How to Generate PDF from HTML Using ReportLab in Python
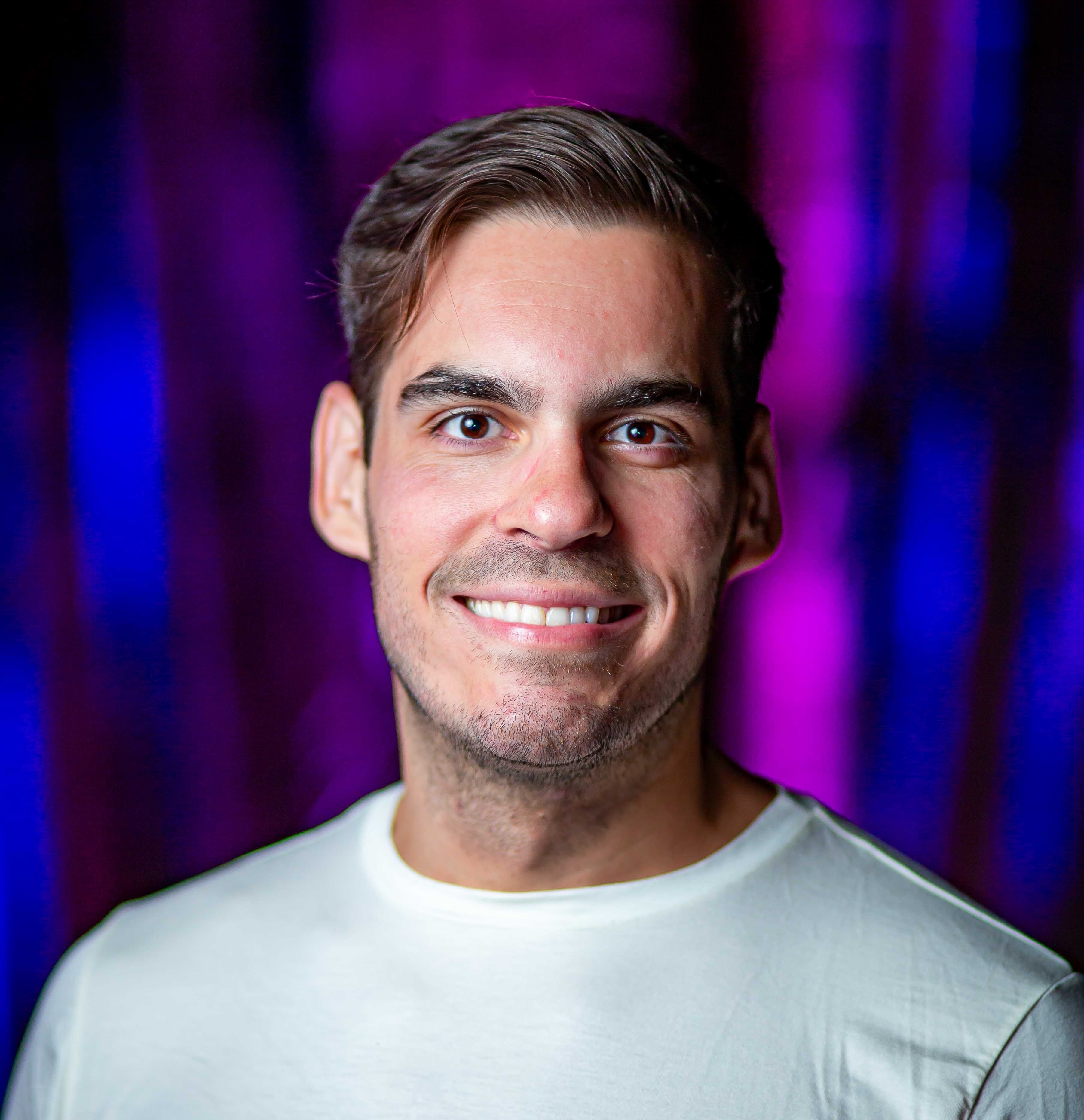
An Introduction to ReportLab: A Python PDF Generation Library
ReportLab is a powerful and flexible Python PDF generation library, well-suited for SaaS applications that require dynamic PDF creation. With ReportLab, you can programmatically create PDFs from scratch, offering deep customization for layouts, fonts, graphics, and tables. Its flexibility allows it to handle complex reporting needs, making it a top choice for developers seeking more than basic HTML-to-PDF conversion.
You can access the full documentation here.
Comparing ReportLab with Pyppeteer and PyPDF2
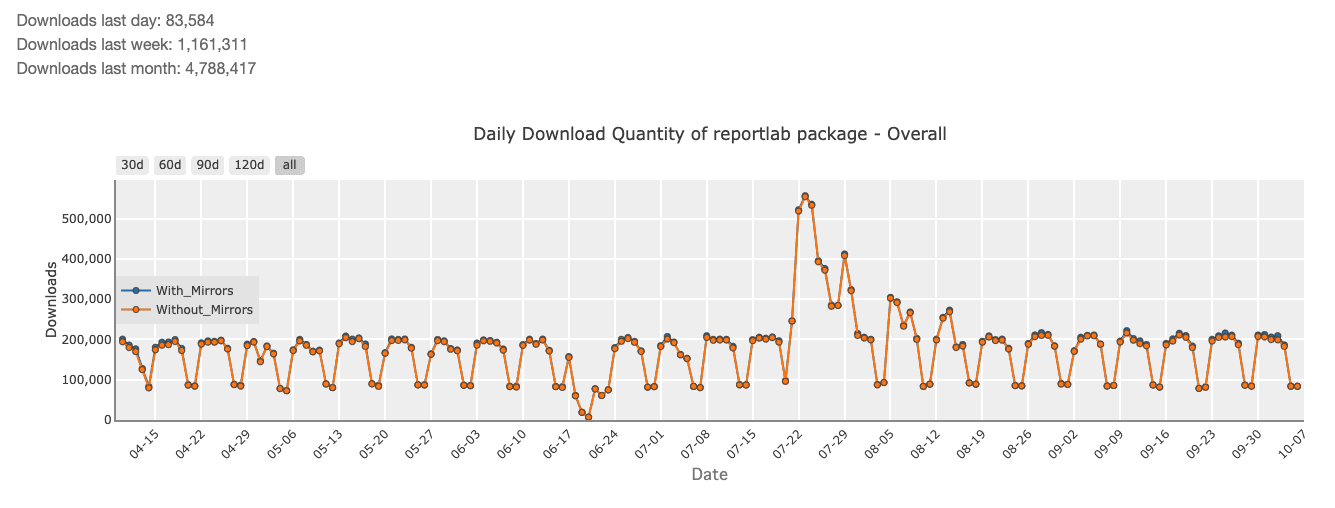
While ReportLab excels in customization and granular control, it is often compared with Pyppeteer (2,063,960 montly downloads) and PyPDF2 (9,982,763 monthly downloads), two other popular libraries.
Pyppeteer, based on Chromium, renders HTML into PDFs using a browser engine, offering pixel-perfect fidelity to web designs, but with less control over layout structure or programmatic content.
On the other hand, PyPDF2 focuses on manipulating existing PDFs—merging, splitting, and encrypting—making it ideal for tasks where PDFs need to be edited rather than created. ReportLab provides a balance, combining the flexibility of creation and customization that Pyppeteer lacks, while offering more control than PyPDF2 in terms of building documents from the ground up.
Setting Up the Environment for HTML to PDF with ReportLab
To start working with ReportLab, we need to install the necessary dependencies and create a project setup.
Installing ReportLab and Required Dependencies
First, install ReportLab via pip:
We’ll also need lxml for parsing HTML content:
A Quick Overview of Python and HTML to PDF Conversion
ReportLab doesn’t directly convert HTML to PDF, but rather, it allows you to create PDFs by manipulating Python objects, with full control over the document structure. We’ll use an HTML invoice example and convert its structure to a PDF format using ReportLab.
Setting Up a Basic Python Project for PDF Generation
Organize your project as follows:
In html_templates/invoice.html, we’ll create a simple invoice template:
We will convert this invoice into a PDF with ReportLab.
Step-by-Step Guide: How to Generate PDF from HTML Using ReportLab
Creating a Simple HTML Structure for Conversion
Load the HTML content into your Python script using lxml:
The HTML structure represents an invoice template with basic CSS for styling. Now, we will use ReportLab to programmatically create a PDF from this structure.
Converting HTML to PDF with ReportLab: The Core Functions
In pdf_generator.py, we can now use ReportLab’s SimpleDocTemplate and Paragraph components to structure the PDF:
This code will capture the basic elements of our invoice and create a PDF.
Customizing PDF Output: Fonts, Styles, and Layout Adjustments
ReportLab allows extensive customization. For example, let’s adjust fonts, margins, and other layout settings:
This gives you control over how the text appears on the PDF, aligning it with your branding.
Handling Dynamic Content: Generating PDFs from Real-Time Data
Dynamic content is crucial for invoices that pull from databases or APIs. Here’s how you could integrate Python variables into the PDF generation process:
This approach allows you to dynamically generate invoices based on user input or database records.
Modifying an Existing HTML File Using ReportLab
One of ReportLab’s powerful features is the ability to modify existing content, such as adding elements to an HTML template or adjusting styles on-the-fly. Suppose you want to update the invoice by adding a company logo or changing the layout dynamically. Here’s how you could achieve that:
This allows for real-time adjustments to HTML-based templates, providing flexibility for modifying documents.
How to Use a PDF API to Automate PDF Creation at Scale
When dealing with large-scale PDF generation, especially in a SaaS environment, automation becomes key. Although ReportLab is a powerful library for generating PDFs, integrating it with a PDF API can streamline the process, particularly when working with high volumes or requiring web-based solutions.
A popular PDF API such as pdforge allows you to offload the rendering and scaling to an external service. Here’s how you can integrate it:
By integrating ReportLab with a PDF API, you can automate bulk PDF generation and scale it across multiple platforms, reducing processing time and system load.
Conclusion
ReportLab offers unparalleled flexibility for creating custom PDF reports in Python, making it ideal for SaaS developers who need dynamic, branded, and highly customized PDFs.
While Pyppeteer is great for pixel-perfect web-to-PDF rendering and PyPDF2 excels at editing existing PDFs, ReportLab stands out with its deep programmatic control over document creation. It’s the best choice for developers needing extensive layout, font, and content customization.
However, for large-scale automation, integrating with a third-party PDF API like pdforge can streamline PDF generation at scale.
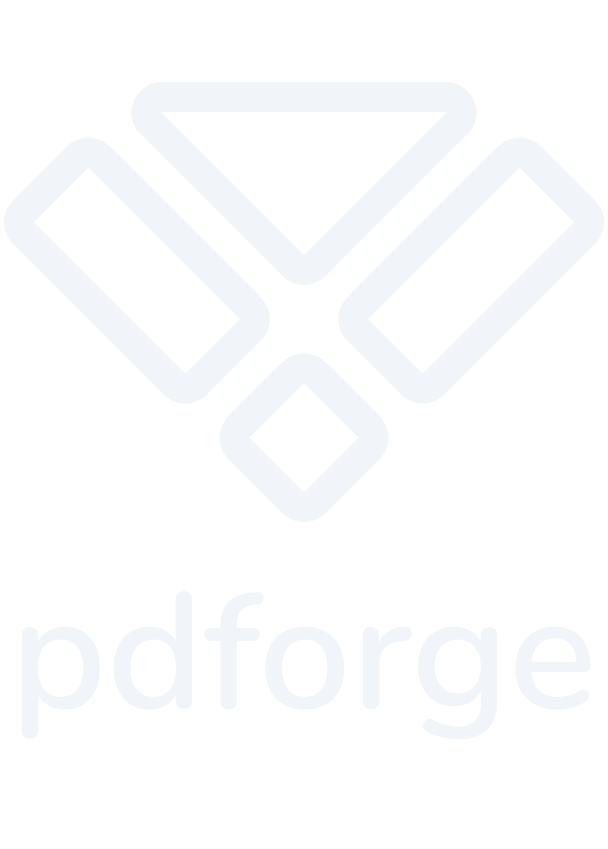
Try for free
7-day free trial