How to Generate PDF Reports from HTML with React-PDF
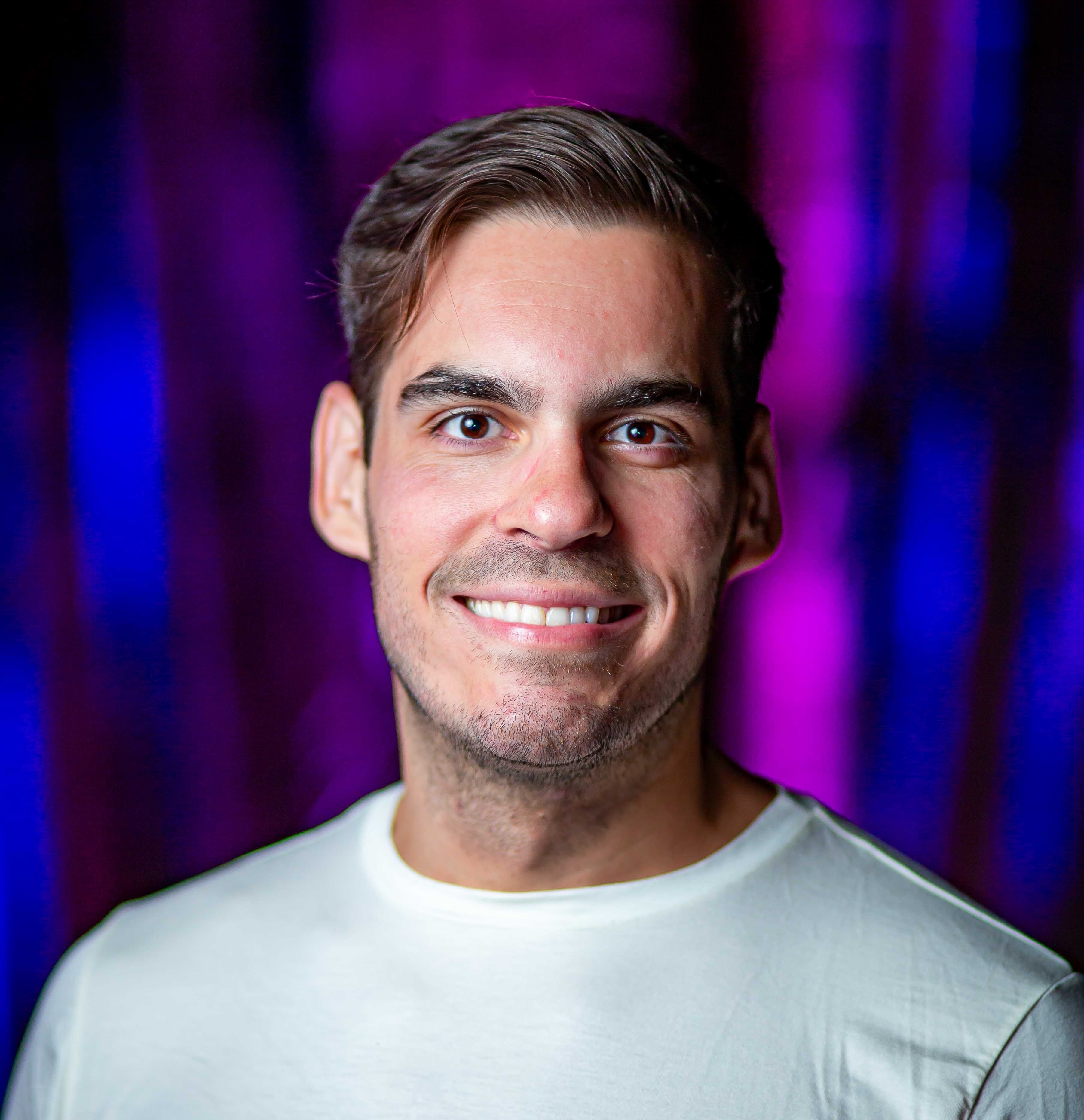
Overview of React-PDF: A Powerful PDF Library for React
Generating PDF reports from HTML content is a ubiquitous need in modern SaaS applications, especially for delivering invoices, receipts, and detailed analytics to users. React-PDF is a robust PDF library for React that empowers developers to create intricate PDF documents using familiar React components. Built on top of PDFKit, React-PDF leverages this solid foundation to provide a declarative and intuitive API for PDF generation.
They have a well structured documentation that you can check out here.
Comparing React-PDF with Other PDF Libraries
When selecting a PDF generation tool, it’s essential to understand how React-PDF stacks up against other libraries. PDF tools generally fall into two categories: canvas-like libraries and browser-oriented libraries.
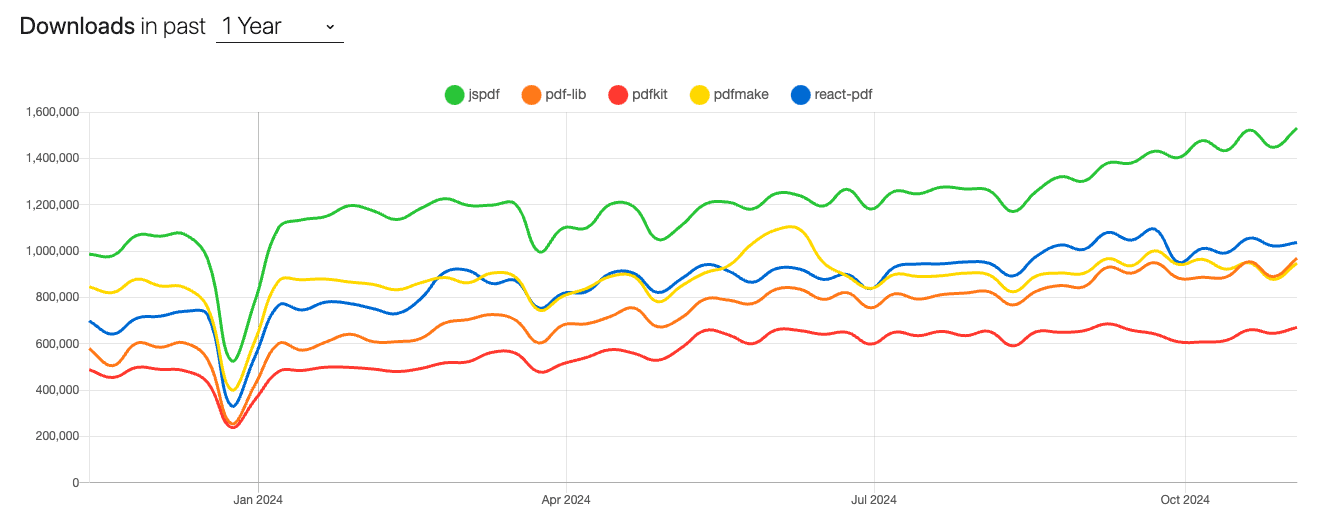
Canvas-Like PDF Libraries:
pdf-lib: A lightweight library for creating and modifying PDFs in JavaScript without external dependencies.
PDFKit: A feature-rich PDF generation library for Node.js, offering low-level control over PDF creation.
pdfmake: Allows creation of PDFs using a declarative syntax, suitable for both Node.js and browser environments.
jsPDF: A client-side library for generating PDFs directly in web browsers.
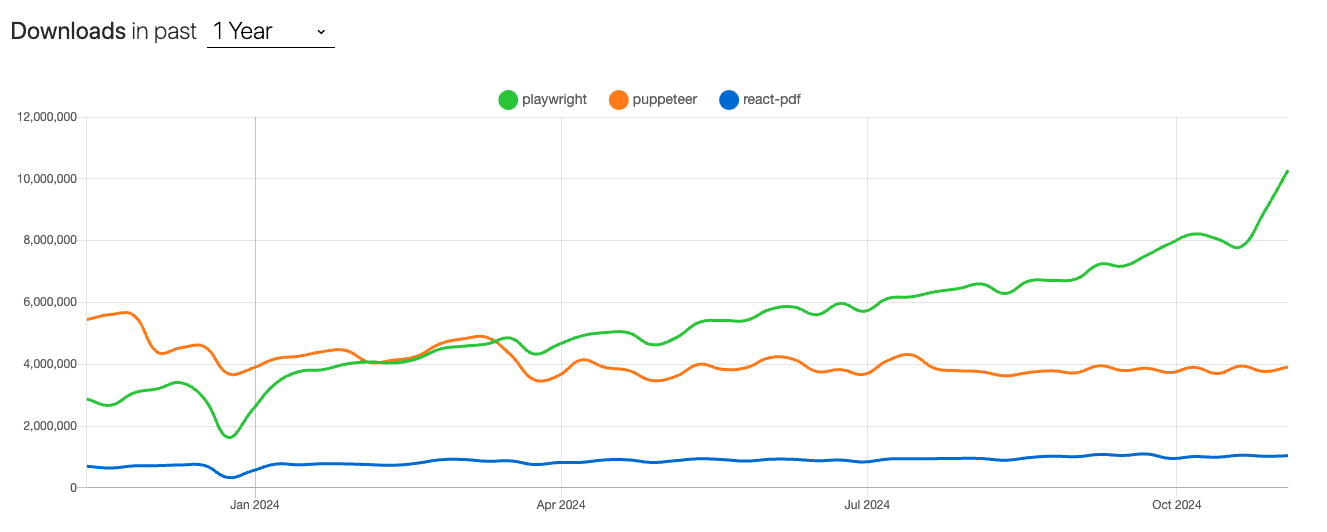
Browser-Oriented Libraries:
Puppeteer: A Node.js library providing a high-level API to control Chrome or Chromium over the DevTools Protocol.
Playwright: Similar to Puppeteer but supports multiple browsers, including Chromium, Firefox, and WebKit.
React-PDF distinguishes itself by combining the declarative nature of React with the power of PDFKit. This synergy allows for seamless integration within React applications, making the process of converting HTML to PDF more intuitive and maintainable.
Setting Up Your Project with React-PDF and Node.js
To get started with React-PDF, ensure that Node.js and npm are installed on your machine. Begin by creating a new React application:
Install React-PDF and its necessary dependencies:
Project Folder Structure
Organizing your project enhances scalability and maintainability. A recommended folder structure might look like this:
components/: Contains all React components, including your PDF templates.
data/: Holds data files or mocks that feed into your components.
App.js: The main application file where components are assembled.
Building the HTML Template and Styling for PDF
Let’s create a complete invoice template as an example. In src/components/Invoice.js, build the PDF document using React-PDF components:
This template demonstrates how to create a dynamic HTML structure for PDF content using React components. Styling is managed through StyleSheet, ensuring consistency across the document. Responsive layouts are handled using Flexbox properties, and images and fonts are optimized by registering only necessary assets.
Generating and Exporting PDFs with React-PDF
In src/App.js, render and export the PDF document:
The PDFDownloadLink component provides a seamless way to export PDFs on the frontend, enhancing the user experience.
To incorporate custom data from JavaScript, update src/data/invoiceData.js:
Exporting PDFs on the Backend with Node.js
For server-side PDF generation, which is crucial for automating PDF creation at scale, set up a Node.js backend using ESM modules. Install the necessary package:
Configure your server in server.js:
Error Handling and Troubleshooting Common Issues with React-PDF
Common issues you might encounter with React-PDF include:
Font Rendering Problems: Ensure fonts are correctly registered and accessible. Use supported font formats like WOFF or TTF.
Styling Limitations: React-PDF does not support all CSS properties. Refer to the documentation for supported styles and utilize Flexbox for layout control.
Image Embedding Errors: Use absolute URLs or properly import images to ensure they render in the PDF.
Large PDF Sizes: Optimize images by compressing them and include only necessary fonts to reduce file size.
Utilize React-PDF’s API and error messages for effective debugging. Implement try-catch blocks and console logging to handle exceptions gracefully.
How to Use a PDF API to Automate PDF Creation at Scale
For SaaS platforms, automating PDF generation at scale might require offloading the heavy lifting to a PDF API.
It's also an option to integrate with third-party APIs like pdforge you can handle high-volume PDF generation, complex formatting, and post-processing, all from a single backend call.
Here’s an example of how to integrate pdforge in Rails to convert HTML content into a PDF via an API call:
This code sends a POST request to the pdforge API, receives the generated PDF, and saves it locally.
Conclusion
React-PDF offers a powerful solution for generating PDF reports from HTML in React applications. Its integration with PDFKit and use of React components make it an excellent choice for developers seeking a declarative and intuitive approach.
However, React-PDF may not suit all scenarios. If you require low-level control over PDF structures or need to convert complex HTML directly, alternatives like pdf-lib or browser-oriented libraries like Puppeteer and Playwright might be more appropriate.
For large-scale applications demanding advanced features and scalability, leveraging third-party PDF APIs such as pdforge is recommended. These services handle the complexities of PDF generation, allowing you to focus on your application’s core functionality.
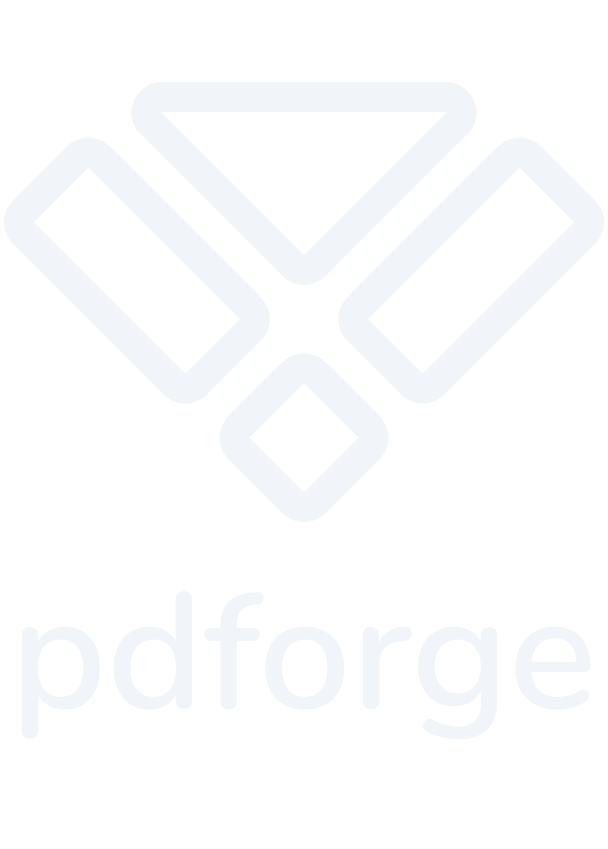
Try for free
7-day free trial