Headers, and Footers Using PDFMake’s Features
To complete the invoice, you can add a company logo or other images using base64 or a URL reference, and customize headers and footers for page numbers or branding:
Creating Interactive PDFs: Adding Links and Form Fields
For interactivity, PDFMake supports hyperlinks and form fields, allowing you to create more engaging documents. You can add links to your invoices for online payment options:
Debugging and Troubleshooting Common Issues in HTML to PDF Conversions
PDFMake doesn’t parse or render HTML. This is both a benefit and a limitation—on the one hand, you have full control over how your document is structured, but on the other, you must manually map HTML content to PDFMake’s syntax. Common issues include incorrect layout rendering or broken table structures. Always ensure that your data structure matches the expected input of PDFMake’s API.
How to Use a PDF API to Automate PDF Creation at Scale
For SaaS platforms generating PDFs at scale, you may need to offload the process to a PDF API. Third-party solutions like pdforge offer robust APIs for automating bulk PDF generation. By sending the structured data to an API, you can automate PDF creation without the need to manage resources directly.
Conclusion
While PDFMake offers an excellent solution for generating highly customizable PDFs with fine-grained control, it’s best suited for cases where you need to build documents from structured data. For more complex layouts or when replicating entire web pages, higher-level tools like Playwright or Puppeteer are preferable due to their ability to render PDFs based on browser rendering. For large-scale PDF generation, consider using third-party PDF Generation APIs, such as pdforge, to automate the process, ensuring performance and scalability.