How to generate PDF from HTML Using Python-PDFKit
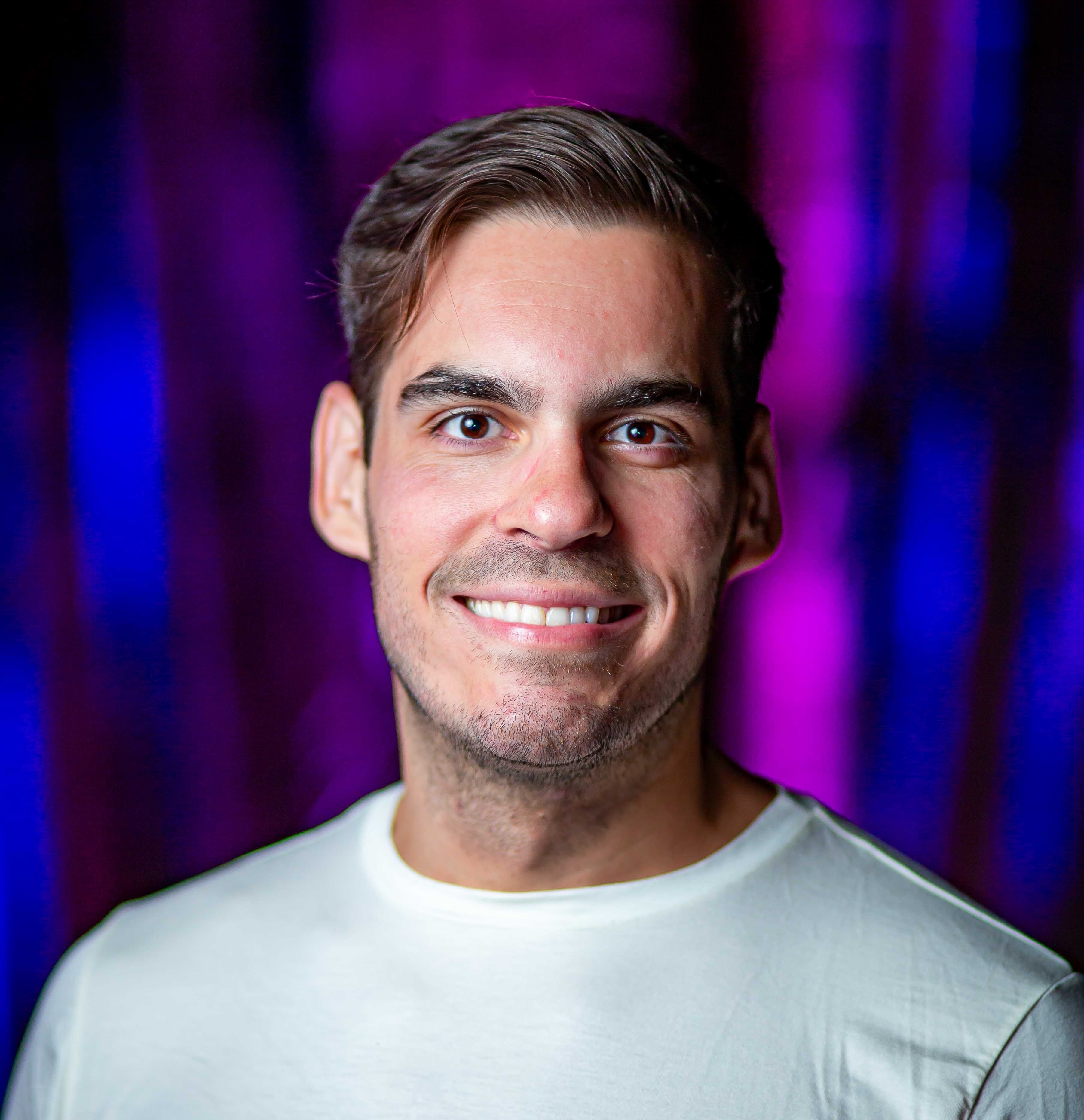
An Introduction to PDFKit: a Python PDF Generation Library
PDFKit is a popular Python library that simplifies the process of converting HTML to PDF, providing an easy way to style your documents using familiar web technologies like HTML and CSS. In this guide, we’ll walk through setting up PDFKit, configuring it to generate PDF documents from HTML, and even handling more advanced use cases like dynamic content and asynchronous generation.
You can check out the pypi documentation here.
Comparing PDFKit with Other Python PDF Libraries
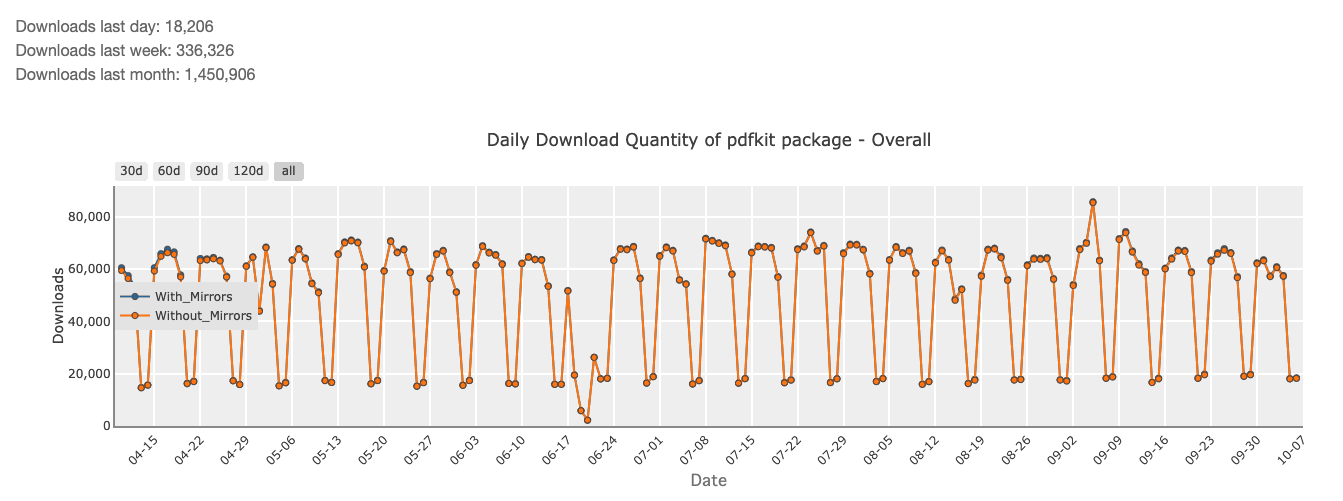
There are many Python libraries available for generating PDFs. Libraries like ReportLab (4,788,417 monthly downloads) and PyPDF2 (9,982,763 monthly downloads) offer powerful document creation tools, but they often require manually defining document structure and layout. This can become cumbersome, especially if you’re more familiar with HTML and CSS.
PDFKit, on the other hand, excels by leveraging wkhtmltopdf, which converts HTML content directly into PDF. This allows you to use existing HTML templates, making it much easier to maintain and style your documents. While other libraries might provide lower-level control over PDF creation, PDFKit offers simplicity, leveraging familiar web technologies.
Setting Up Python-PDFKit for HTML to PDF Conversion
Installing PDFKit and Dependencies for a Smooth Setup
To start using PDFKit, you’ll first need to install both the pdfkit Python package and the wkhtmltopdf binary, which handles the heavy lifting of converting HTML to PDF. Start by installing the necessary dependencies:
For wkhtmltopdf, you’ll need to install it separately based on your OS. On Ubuntu, for instance, you can install it via:
On macOS, you can use Homebrew:
Once both are installed, PDFKit is ready to use. If you encounter issues with the installation, ensure that wkhtmltopdf is correctly configured in your system’s PATH.
Configuring wkhtmltopdf: The Engine Behind Python-PDFKit
wkhtmltopdf is the core engine that powers PDFKit, translating your HTML and CSS into a PDF file. For a smooth experience, make sure you configure the path to wkhtmltopdf correctly in your code. You can set the path manually if necessary:
By explicitly defining the path, you avoid potential issues with the binary not being found, especially in different environments like Docker or cloud servers.
Key Features of Python-PDFKit You Should Know
PDFKit allows you to generate PDFs from URLs, strings, or files. It provides extensive options for customizing the conversion process, such as setting margins, page sizes, and header/footer content.
Some key features include:
• Ability to convert HTML files, strings, or web pages.
• Support for custom page settings, like orientation and margins.
• Options for embedding metadata, like title, subject, and author.
• Advanced control over CSS for precise styling.
Essential Python Code Snippets to Convert HTML to PDF
Here’s an example of converting a simple HTML file to PDF using PDFKit:
With just a few lines of code, PDFKit handles the heavy lifting of transforming your HTML content into a polished PDF document.
Step-by-Step Guide: Generating PDFs from HTML Using PDFKit
Creating a Complete Invoice HTML/CSS File for Example
Let’s walk through creating an invoice PDF from an HTML template. Below is a basic example of an HTML invoice:
This HTML template defines the structure of the invoice and includes placeholders for dynamic content such as the invoice date and number.
Using PDFKit to Render HTML and Convert It to a PDF
Once you’ve designed the HTML template, converting it into a PDF with PDFKit is straightforward:
The result is a PDF file styled according to your HTML and CSS. You can customize this further by passing additional options to wkhtmltopdf, like page size or orientation:
Styling PDFs: Managing CSS for Professional-Looking Documents
One of the benefits of using HTML/CSS for PDF generation is that you can leverage all the power of CSS to style your documents. You can create tables, adjust font sizes, apply background colors, and more. Ensure your stylesheets are correctly linked in the HTML:
This allows for clean separation of content and design, making it easier to maintain and update your PDFs.
Dynamic Data with HTML Template Engine
For dynamic content like invoices, you can use a templating engine like Jinja2 to populate your HTML template with data:
Using Jinja2 ensures that you can dynamically generate content for each PDF without manually editing the HTML file.
Improving Performance: Asynchronous PDF Generation in Python
In high-traffic applications, generating PDFs synchronously might create bottlenecks. You can offload this task by using asynchronous Python libraries like Celery to generate PDFs in the background, improving performance and user experience:
This approach ensures scalability by allowing your application to handle PDF generation as a background process.
Debugging Common Issues When Converting HTML to PDF with PDFKit
Sometimes, HTML elements might not render as expected in your PDF. This could be due to unsupported CSS properties in wkhtmltopdf. Use the --debug-javascript flag to help identify issues with JavaScript execution, and ensure that all assets like fonts or images are correctly loaded.
How to Use a PDF API to Automate PDF Creation at Scale
For SaaS applications that need to generate PDFs at scale, integrating with a third-party PDF API can be a more efficient approach. APIs such as pdforge offer extensive features like watermarking, encryption, and built-in scaling capabilities. They simplify the process of handling high volumes of PDF generation requests without the overhead of managing your own infrastructure.
Here’s an example of how you might integrate with a PDF API:
Using a PDF API like this can help offload the heavy lifting involved in creating and managing large-scale PDF generation.
Conclusion
PDFKit is an excellent choice for generating PDFs from HTML when you need flexibility and ease of use in your SaaS application. However, for advanced use cases or large-scale deployments, you may want to consider third-party solutions like pdforge.
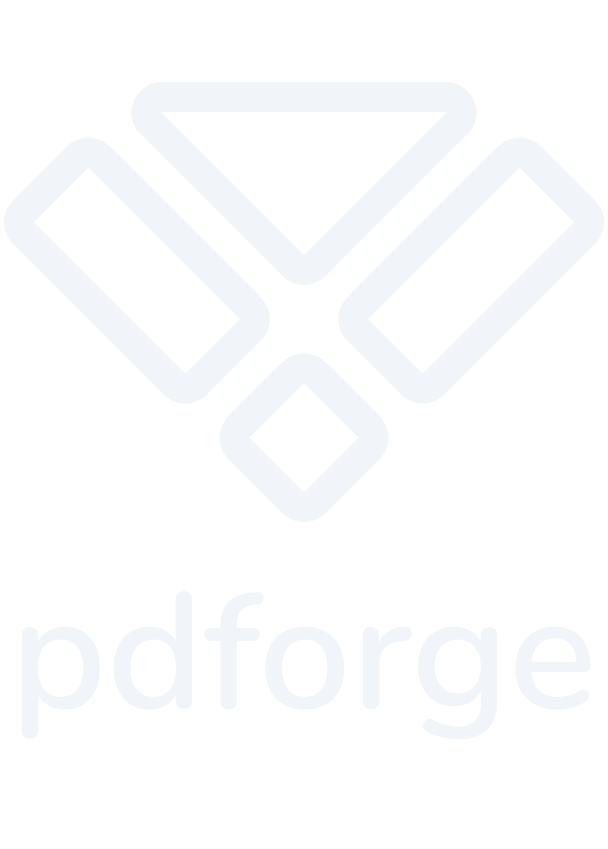
Try for free
7-day free trial