How to Scale HTML to PDF with AWS Lambda and Playwright
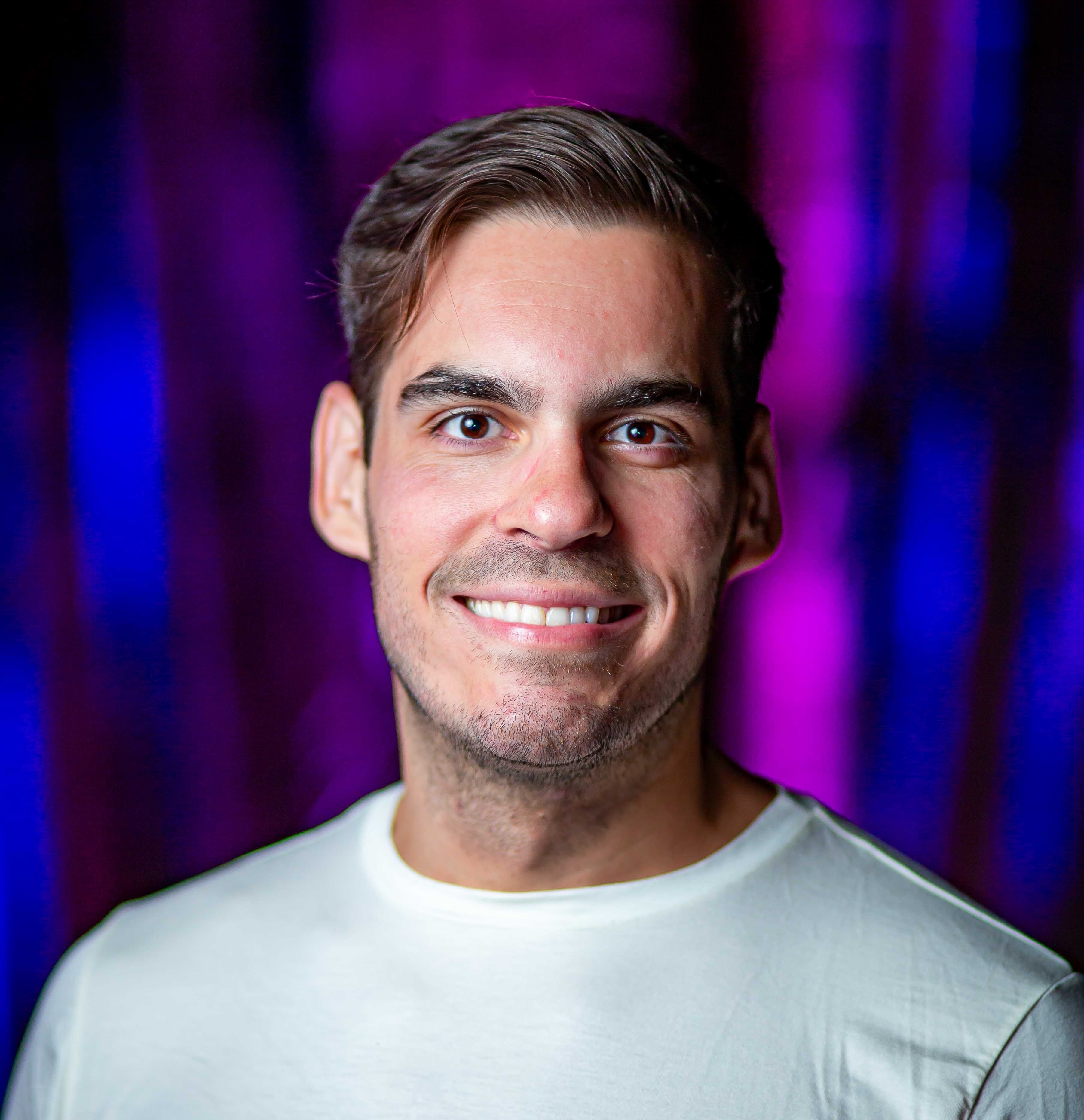
Introduction to Serverless HTML to PDF Conversion
Looking for a step-by-step guide to deploy HTML to PDF on a serverless architecture using AWS Lambda with Playwright? Came to the right place!
While there are numerous guides on using HTML to PDF libraries, practical instructions on scaling this process are scarce. This article will help you implement a scalable solution for generating PDFs in your SaaS application.
The Need for Scalable PDF Generation in SaaS Applications
SaaS applications often require dynamic PDF generation for reports, invoices, and documentation. Traditional server-based methods can be resource-intensive and challenging to scale. Leveraging a serverless architecture allows you to generate PDFs efficiently while automatically handling scaling during peak usage.
Playwright Overview
Playwright is an open-source Node.js library that automates browser interactions. It supports Chromium, Firefox, and WebKit, making it ideal for rendering web pages and generating PDFs. Its ability to run headless browser instances makes it suitable for serverless environments like AWS Lambda.
We have several guides on how to use playwright for pdf generation, so this article will focus mainly on the serverless architecture, but you can check out the full guides here:
Setting Up Playwright and AWS Lambda for Serverless PDF Generation
Integrating Playwright with AWS Lambda enables scalable and cost-effective PDF generation without managing server infrastructure.
Implementing the HTML to PDF Serverless Function
First, set up a Node.js project and install Playwright:
Create a script that navigates to a URL and generates a PDF:
Configuring AWS Lambda
AWS Lambda doesn’t include the necessary Chromium binaries by default. You’ll need to include them in a Lambda Layer.
Uploading Chromium to an AWS Lambda Layer
Download a compatible version of Chromium for AWS Lambda. You can find precompiled binaries in repositories like alixaxel/chrome-aws-lambda or build your own.
1. Download Chromium Binary:
Download the headless Chromium binary compatible with AWS Lambda’s Amazon Linux environment.
2. Create a ZIP Archive:
Package the chromium binary and necessary libraries into a ZIP file.
3. Create a Lambda Layer:
In the AWS Lambda console, navigate to “Layers” and create a new layer. Upload the ZIP archive you created.
4. Add the Layer to Your Function:
In your Lambda function’s configuration, add the newly created layer.
Configuring Lambda with Docker (Recommended)
Alternatively, you can package your Lambda function and Chromium dependencies using Docker.
Dockerfile Example:
If you want to learn more about build Docker image from custom Dockerfile and how to enhance it, we recommend this guide.
Full Lambda Function
Here’s the complete Lambda function code:
Uploading the Docker Image to AWS
To deploy the Docker image to AWS:
1. Build the Docker Image for AWS Lambda:
Note: If you’re using an M1 MacBook, use docker buildx to emulate the linux/amd64 platform.
2. Tagging Your Docker Image:
After building the image, tag it with your Amazon ECR repository URI to prepare it for pushing.
• Create an ECR Repository (if you haven’t already):
• Authenticate Docker to Your ECR Registry:
• Tag Your Docker Image:
Replace your-image-name, your-account-id, your-region, and your-repo-name with your specific AWS account details and desired repository name.
3. Push the Image to ECR:
4. Deploy the Lambda Function:
In AWS Lambda, create a new function using the container image you’ve pushed to ECR.
Advanced Topics
Handling Concurrency and Scaling with AWS Lambda
AWS Lambda has a default concurrency limit of 1,000 simultaneous executions per region. To increase this limit:
• Request a Quota Increase:
Go to the AWS Service Quotas console and request an increase for “Concurrent executions” for Lambda.
Common Issues with Playwright and AWS Lambda
Running Playwright in a serverless environment can present challenges.
Chrome Being Memory-Intensive
Chromium can consume significant memory. To mitigate issues:
• Clean the /tmp
Directory:
AWS Lambda provides a limited /tmp directory (512 MB). Cleaning up temporary files helps manage space.
M1 vs. Intel Processors
If you’re developing on an M1 MacBook, you may encounter compatibility issues due to architecture differences.
• Use Docker Buildx for Cross-Platform Builds:
This command emulates the linux/amd64 platform, ensuring compatibility with AWS Lambda’s execution environment.
Conclusion
Implementing HTML to PDF conversion in a serverless architecture with Playwright and AWS Lambda offers scalability and cost savings.
While setting up this infrastructure requires effort, it eliminates the need to manage servers.
Alternatively, third-party solutions like pdforge can handle PDF generation without the overhead of maintaining your own architecture.
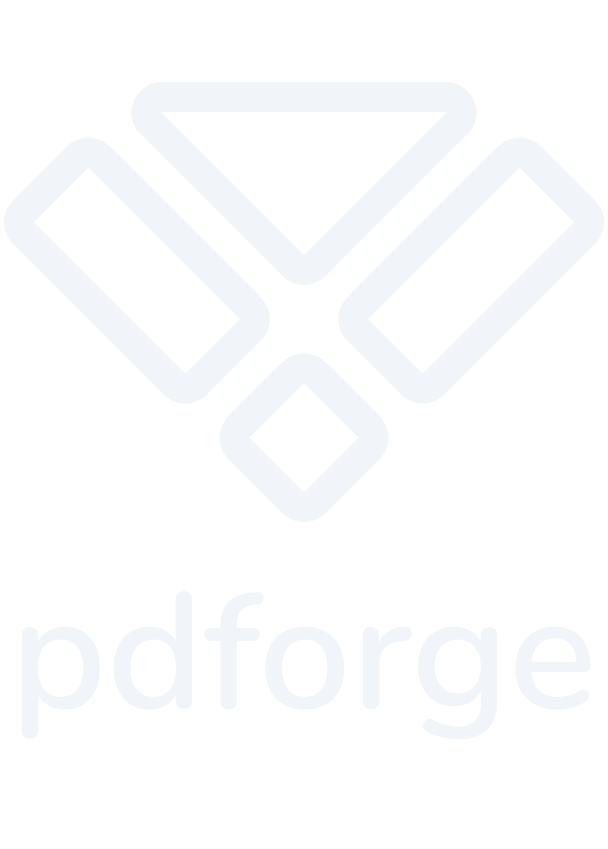
Try for free
7-day free trial