Overview: top pdf python libraries
This article covers top pdf libraries in python to help developers improve pdf generation workflows. It introduces how html to pdf transforms static or dynamic content into portable documents. It also outlines best libraries, from browser-driven conversions to third-party APIs, helping you find a tailored solution for your SaaS needs.
Browser-based PDF libraries convert web pages into pdf documents by leveraging a headless browser’s print API.
Non-browser-based methods rely on native Python systems like wkhtmltopdf or a drawing canvas.
Third-party pdf generation API can lighten operational load while delivering advanced features, including collaborative editing.
Browser-based PDF libraries
Browser-based tools operate by rendering your HTML in a headless environment, then invoking a print-like method to create a final pdf. This ensures high-fidelity layouts consistent with real-world browser behavior.
Playwright and Pyppeteer dominate this category, each depending on browser engines to capture complex CSS or JavaScript. They excel in scenarios like form creation or interactive UI where visual accuracy is paramount.
Non-browser-based PDF libraries
Non-browser-based solutions can operate without launching a headless browser. They either leverage a command-line utility like wkhtmltopdf or rely on native Python drawing APIs. This approach typically consumes fewer system resources.
PDFKit and pypdf2 sit in this realm. PDFKit seamlessly transforms HTML to PDF with minimal setup, while pypdf2 focuses on merging, splitting, or watermarking existing files. Meanwhile, ReportLab uses a canvas model for entirely custom design, allowing you to position text, images, or shapes with absolute precision.
Third party pdf generation API
Third-party APIs such as pdforge integrate easily with existing code bases and scale to large volume pdf generation. They reduce infrastructural strain and provide easy ways to embed dynamic data into HTML or no-code templates, making them ideal for projects requiring robust collaboration or custom branding.
In-depth look at top pdf libraries python
Generation PDF with Playwright
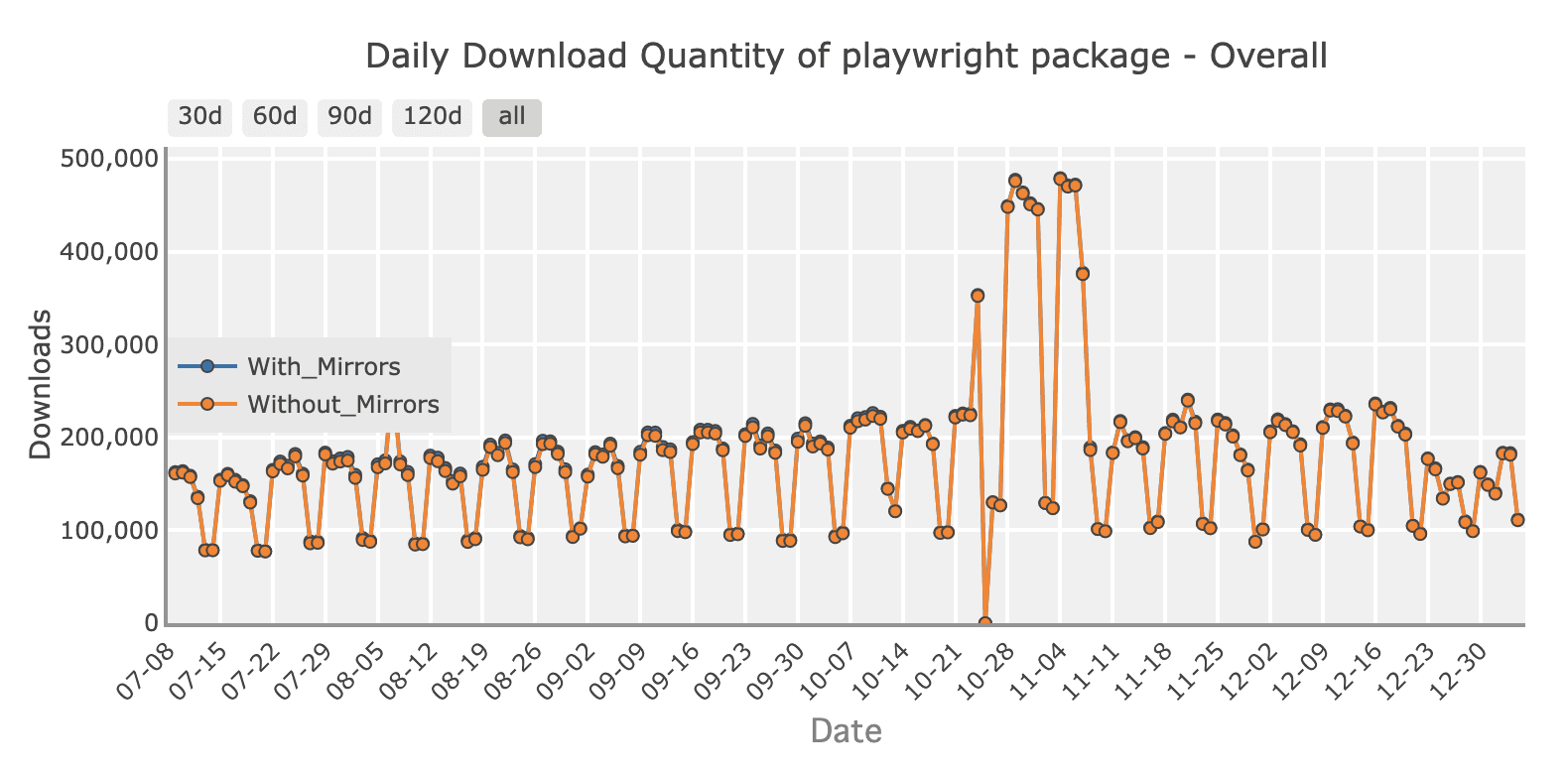
Playwright is a cross-browser automation library from Microsoft. Install with:
It supports Chromium, Firefox, and WebKit. Suppose you have an invoice.html describing client details and itemized charges:
Playwright is ideal for dynamic html to pdf tasks. For deeper details, see our full guide on how to transform html into pdf with playwright in Python.
Generation PDF with Pyppeteer
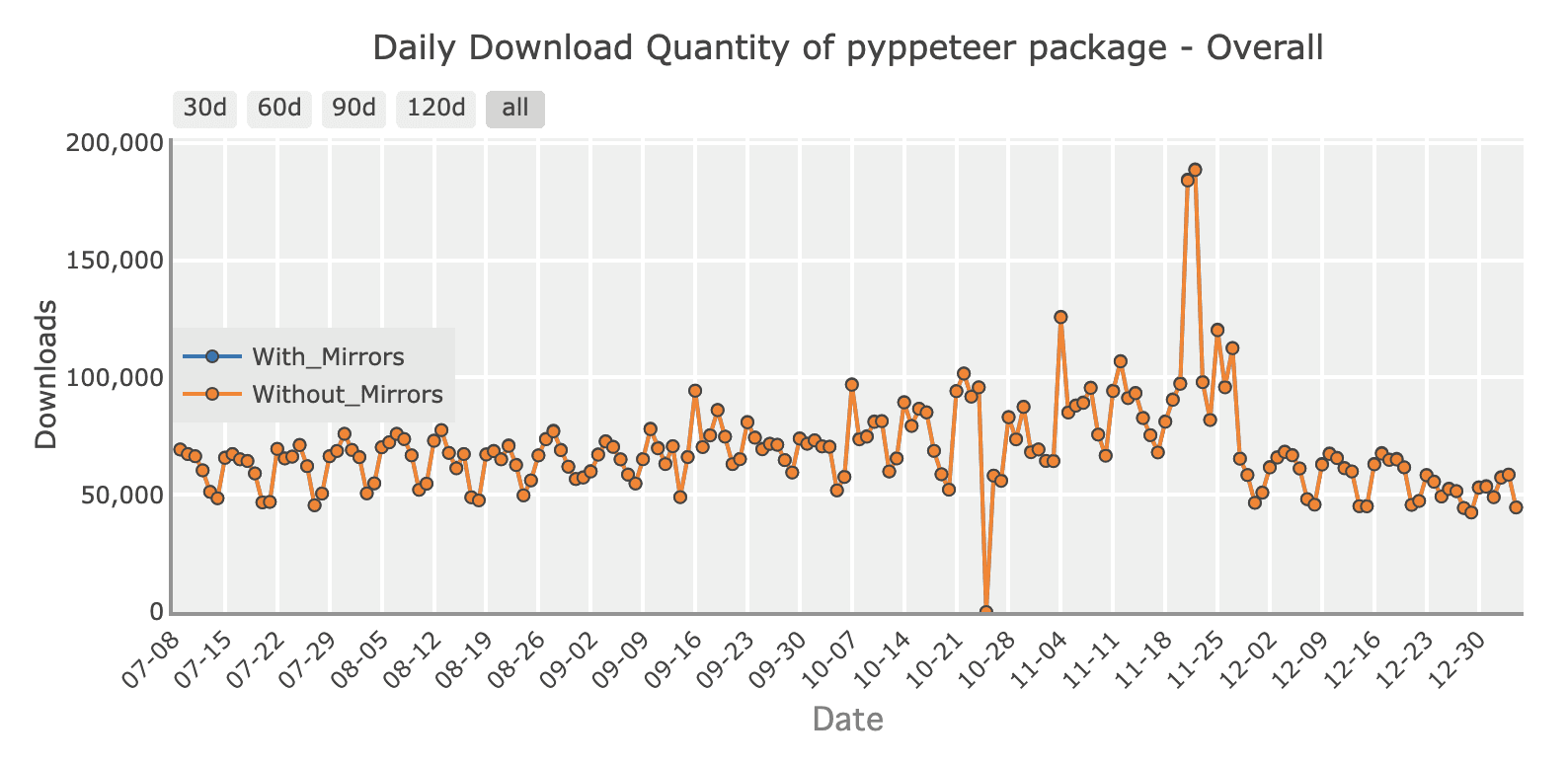
Pyppeteer, an unofficial port of Puppeteer, offers browser-based pdf generation in Python. Install with:
Same invoice.html example:
It accurately reflects any JavaScript or styling on the page. You can check out our guide for HTML into PDF transformation using pyppeteer.
Generation PDF with PDFKit
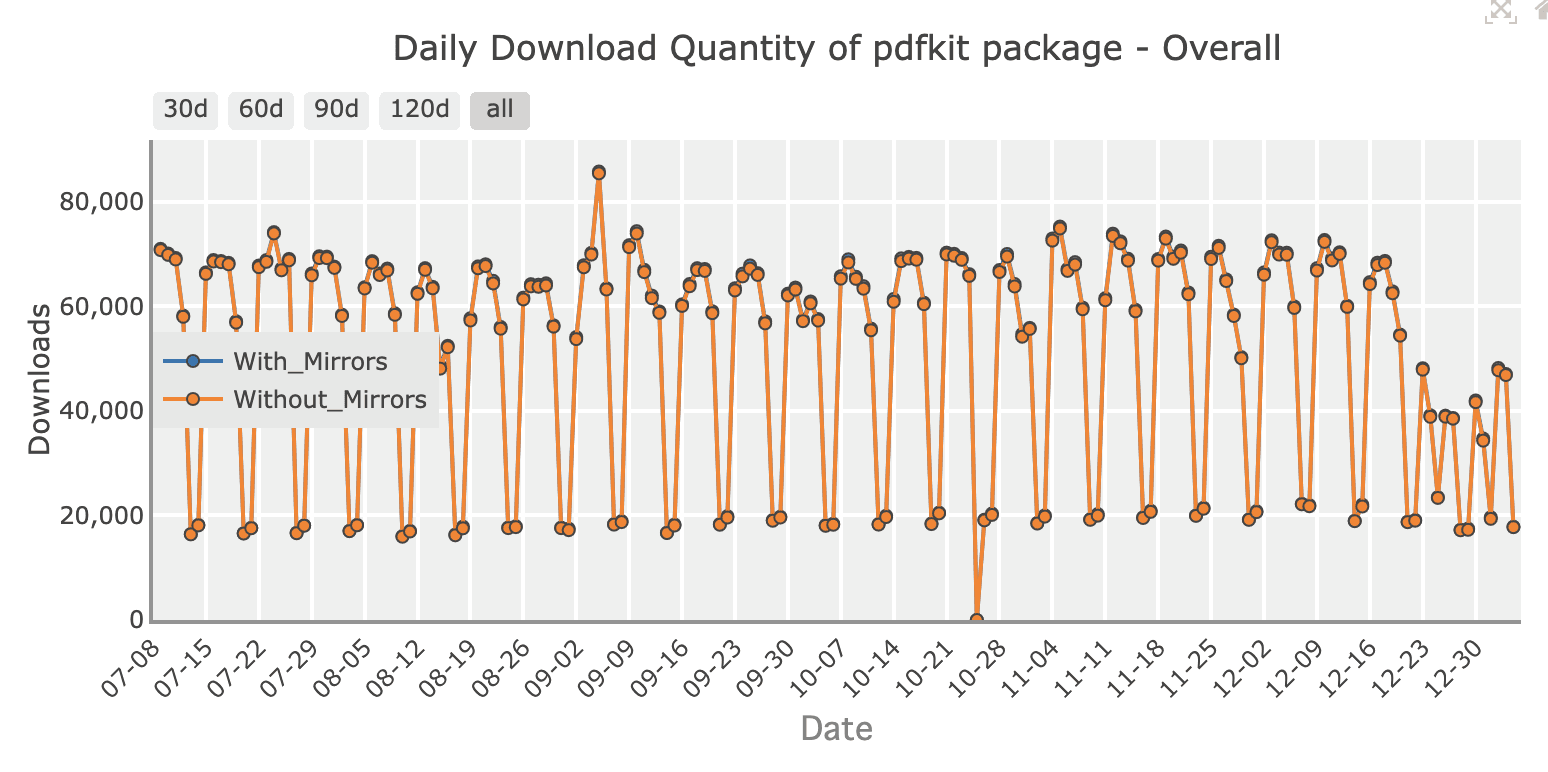
PDFKit converts HTML content into PDFs using wkhtmltopdf. Install:
Using the same invoice.html:
PDFKit delivers simplicity and stable results with minimal overhead. We also have a full guide on generating pdf from html with pdfkit.
Generation PDF with pypdf2
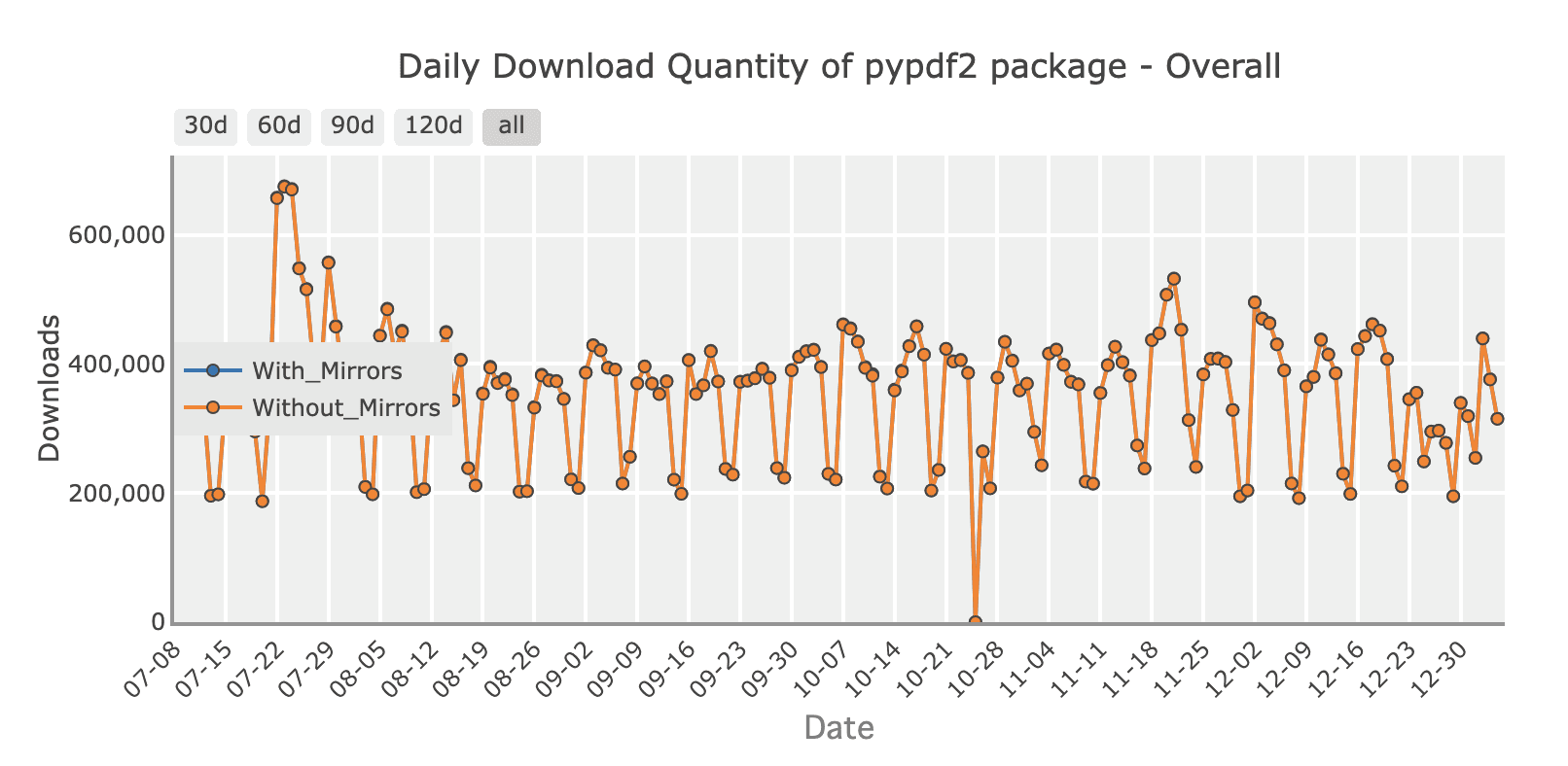
pypdf2 focuses on modifying existing PDF documents. Install with:
pypdf2 primarily enables reading, merging, splitting, or adding pages to existing PDFs. Though it lacks robust HTML conversion capabilities, you can still assemble a new invoice PDF by using a base template and placing text annotations on the page.
Below is an example of creating an invoice from a predefined invoice_template.pdf. The code adds a free text annotation, effectively writing text into the PDF:
In practice, this approach works best when you already have a PDF with placeholders or background graphics. Although pypdf2 can’t transform raw HTML, it complements your pipeline if you often deal with watermarks, bookmarks, or merging multi-page PDFs in a SaaS environment. For more powerful layout and direct HTML-to-PDF needs, consider pairing it with other libraries or template engines. If you want to know more about pypdf2, check out our guide here.
Generation PDF with reportlab
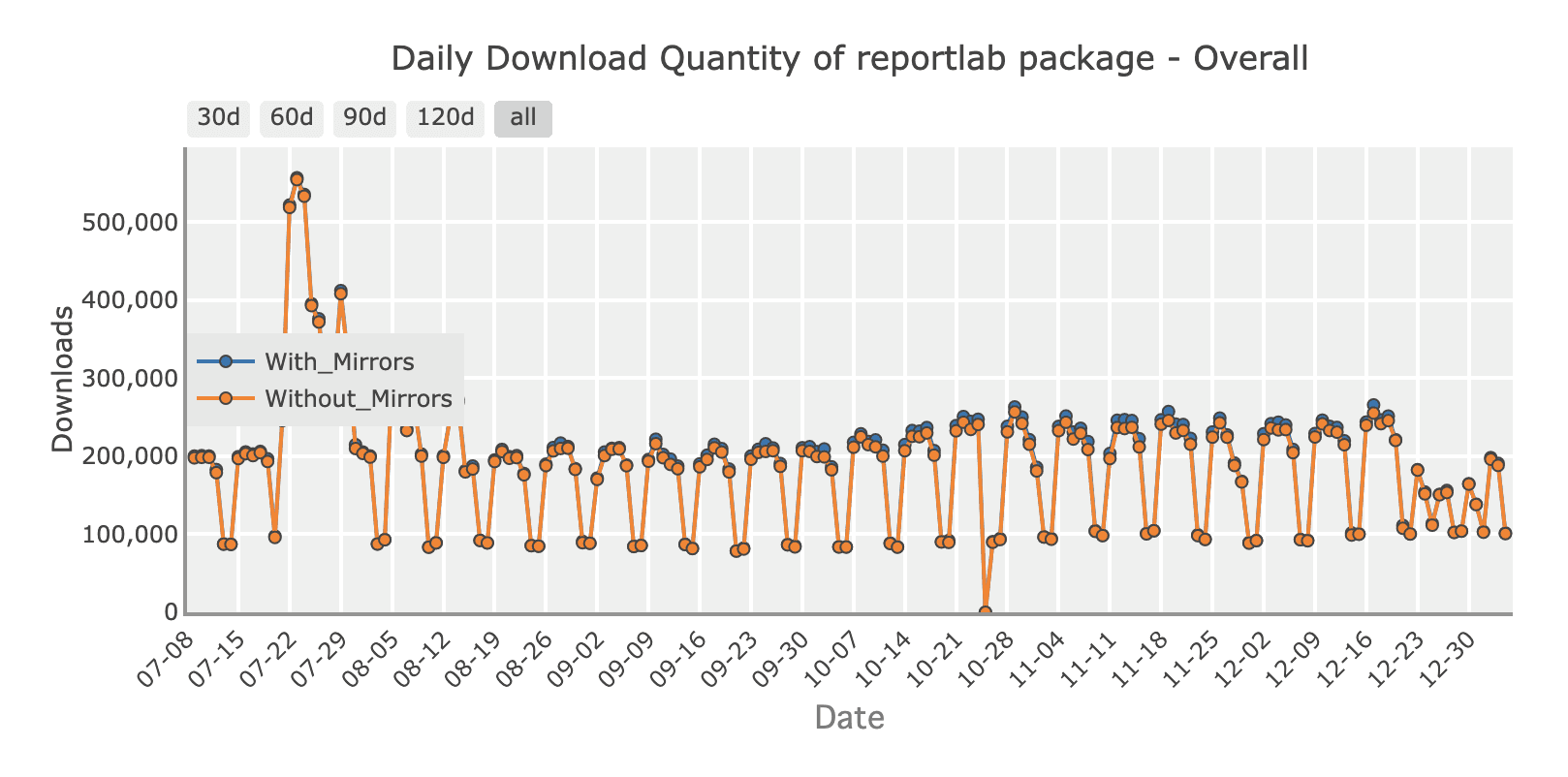
ReportLab builds PDFs from scratch using a drawing canvas. Install:
Generating an invoice:
ReportLab supports text flow, shapes, and custom styles for professional branding. Here's a guide on how to generate pdf documents with reportlab.
Generation PDF with pdforge
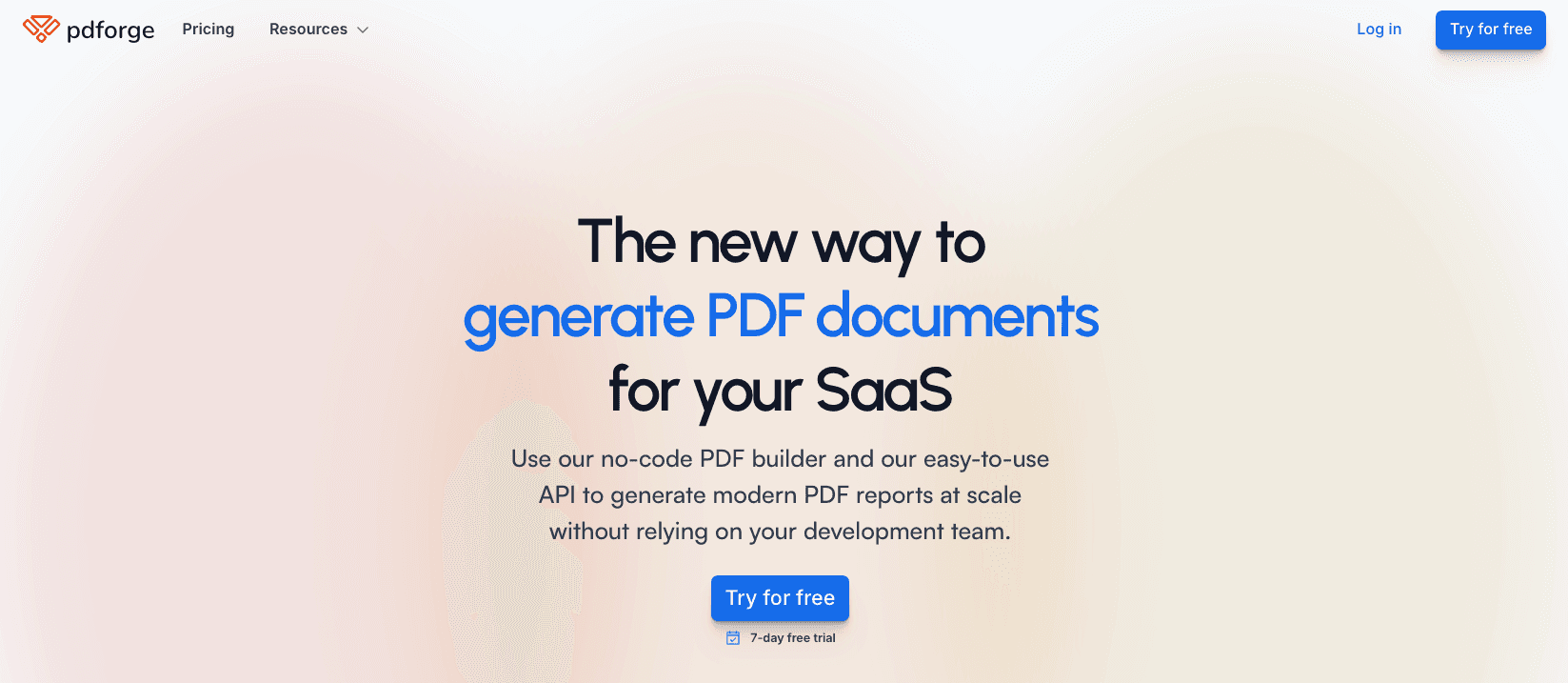
pdforge is a third-party pdf generation API. You can create beautiful reports with flexible layouts and complex components with an easy-to-use opinionated no-code builder. Let the AI do the heavy lifting by generating your templates, creating custom components or even filling all the variables for you.
You can handle high-volume PDF generation from a single backend call.
Here’s an example of how to generate pdf with pdforge via an API call:
You can create your account, experience our no-code builder and create your first layout template without any upfront payment clicking here.
If you want to explore other Third Party APIs, we listed all the top 7 PDF Generation APIs in 2025 for PDF Automation. Take a look and choose the best option for you!
Comparison between libraries
Each library addresses different top pdf libraries python priorities:
Conclusion
The best pdf generation solution depends on your requirements.
If your primary goal is precise browser rendering, choose browser-based tools such as Playwright or Pyppeteer. Those seeking simpler pipelines might favor PDFKit. Browser-based pdf generation provides high fidelity for visually dynamic pages, whereas non-browser-based solutions focus on performance and ease of setup.
Choose third-party pdf generation APIs, like pdforge, if you don't want to waste time maintaining pdfs layouts and their infrastructure or if you don't want to keep track of best practices to generate PDFs at scale.